Question
This is a Java program - I need help figuring out why the interrupt thread works but the uninterrupted one doesn't. I have looked at
This is a Java program - I need help figuring out why the interrupt thread works but the uninterrupted one doesn't. I have looked at several examples but still can't figure it out.
Using the concepts from the Concurrency Basics Tutorial I provided in Modules, write a program that consists of two threads. The first is the main thread that every Java application has. The main thread should create a new thread from the Runnable object, MessageLoop, and wait for it to finish. If the MessageLoop thread takes too long to finish, the main thread should interrupt it. Use a variable named maxWaitTime to store the maximum number of seconds to wait. The main thread should output a message stating that it is still waiting every second (or a different value so that it will output this message a few times, depending on your other wait times).
The MessageLoop thread should print out a series of 5 messages. It should wait a few seconds between printing messages to create a delay. Make the value sufficiently high to cause main to output Continuing to wait messages. If it is interrupted before it has printed all its messages, the MessageLoop thread should print "Message loop interrupted" and exit.
Your program must demonstrate that it can both output messages and interrupt the message output. To do this, place your code in main into a for loop using maxWaitTime as the index. Choose values that will demonstrate this. You can iterate this loop 2 times or 10 times, as many as needed to demonstrate the above requirement.
This is what the output should look like:
Sample output :
maxWaitTime : 1 second(s)
main: Starting MessageLoop thread main: Waiting for MessageLoop thread to finish main: Continuing to wait... main: Interrupting Thread-0: Message loop interrupted main: Finished!
maxWaitTime : 2 second(s)
main: Starting MessageLoop thread main: Waiting for MessageLoop thread to finish main: Continuing to wait... main: Continuing to wait... Thread-0: Message 1 main: Continuing to wait... main: Continuing to wait... Thread-0: Message 2 main: Continuing to wait... main: Continuing to wait... Thread-0: Message 3 main: Continuing to wait... main: Continuing to wait... Thread-0: Message 4 main: Continuing to wait... main: Continuing to wait... Thread-0: Message 5 main: Finished!
package thread;
public class MainThread { public static void main(String[] args) { // TODO Auto-generated method stub class MainThread { public void main(String[] args) { // TODO Auto-generated method stub Thread t1 = new Thread((Runnable) new MainThread()); Thread t2 = new Thread((Runnable) new MainThread()); t1.start(); t2.start(); } } } } class MessageLoop implements Runnable { public void run() { try { for(int i=1;i<=5;i++){ Thread.sleep(2100); System.out.println("Thread-0: Message "+(i)); } } catch (InterruptedException ex) { System.out.println("Thread-0: Message loop interrupted"); } } public static void main(String[] args) { System.out.println("main: Starting MessageLoop thread"); //create a thread Thread t1 = new Thread(new MessageLoop()); //start the thread t1.start(); System.out.println("main: Waiting for MessageLoop thread to finish"); //until thread alive while(t1.isAlive()){ try { //1 second delay and print message Thread.sleep(1000); System.out.println("main: Continuing to wait..."); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } System.out.println("main: Finished!"); } }class MessageLoop2 implements Runnable { public void run() { try { for(int i=1;i<=5;i++){ Thread.sleep(2100); System.out.println("Thread-0: Message "+(i)); } } catch (InterruptedException ex) { System.out.println("Thread-0: Message loop interrupted"); } } public static void main(String[] args) { System.out.println("main: Starting MessageLoop thread"); //create a thread Thread t1 = new Thread(new MessageLoop()); //start the thread t1.start(); System.out.println("main: Waiting for MessageLoop thread to finish"); //until thread alive while(t1.isAlive()){ try { //1 second delay and print message System.out.println("main: Continuing to wait..."); System.out.println("main: Interrupting"); t1.interrupt(); Thread.sleep(1000); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } System.out.println("main: Finished!"); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
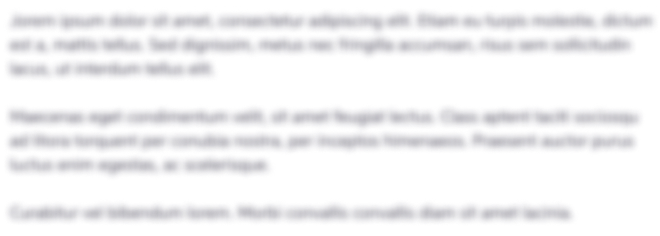
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started