Question
This is a c++ class involving ADT in which I need to implement a specific function into my program. This program has two files, ArrayBag.hpp
This is a c++ class involving ADT in which I need to implement a specific function into my program. This program has two files, ArrayBag.hpp and ArrayBag.cpp.
I need to implement the following public member functions:
/** @param a_bag to be combined with the contents of this bag @return a new ArrayBag that contains all elements from this bag (items_) and as many elements from the argument a_bag as space requires. Note that it may contain duplicates */
ArrayBag
//Please note that because ArrayBag is of fixed size, bagUnion will copy the contents of the calling bag first and then whatever fits from the argument a_bag.
CourseMember.cpp
------------------------------------------------------
#include
CourseMember::CourseMember(int id, std::string first, std::string last) { id_ = id; first_name_ = first; last_name_ = last; }
//Return ID int CourseMember::getID() const { return id_; }
/** @return returns first_name_ */ string CourseMember::getFirstName() const { return first_name_; }
/** @return returns last_name_ */ string CourseMember::getLastName() const { return last_name_; }
---------------------------------------------------------------
ArrayBag.hpp
------------------------------------------
/** Header file for an array-based implementation of the ADT bag. @file ArrayBag.h */
#ifndef ARRAY_BAG_ #define ARRAY_BAG_
#include
template
public: /** default constructor**/ ArrayBag(); /** @return item_count_ : the current size of the bag **/ int getCurrentSize() const; /** @return true if item_count_ == 0, false otherwise **/ bool isEmpty() const; /** @return true if new_etry was successfully added to items_, false otherwise **/ bool add(const T& new_entry); /** @return true if an_etry was successfully removed from items_, false otherwise **/ bool remove(const T& an_entry); /** @post item_count_ == 0 **/ void clear(); /** @return true if an_etry is found in items_, false otherwise **/ bool contains(const T& an_entry) const; /** @return the number of times an_entry is found in items_ **/ int getFrequencyOf(const T& an_entry) const; /** @return a vector having the same cotntents as items_ **/ std::vector
private: static const int DEFAULT_CAPACITY = 200; //max size of items_ T items_[DEFAULT_CAPACITY]; // Array of bag items int item_count_; // Current count of bag items /** @param target to be found in items_ @return either the index target in the array items_ or -1, if the array does not containthe target. **/ int getIndexOf(const T& target) const; }; // end ArrayBag
#include "ArrayBag.cpp" #endif
---------------------------------------------
ArrayBag.cpp
-------------------------------------------------
/** Implementation file for the class ArrayBag. @file ArrayBag.cpp */ #include "ArrayBag.hpp" #include/** default constructor**/ template ArrayBag ::ArrayBag(): item_count_(0) { } // end default constructor /** @return item_count_ : the current size of the bag **/ template int ArrayBag ::getCurrentSize() const { return item_count_; } // end getCurrentSize /** @return true if item_count_ == 0, false otherwise **/` template bool ArrayBag ::isEmpty() const { return item_count_ == 0; } // end isEmpty /** @return true if new_etry was successfully added to items_, false otherwise **/ template bool ArrayBag ::add(const T& new_entry) { bool has_room = (item_count_ < DEFAULT_CAPACITY); if (has_room) { items_[item_count_] = new_entry; item_count_++; } // end if return has_room; } // end add /** @return true if an_etry was successfully removed from items_, false otherwise **/ template bool ArrayBag ::remove(const T& an_entry) { int found_index = getIndexOf(an_entry); bool can_remove = !isEmpty() && (found_index > -1); if (can_remove) { item_count_--; items_[found_index] = items_[item_count_]; } // end if return can_remove; } // end remove /** @post item_count_ == 0 **/ template void ArrayBag ::clear() { item_count_ = 0; } // end clear /** @return the number of times an_entry is found in items_ **/ template int ArrayBag ::getFrequencyOf(const T& an_entry) const { int frequency = 0; int cun_index = 0; // Current array index while (cun_index < item_count_) { if (items_[cun_index] == an_entry) { frequency++; } // end if cun_index++; // Increment to next entry } // end while return frequency; } // end getFrequencyOf /** @return true if an_etry is found in items_, false otherwise **/ template bool ArrayBag ::contains(const T& an_entry) const { return getIndexOf(an_entry) > -1; } // end contains /** @return a vector having the same cotntents as items_ **/ template std::vector ArrayBag ::toVector() const { std::vector bag_contents; for (int i = 0; i < item_count_; i++) bag_contents.push_back(items_[i]); return bag_contents; } // end toVector // PRIVATE /** @param target to be found in items_ @return either the index target in the array items_ or -1, if the array does not containthe target. **/ template int ArrayBag ::getIndexOf(const T& target) const { bool found = false; int result = -1; int search_index = 0; // If the bag is empty, item_count_ is zero, so loop is skipped while (!found && (search_index < item_count_)) { if (items_[search_index] == target) { found = true; result = search_index; } else { search_index++; } // end if } // end while return result; } // end getIndexOf
-------------------------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
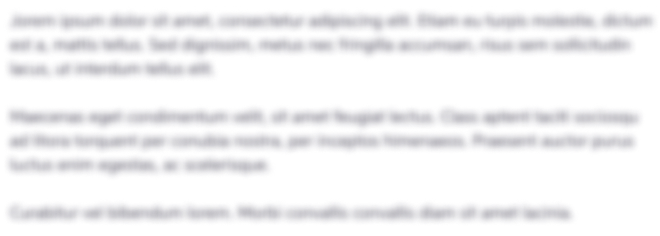
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started