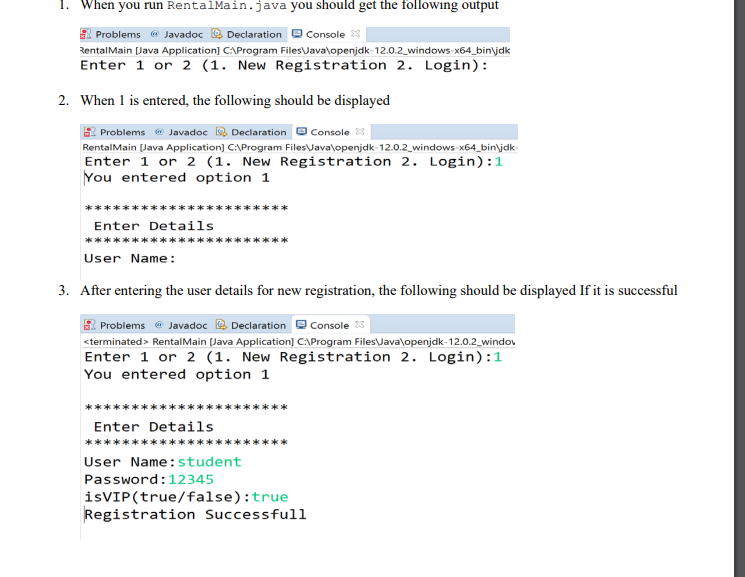
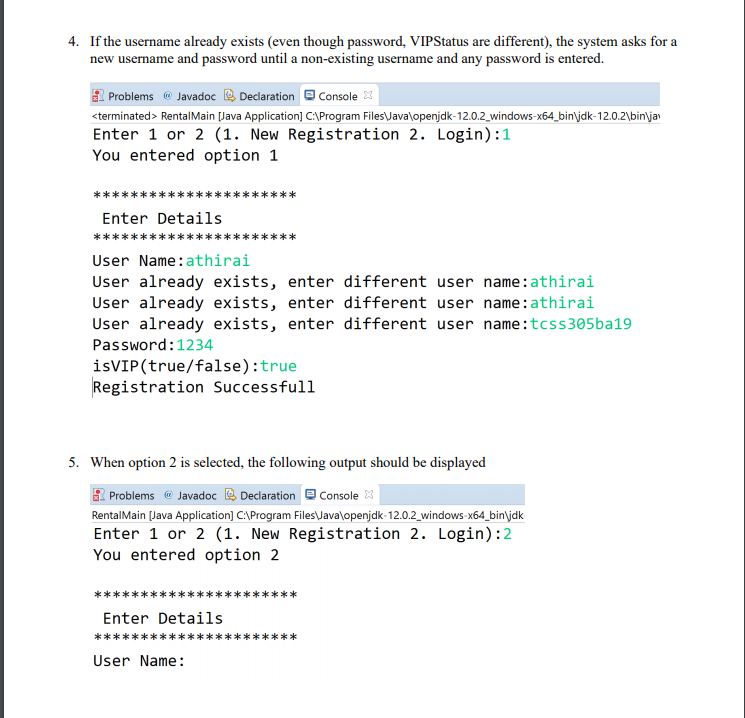
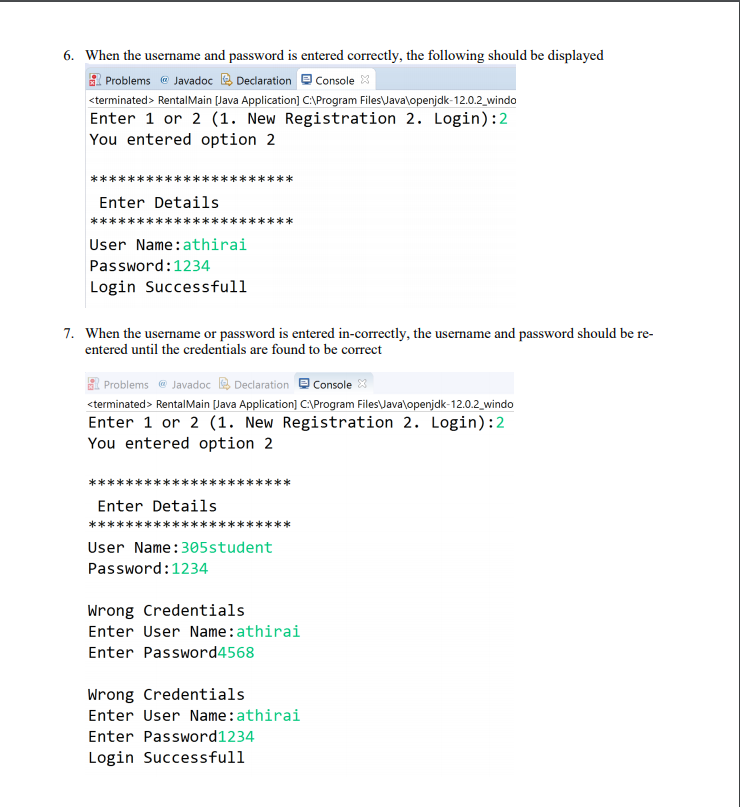
/* * This file is the registration class for the Vehicle Rental System. * */
package model;
import java.util.Map; import utility.FileLoader;
/** * Represents User Sign-in Object. * * Methods of this class throw NullPointerException if required parameters are null. * */
public class Registration {
/** * User Storage File. */ public static final String USERFILE_NAME = "./resources/registeredusers.txt";
/** * The registered user list for signin. */ private final Map myUserList;
/** * Constructs a sigin/registration system. * * */ public Registration() { myUserList = FileLoader.readItemsFromFile(USERFILE_NAME); }
/** * getter for myUserList. * * @return myUserList */ public Map getMyUserList() { return myUserList; }
/** * display sign-in or registration options. */ public void printSignin() {
// ------------Fill in--------------------// }
/** * Verify Sign-in procedure. * * @param theUsername username for sign-in * @param thePassword password for signin * @return sign-in success */ public boolean login(final String theUsername, final String thePassword) {
// ------------Fill in--------------------// return true; }
/** * Adds a user to the registered user list. * * @param theUser an order to add to this shopping cart * @return true/false returns if registration is successfull */ public boolean register(final User theUser) {
// ------------Fill in--------------------//
return true;
}
/** * Empties the user list. */ public void clear() {
// ------------Fill in--------------------// }
@Override /** * String representation of the object * */ public String toString() {
// ------------Fill in--------------------// return "rentz"; }
}
****************************************************
package model;
/** * RentalMain provides the main method for a simple VehicleRental application. *
*/ public final class RentalMain {
/** * A private constructor, to prevent external instantiation. */ private RentalMain() {
}
/** * Main method for Rentz. * * @param theArgs argument for main method. */ public static void main(final String[] theArgs) { final Registration reg = new Registration(); reg.printSignin(); }
}
**************************************************** package model;
/** * Represents a single user for registration or sign-in. User is an immutable object. * * Constructors and methods of this class throw NullPointerException if required parameters are * null. * */ public final class User {
}
