Question
This is a C PROGRAM. I have some missing parts in my program from the GRADING RUBRIC attached below. Can you please fix it and
This is a C PROGRAM. I have some missing parts in my program from the GRADING RUBRIC attached below. Can you please fix it and make sure everything in the grading rubric is in the code and works? I really need to get 100 points in this program, much appreciated!
I attached the Grading Rubric and the Program.
Thanks a lot!
---------------------------------------------------------
The Code:
#include "Header.h"
struct namerecord {
char first[25];
char last[25];
};
struct payrecord {
int id;
struct namerecord name;
float hoursWorked;
float hourlyRate;
float regularPay;
float overtimePay;
float gross;
float tax;
float netPay;
};
typedef struct payrecord payrecord;
int getRecords(payrecord payroll[], int max);
int getSelection();
double calcPayroll(payrecord payroll[], int n, double * tax);
void printAllRecords(payrecord payroll[], int n);
void getName(payrecord payroll[], int i);
void printGrossPayroll(double gross, double tax);
void displayMenu();
int main()
{
int userSelection;
int i = 0, n = 0, numOfEmployees = 0;
double gross = 0, tax = 0;
payrecord payroll[MAX];
// Ask the user how many employees to add
printf("How many employees do you want to add? ");
scanf("%d%*c", &numOfEmployees);
do
{
userSelection = getSelection();
switch (userSelection)
{
case 1:
CLS;
// Get the employee information from the user
n = getRecords(payroll, numOfEmployees);
PAUSE;
break;
case 2:
CLS;
gross = calcPayroll(payroll, n, &tax);
printAllRecords(payroll, n);
PAUSE;
break;
case 3:
CLS;
printGrossPayroll(gross, tax);
PAUSE;
break;
default:
PAUSE;
break;
}
} while (userSelection != 4);
return 0;
}
void displayMenu()
{
CLS;
printf("=========== PAYROLL PROGRAM =========== ");
printf("=========== Menu =========== ");
printf("1. Add an employee ");
printf("2. Display ALL employees ");
printf("3. Display GROSS Payroll ");
printf("4. Quit ");
printf("======================================= ");
printf("Enter your selection: ");
} // end displayMenu()
int getSelection()
{
int result;
displayMenu();
scanf("%i", &result);
fflush(stdin);
return result;
} // end getSelection()
int getRecords(payrecord payroll[], int max)
{
int i, j, n;
float z;
// Ask the user for the employee name
void getName(payrecord payroll[], int i);
// Loop array of structs
for (i = 0; i
{
printf("ID Number: ");
j = scanf("%d%*c", &n);
// Error checking
if (j == EOF)
return i;
payroll[i].id = n;
getName(payroll, i);
printf("Hours Worked: ");
j = scanf("%f%*c", &z);
payroll[i].hoursWorked = z;
printf("Hourly Rate: ");
j = scanf("%f%*c", &z);
payroll[i].hourlyRate = z;
} // end for loop
return i;
}
void getName(payrecord payroll[], int i)
{
printf("First Name: ");
gets(payroll[i].name.first);
printf("Last Name: ");
gets(payroll[i].name.last);
}
double calcPayroll(payrecord payroll[], int n, double * tax)
{
int i;
double gross = 0;
*tax = 0;
// Loops through struct and calculates if an employee worked under 40 hours.
// If an employee worked under 40 hours then calculate hoursWorked * hourlyWorked
// Else get the amount of overtime worked and multiply hourly rate * 1.5
// After calculating the gross income calculate the amount of tax to remove based
// on income.
// Tax removed from gross pay:
// 10% if under 2000
// 15% if under 5000
// 25% if over 5000
for (i = 0; i
{
if (payroll[i].hoursWorked
{
payroll[i].regularPay = payroll[i].gross = payroll[i].hoursWorked * payroll[i].hourlyRate;
payroll[i].overtimePay = 0;
}
else {
payroll[i].regularPay = 40 * payroll[i].hourlyRate;
payroll[i].overtimePay = (payroll[i].hoursWorked - 40) * 1.5 * payroll[i].hourlyRate;
}
// Gross pay
payroll[i].gross = payroll[i].regularPay + payroll[i].overtimePay;
// Tax bracket
if (payroll[i].gross
{
payroll[i].tax = payroll[i].gross * 0.1;
}
else if (payroll[i].gross
{
payroll[i].tax = payroll[i].gross * 0.15;
}
else if (payroll[i].gross > 5000)
{
payroll[i].tax = payroll[i].gross * 0.25;
}
gross += payroll[i].gross;
*tax += payroll[i].tax;
// Calculate the netPay by subtracting the grossPay with the tax taken out
payroll[i].netPay = payroll[i].gross - payroll[i].tax;
}
return gross;
}
void printAllRecords(payrecord payroll[], int n)
{
int i, x;
float z;
printf("===== Employee List ===== ");
for (i = 0; i
{
printf("ID Number: %d ", payroll[i].id);
printf("Name: %s %s ", payroll[i].name.first, payroll[i].name.last);
printf("Hours Worked: %.2f ", payroll[i].hoursWorked);
printf("Overtime Pay: $%.2f ", payroll[i].overtimePay);
printf("Hourly Rate: $%.2f ", payroll[i].hourlyRate);
printf("Gross Pay: $%.2f ", payroll[i].gross);
printf("Tax Paid: $%.2f ", payroll[i].tax);
}
}
void printGrossPayroll(double gross, double tax)
{
printf("==== TOTAL PAYROLL ==== ");
printf("Gross Employee Pay: $%.2f ", gross);
printf("Gross Company Tax: $%.2f ", tax);
}
Possible 2 Theme for the program (the purpose)(The Specification Docs) 3 Design tool 4 Documentation with in the code 5 Use of functions (everything is a function) 6 Names of functions (they make sense and are self documenting) 7 Your struct (see above for the rule) 5 5 5 8 Your menu system 9Adds to the Array 10Removes from the Array 11Does sorting 12Does searching 13Does some type of calculations 14 Allows the user to enter the number of things to store 15Allocates disjoint memory and uses this memory 16 Output is neat and makes sense 17Compiles and runs 18Error checking...stops the user from doing bad things 19Freeing Memory Correctly 20 Completed on time and properly uploaded to Blackboard 21 Uses a programmer created header file 5 5 5 5 5 5 100 23Step by Step Solution
There are 3 Steps involved in it
Step: 1
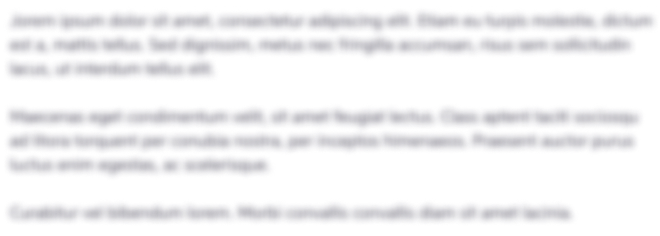
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started