Question
This homework will focus on String manipulation, interfaces and recursion. We will be creating a StringHelpers class that allows us to perform interesting operations on
This homework will focus on String manipulation, interfaces and recursion. We will be creating a StringHelpers class that allows us to perform interesting operations on String objects. Remember, the String class is immutable!
Interface
Write an interface called StringOperations with the following methods:
Name | Return type | Parameters | Description |
containsVowel | boolean | String searchString | Takes a String input and returns whether the String contains a vowel or not: "hello world!" would return true "twyndyllyngs" would return false |
containsConsonant | boolean | String searchString | Takes a String input and returns whether the String contains a consonant or not: "hello world!" would return true "aeaea" would return false |
printLengthyWords | void | String searchString | Prints all words in the String, one per line on the console, that have over five characters "the lazy do pranced and danced all over the yard" would print out: pranced danced |
printNumberOfCharacters | int | String searchString, char searchCharacter | Prints the number of times searchCharacter shows up searchString: If searchString is "President Obama is interested in net neutrality" and searchCharacter is "e" then printNumberOfCharacters would return 7. If searchString is "the world is flat" and searchCharacter is "u" then printNumberOfCharacters would return 0. |
findPalindrome | boolean | String searchString | Takes a String input and returns whether the String is a palindrome. A palindrome is word that is the same when spelled backwards (ignoring spaces and punctuation). For example: "civic", "radar", "deleveled", "borrow or rob", "a man, a plan, a canal, panama" would all return true. "pizza" would return false. |
StringHelpers Class
Write a class called StringHelpers that implements the StringOperations interface above.
containsVowel: you can use the indexOf() or contains() method to identify vowels
containsConsonant: you can use the indexOf() or contains() method to identify consonants
Note: this can be completed using only one line of code (take a moment and consider this)
printLengthyWords: use the split() method to identify words and use the length() method to determine word length
Note: first focus on retrieving the words, then worry about word length
printNumberOfCharacters: this method should be solved using recursion
You will need to use the indexOf() method to determine where the first searchCharacter is at in your searchString
You will need to keep track of a counter that counts the number of characters seen so far. You will then return this counter variable with each recursive call.
Your base case should be when the character is no longer found in searchString.
findPalindrome: this method sould be solved using recursion
To be a palindrome, the first and last character of a string must be equal: "deleveled"
Your recursive method should first check that these characters are equal and then pass the remaining string back to the method recursively "elevele" would be checked next, then "level", then "eve"...
Your base case will be when you get a string with one character "v" or no characters (a string with an even number of characters
Driver Class
Write a driver class that creates a StringHelpers object and lets the user interact with methods above on the console. You should ask the user for which method they wish to call, then give the user a chance to enter an parameters the method requires. Print the results of the method call if the method has a return type.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
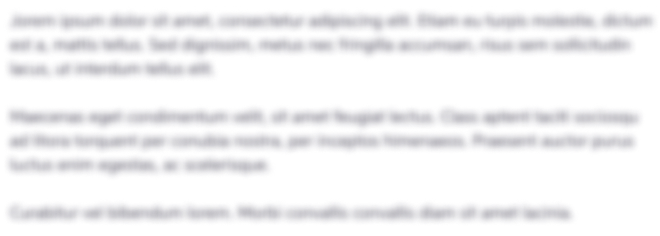
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started