1. When you run RentalMain.java you should get the following output Problems Javadoc Declaration Console 3 RentalMain [Java Application] C:Program FilesVava\openjdk-12.0.2_windows-x64_bin jdk Enter 1 or 2 (1. New Registration 2. Login): 2. When 1 is entered the following should be displayed 82 Problems w Javadoc Declaration Console RentalMain [Java Application) C:\Program Files\ava\openjdk-12.0.2_windows-x64_binyjdk- Enter 1 or 2 (1. New Registration 2. Login): 1 You entered option 1 *** Enter Details ****** User Name: 3. After entering the user details for new registration, the following should be displayed If it is successful 3. Problems @ Javadoc Declaration Console x3
RentalMain (Java Application] C:\Program Files\Java\openjdk-12.0.2_windo Enter 1 or 2 (1. New Registration 2. Login): 1 You entered option 1 Enter Details User Name: student Password: 12345 isVIP(true/false): true Registration successfull 4. If the username already exists (even though password, VIPStatus are different), the system asks for a new username and password until a non-existing username and any password is entered. 22. Problems @ Javadoc Declaration Console 2 RentalMain [Java Application] C:\Program Files\Java\openjdk-12.0.2_windows-x64_bin\jdk-12.0.2\binja Enter 1 or 2 (1. New Registration 2. Login):1 You entered option 1 Enter Details ****** ** User Name: athirai User already exists, enter different user name: athirai User already exists, enter different user name:athirai User already exists, enter different user name:tcss305ba19 Password: 1234 isVIP(true/false):true Registration Successfull 5. When option 2 is selected, the following output should be displayed 2. Problems @ Javadoc Declaration Console X RentalMain (Java Application) C:\Program Files\Java\openjdk-12.0.2_windows-x64_bin jdk Enter 1 or 2 (1. New Registration 2. Login):2 You entered option 2 Enter Details User Name: 6. When the username and password is entered correctly, the following should be displayed 2. Problems Javadoc Declaration Console RentalMain [Java Application) C:\Program Files\Java\openjdk-12.0.2_windo Enter 1 or 2 (1. New Registration 2. Login):2 You entered option 2 Enter Details User Name: athirai Password: 1234 Login Successfull 7. When the username or password is entered in-correctly, the username and password should be re- entered until the credentials are found to be correct al Problems Javadoc Declaration Console X RentalMain [Java Application] C:\Program Files\Java\openjdk-12.0.2_windo Enter 1 or 2 (1. New Registration 2. Login):2 You entered option 2 Enter Details User Name: 305 student Password: 1234 Wrong Credentials Enter User Name: athirai Enter Password4568 Wrong Credentials Enter User Name: athirai Enter Password1234 Login Successfull 1. When you run RentalMain.java you should get the following output