Question
This exercise asks you to to develop a GradeSummary class that takes multiple instances of StudentResult and produce a summary of how many students achieved
This exercise asks you to to develop a GradeSummary
class that takes multiple instances of StudentResult
and produce a summary of how many students achieved each grade (HD, D, C, etc) and who the top scoring student was.
For this exercise your GradeSummary
class will be tested directly. The Runner
class is not involved in testing at all, and any changes you make to it will be ignored. Feel free to edit it to isolate and test different parts of your code.
Specification
Your GradeSummary
class must provide the following methods:
A constructor that accepts no input. Calling this constructor should result in a new instance of GradeSummary
being created that is empty (i.e. does not include any student results).
An add
method that accepts an instance of StudentResult
as input, and returns nothing. Calling this method should cause the summary to be updated to include the given student result.
An addAll
method that accepts an array of StudentResult
as input, and returns nothing. Calling this method should cause the summary to be updated to include all of the given student results.
A reset
method that accepts no input, and returns nothing. Calling this method should cause all data in the summary to be completely reset (i.e. so that it does not include any student results).
A renderToString
method that accepts no input, and returns a String
containing a rendered summary of student results. If the summary is empty (i.e. if it has just been created or just been reset), then this method should return "No results to show" without any punctuation. If at least one student result has been added then it should return a summary of how many students achieved each grade (HD, D, C, etc) and who the top scoring student was (see example section below).
All of these methods should be public
and not static
. There should not be any other public methods or properties, but you are welcome to add any private properties and methods that you feel are necessary/helpful.
Available classes and methods
To assist you in this task, your workspace includes precompiled versions of Student
, Subject
and StudentSummary
, all of which have been implemented according to the specification defined in the previous task (Storing student results). You can use them here even if you didn't successfully complete the previous task.
Your workspace also incudes a precompiled PercentFormatter
class, which provides the following methods:
A static format
method that accepts a decimal number (as a double
) as input, and returns a String
containing the number formatted as a percentage value. For example, if given the number 25 as input, this method will return the string "25.0%".
A static format
method that accepts a dividend (as an int
) and a divisor (as an int
), and returns a String
containing the result of dividing the dividend by the divisor, formatted as a percentage value .For example, if given the dividend 1 and the divisor 3, this method will return the string "33.3%".
Finally, we have also provided the following helper properties/methods within the GradeSummary
class:
A static grades
property that contains a list of available grades (as a String
array) in descending order: e.g. HD, D, C, etc.
A static getGradeIndex
method that accepts a grade (as a String
) and returns the index of that grade in the grades array (as an int
). For example if given the grade "HD", this method will return 0. If given the grade "D" this method will return 1.
Example
Here is what the output of your program should look like if you implement GradeSummary
correctly and leave the runner unchanged:
Note that these results are completely fictional, and have been generated randomly.
Results for Programming Fundamentals HD: 12 (19.4%) D: 6 (9.7%) C: 4 (6.5%) P: 12 (19.4%) Z: 28 (45.2%) Top student: 2532375: Maximilian Owens with 96.7% (HD) Results for Communication for IT Professionals HD: 12 (19.7%) D: 3 (4.9%) C: 4 (6.6%) P: 7 (11.5%) Z: 35 (57.4%) Top student: 2570704: Rebecca Holmes with 100.0% (HD) Results for Introduction to Information Systems HD: 10 (13.0%) D: 9 (11.7%) C: 3 (3.9%) P: 14 (18.2%) Z: 41 (53.2%) Top student: 2420256: Michael Riley with 97.4% (HD)
Your code will be tested with different data (i.e. different student results) so you can't pass the tests just by printing out this summary verbatim.
Runner.java:
public class Runner {
public static void main(String[] args) throws Exception {
StudentResult[] allResults = ResultLoader.readFile("results.csv");
//Create summaries for each subject String[] subjects = { "Programming Fundamentals", "Communication for IT Professionals", "Introduction to Information Systems" };
GradeSummary[] subjectSummaries = new GradeSummary[subjects.length]; for (int i=0 ; i //Go through each result, adding it to the appropriate summary for (StudentResult result: allResults) { //add result to appropriate subject summary for (int i=0 ; i //if the current result is for the current subject if (result.getSubject().getName().equals(subjects[i])) { //add it to the corresponding summary subjectSummaries[i].add(result); } } } //print summaries for (int i=0 ; i< subjects.length ; i++) { System.out.println("Results for " + subjects[i]); System.out.println(subjectSummaries[i].renderToString()); } } } GradeSumamry.java: public class GradeSummary { private static String[] grades = {"HD", "D", "C", "P", "Z"} ; private static int getGradeIndex(String grade) { switch (grade) { case "HD": return 0; case "D": return 1 ; case "C": return 2 ; case "P": return 3 ; case "Z": return 4 ; } return -1 ; } //Implement the rest of GradeSummary here }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
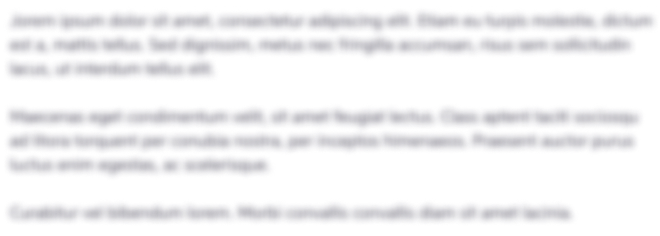
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started