Question
this code wont complie pls fix it // File Name Point2D.h #ifndef Point2D_H #define Point2D_H #include using namespace std; // Class Point2D definition class Point2D
this code wont complie pls fix it
// File Name Point2D.h #ifndef Point2D_H #define Point2D_H #include
// Class Point2D definition class Point2D { private: // Data member double xval, yval; public: // Prototype of member function Point2D(double, double); Point2D(); double x(); double y(); double dist(Point2D); Point2D add(Point2D); Point2D sub(Point2D); void move(double, double); bool equals(Point2D); void print(); };// End of class #endif // End of header file
--------------------------------------------------------------------------------------------
// File Name Point2D.cpp #include "Point2D.h" #include
// Define the Default constructor Point2D::Point2D() { xval = 0.0; yval = 0.0; }// End of function
// Define the two argument constructor with arguments x and y Point2D::Point2D(double x, double y) { xval = x; yval = y; }// End of function
// Define the method x which returns the xval double Point2D::x() { return xval; }// End of function
// This method returns the yval of the point double Point2D::y() { return yval; }// End of function
// Define the method dist which takes a Point2D as input and returns a double double Point2D::dist(Point2D other) { // Calculate xd by subtracting this point's xval with the input point's xval double xd = other.xval - this->xval; // Calculate yd by subtracting this point's yval with the input point's yval double yd = other.yval - this->yval; // return the square root of (xd*xd + yd*yd) return sqrt((xd * xd) + (yd * yd)); }// End of function
// Adds the two points and returns the result Point2D Point2D::add(Point2D b) { return Point2D(xval + b.x(), yval + b.y()); }// End of function
// Subtracts the two points and returns the result. Point2D Point2D::sub(Point2D b) { return Point2D(xval - b.x(), yval - b.y()); }// End of function
// Define the function move which takes a and b as inputs and adds them to xval // and yval respectively void Point2D::move(double a, double b) { xval += a; yval += b; }// End of function
// Define the print function that prints: (xval,yval) followed by newline void Point2D::print() { cout<<" X - Position: "< // Compares if the two points are equal // Points are equal if their respective xval and yval are equal bool Point2D::equals(Point2D other) { // Compare if the xval of this point equals the xval of the input and // if yval of this point equals yval of input point if(other.xval == xval && other.yval == yval) return true; else return false; }// End of function ---------------------------------------------------------------------------------------------------- // File Name Point2DMain.cpp #include // main function definition int main() { //Lets create a point(0,0) (use default constructor) and name it pointA; Point2D pointA; //Lets create a point(2,2) and name it pointB; Point2D pointB(2,2); //Lets print the details of the points cout<<" The points pointA and pointB are: "; // Print pointA pointA.print(); // Print pointB pointB.print(); // Find the distance between pointA and pointB and print it. cout<<" Distance between pointA and pointB is "< // Lets shift the points pointA and pointB by (3,3) // Use the move function to shift the point cout<<" Moving the points by (3, 3) "; // Move pointA by (3,3) pointA.move(3, 3); // Move pointB by (3,3) pointB.move(3, 3); // Lets the print the new details of the changed points cout<<"The new points pointA and pointB are: "; pointA.print(); pointB.print(); // Lets see if the distance between pointA and pointB has changed // Find the new distance between pointA and pointB and print it. // Print out "The new distance between the pointA and pointB is ....[Fill the rest] cout<<" New distance between pointA and pointB is "< // Show that vector addition works with our Point2D objects. // We will show that if // a1 = (x1, y1) and a2 = (x2, y2), then // if a3 = a1 + a2 it implies a3 will be (x1+x2, y1+y2) // Example a1 = (1,1) and a2 = (2,3) then // a3 = a1 + a2 implies a3 = (3,4); // Create point a1 = (1,1) Point2D a1(1,1); // Create point a2 = (2,3) Point2D a2(2,3); // Create point a3 = (3,4) Point2D a3(3,4); cout<<" The points a1 and a2 are: "; a1.print(); a2.print(); cout<<" The sum a3 = a1 + a2 is "; a3.print(); // Call the add function using a1 and a2 and assign the result to new_a3 Point2D new_a3; cout<<" The point new_a3 = a1.add(a2) is "; new_a3 = a1.add(a2); new_a3.print(); // Check if a3 and new_a3 are equal using the equals function. // If they are equal print "My vector addition works!!" // else print "My vector addition fails!!" if(a3.equals(new_a3)) cout<<" My vector addition works!!"; else cout<<" My vector addition fails!!"; // Lets now do the same for subtraction. // We will show that if // s1 = (x1, y1) and s2 = (x2, y2), then // if s3 = s1 - s2 it implies s3 will be (x1-x2, y1-y2) // Example s1 = (1,1) and s2 = (2,3) then // s3 = s1 - s2 implies s3 = (-1,-2); // Lets use user input to create two points and subtract them double x1, y1, x2, y2; cout<<"Enter details of s1 "; cout<<"x = "; cin>>x1; cout<<"y = "; cin>>y1; // Create point s1 using x1 and y1 Point2D s1(x1, y1); cout<<"Enter details of s2 "; cout<<"x = "; cin>>x2; cout<<"y = "; cin>>y2; // Create point s2 using x2 and y2 Point2D s2(x2, y2); // Create point s3 as (x1-x2, y1-y2) Point2D s3(x1 - x2, y1 - y2); // Call the sub function using s1 and s2 and assign the result to new_s3 Point2D new_s3; new_s3 = s1.sub(s2); cout<<"The points s1 and s2 are: "; s1.print(); s2.print(); cout<<" The difference s3 = s1 - s2 is "; s3.print(); // Call the sub function using s1 and s2 and assign the result to new_s3 cout<<" The point new_s3 = s1.sub(s2) is "; new_s3.print(); // Check if s3 and new_s3 are equal using the equals function. If they are equal print "My vector subtraction works!!" // else print "My vector subtraction fails!!" if(s3.equals(new_s3)) cout<<" My vector subtraction works!!"; else cout<<" My vector subtraction fails!!"; return 0; }// End of main Sample Output: The points pointA and pointB are: X - Position: 0 Y - Position: 0 X - Position: 2 Y - Position: 2 Distance between pointA and pointB is 2.82843 Moving the points by (3, 3) The new points pointA and pointB are: X - Position: 3 Y - Position: 3 X - Position: 5 Y - Position: 5 New distance between pointA and pointB is 2.82843 The points a1 and a2 are: X - Position: 1 Y - Position: 1 X - Position: 2 Y - Position: 3 The sum a3 = a1 + a2 is X - Position: 3 Y - Position: 4 The point new_a3 = a1.add(a2) is X - Position: 3 Y - Position: 4 My vector addition works!!Enter details of s1 x = 12 y = 3 Enter details of s2 x = 6 y = 2 The points s1 and s2 are: X - Position: 12 Y - Position: 3 X - Position: 6 Y - Position: 2 The difference s3 = s1 - s2 is X - Position: 6 Y - Position: 1 The point new_s3 = s1.sub(s2) is X - Position: 6 Y - Position: 1 My vector subtraction works!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
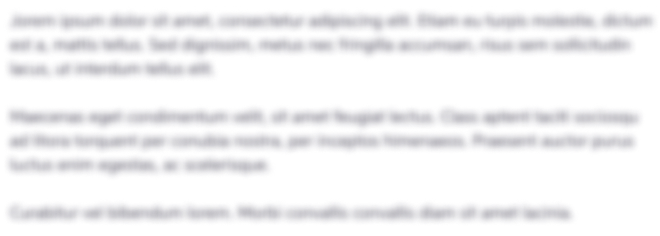
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started