Problems Javadoc Declaration Console 3 RentalMain [Java Application] C:Program FilesVava\openjdk-12.0.2_windows-x64_bin jdk Enter 1 or 2 (1. New Registration 2. Login): 2. When 1 is entered the following should be displayed 82 Problems w Javadoc Declaration Console RentalMain [Java Application) C:\Program Files\ava\openjdk-12.0.2_windows-x64_binyjdk- Enter 1 or 2 (1. New Registration 2. Login): 1 You entered option 1 *** Enter Details ****** User Name: 3. After entering the user details for new registration, the following should be displayed If it is successful 3. Problems @ Javadoc Declaration Console x3 RentalMain (Java Application] C:\Program Files\Java\openjdk-12.0.2_windo Enter 1 or 2 (1. New Registration 2. Login): 1 You entered option 1 Enter Details User Name: student Password: 12345 isVIP(true/false): true Registration successfull 4. If the username already exists (even though password, VIPStatus are different), the system asks for a new username and password until a non-existing username and any password is entered. 22. Problems @ Javadoc Declaration Console 2 RentalMain [Java Application] C:\Program Files\Java\openjdk-12.0.2_windows-x64_bin\jdk-12.0.2\binja Enter 1 or 2 (1. New Registration 2. Login):1 You entered option 1 Enter Details ****** ** User Name: athirai User already exists, enter different user name: athirai User already exists, enter different user name:athirai User already exists, enter different user name:tcss305ba19 Password: 1234 isVIP(true/false):true Registration Successfull 5. When option 2 is selected, the following output should be displayed 2. Problems @ Javadoc Declaration Console X RentalMain (Java Application) C:\Program Files\Java\openjdk-12.0.2_windows-x64_bin jdk Enter 1 or 2 (1. New Registration 2. Login):2 You entered option 2 Enter Details User Name: 6. When the username and password is entered correctly, the following should be displayed 2. Problems Javadoc Declaration Console RentalMain [Java Application) C:\Program Files\Java\openjdk-12.0.2_windo Enter 1 or 2 (1. New Registration 2. Login):2 You entered option 2 Enter Details User Name: athirai Password: 1234 Login Successfull 7. When the username or password is entered in-correctly, the username and password should be re- entered until the credentials are found to be correct al Problems Javadoc Declaration Console X RentalMain [Java Application] C:\Program Files\Java\openjdk-12.0.2_windo Enter 1 or 2 (1. New Registration 2. Login):2 You entered option 2 Enter Details User Name: 305 student Password: 1234 Wrong Credentials Enter User Name: athirai Enter Password4568 Wrong Credentials Enter User Name: athirai Enter Password1234 Login Successfull