Question
This Individual Assignment is a set of three problems. The first is a recursion warm up exercise, and the second two are QuickSort variations. The
This Individual Assignment is a set of three problems. The first is a recursion "warm up" exercise, and the second two are QuickSort variations. The "warm up" should be implemented as a static method in your main App class and the second two will use additional classes (as instructed below). All three problems should be included in the same NetBeans project (exported to ZIP as usual).
-----------------
All of your classes should be properly encapsulated, follow all proper coding conventions discussed in class, and be logically constructed following good Object Oriented design. Your submitted code should compile and run without errors.
-----------------
Problem #1: The Recursion Warm Up (10 points):
Write a recursive method to reverse and capitalize a String. For example:
reverseAndCap("abcdefg3!")
should return:
!3GFEDCBA
Note that non-alphabetic characters are included (but obviously cannot be capitalized).
Also, no points will be given for a non-recursive solution.
Problem #2: The Array CatSort (45 points):
- Create a "Cat" class with the following attributes:
- Name
- Weight
- Age
Include a constructor and getter methods, and override toString() to display all info (you may let NetBeans do this for you).
- In your main method, create an array of 5 Cats and initialize their attributes.
- Implement the QuickSort algorithm (as shown in class) to sort Cats by weight. You may create this as a static recursive method.
- Display the sorted array contents in main.
Problem #3: The Linked List CatSort (45 points):
- Modify the Linked List example that you will be given in class into a LinkedCatList class that implements a Linked List of the Cat objects that you created in Problem #2.
- In your main method, create a LinkedCatList of 5 Cats and initialize their attributes.
- Create a sort method in your LinkedCatList class that uses QuickSort (implemented by you) to sort the list of Cats alphabetically (lexicographicaly) by Name.
- Display the sorted list contents in main.
This is the LinkedList Starter code that I am supposed to use for #3
import java.util.NoSuchElementException; public class LinkedStringList { private Node first; private Node currentNode; private int length; class Node { private String data; private Node next; public void printNodeData() { System.out.println("Node data: " + data); } public Node getNext() { return next; } } public LinkedStringList() { first = null; currentNode = null; length = 0; } public void setFirst(String value) { first.data = value; } public void setCurrent(String value) { currentNode.data = value; } public void addFirst(String value) { //create the Node and set its value Node newNode = new Node(); newNode.data = value; // if newNode is the first node, this will be null // otherwise it will point to the former "first" now newNode.next = first; //set our "first" Node to the Node we just created first = newNode; currentNode = newNode; length++; } public void removeFirst() { if (first == null) { throw new NoSuchElementException(); } else { first = first.next; length--; } } public void remove() { if (currentNode == first) { removeFirst(); } else { Node tempCurrent = currentNode; moveFirst(); boolean found = false; while (!found) { if(currentNode.next == tempCurrent) { found = true; } else { moveNext(); } }
currentNode.next = tempCurrent.next; } }
public int indexOf(String value) { Node tempNode = first; int position = 0; while (tempNode != null) { if (tempNode.data == value) { return position; } else { tempNode = tempNode.next; position++; } } return -1; } //adds after currentNode public void add(String value) { if (first == null) { addFirst(value); } else {
//create node having "next" pointing to tempSwap Node newNode = new Node(); newNode.data = value; newNode.next = currentNode.next; //make the old current node point to our newNode currentNode.next = newNode; //move current refer to node we just added moveNext(); //increment length of list length++; }
} public boolean isEmpty() { return (first == null); } public int getLength() { return length; } public String getFirstValue() { if (first == null) { throw new NoSuchElementException(); } else { return first.data; } } public String getCurrentValue() { if (currentNode == null) { throw new NoSuchElementException(); } else { return currentNode.data; } } public void moveNext() { currentNode = currentNode.next; } public void moveFirst() { currentNode = first; } public void displayList() { Node currentNode = first; System.out.println("List contents:"); while (currentNode != null) { currentNode.printNodeData(); currentNode = currentNode.getNext(); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
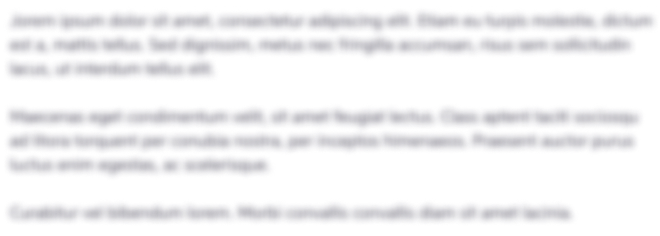
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started