Question
This is a java programming assignment that I am having trouble getting to loop back up to the add more ingredients prompt. I have tried
This is a java programming assignment that I am having trouble getting to loop back up to the add more ingredients prompt. I have tried adding the following code but keep getting "missing return statement" errors. So I have resorted to repeating the whole code twice just to get a couple of ingredients! :
do { System.out.println("Please enter the ingredient name or type 'e' if you are done: ");
String ingredientName = scnr.next();
if (ingredientName.toLowerCase().equals("e")) {
addMoreIngredients = false;
} else {
Ingredient tempIngredient = new Ingredient().enterNewIngredient(ingredientName);
recipeIngredients.add(tempIngredient);
}
} while (addMoreIngredients); ....
CANT GET THIS TO WORK WITH ALL THE OTHER WHILE STATEMENTS USED TO VALIDATE INPUT!
I am also having trouble figuring out how to add the following components: I have added the instance variable accessors, mutators but can't seem to get much further because I can't get the original code to loop back to add more ingredients. Here is the code I was given to build on:
public class SteppingStone6_RecipeBox {
/** * Declare instance variables: * a private ArrayList of the type SteppingStone5_Recipe named listOfRecipes * */
/** * Add accessor and mutator for listOfRecipes * */
/** * Add constructors for the SteppingStone6_RecipeBox() * */
/** * Add the following custom methods: *
* //printAllRecipeDetails(SteppingStone5_Recipe selectedRecipe) * This method should accept a recipe from the listOfRecipes ArrayList * recipeName and use the SteppingStone5_Recipe.printRecipe() method * to print the recipe
* * //printAllRecipeNames() <-- This method should print just the recipe * names of each of the recipes in the listOfRecipes ArrayList * *
//addRecipe(SteppingStone5_Recipe tmpRecipe) <-- This method should use * the SteppingStone5_Recipe.addRecipe() method to add a new * SteppingStone5_Recipe to the listOfRecipes * */
/** * A variation of following menu method should be used as the actual main * once you are ready to submit your final application. For this * submission and for using it to do stand-alone tests, replace the * public void menu() with the standard * public static main(String[] args) * method * */ public void menu() {
// Create a Recipe Box //SteppingStone6_RecipeBox myRecipeBox = new SteppingStone6_RecipeBox();
//Uncomment for main method Scanner menuScnr = new Scanner(System.in);
/** * Print a menu for the user to select one of the three options:
* */ System.out.println("Menu " + "1. Add Recipe " + "2. Print All Recipe Details " + "3. Print All Recipe Names " + " Please select a menu item:");
while (menuScnr.hasNextInt() || menuScnr.hasNextLine()) {
System.out.println("Menu " + "1. Add Recipe " + "2. Print All Recipe Details " + "3. Print All Recipe Names " + " Please select a menu item:");
int input = menuScnr.nextInt();
/** * The code below has two variations: *
1. Code used with the SteppingStone6_RecipeBox_tester. *
2. Code used with the public static main() method * * One of the sections should be commented out depending on the use. */
/** * This could should remain uncommented when using the * SteppingStone6_RecipeBox_tester. *
* Comment out this section when using the * public static main() method */
if (input == 1) { newRecipe(); } else if (input == 2) {
System.out.println("Which recipe? ");
String selectedRecipeName = menuScnr.next();
printAllRecipeDetails(selectedRecipeName);
} else if (input == 3) { for (int j = 0; j < listOfRecipes.size(); j++) {
System.out.println((j + 1) + ": " + listOfRecipes.get(j).getRecipeName());
}
} else {
System.out.println(" Menu " + "1. Add Recipe " + "2. Print Recipe " + "3. Adjust Recipe Servings " + " Please select a menu item:"); }
/** * This could should be uncommented when using the * public static main() method * * Comment out this section when using the * SteppingStone6_RecipeBox_tester. *
if (input == 1) { myRecipeBox.newRecipe();
} else if (input == 2) {
System.out.println("Which recipe? ");
String selectedRecipeName = menuScnr.next();
myRecipesBox.printAllRecipeDetails(selectedRecipeName);
} else if (input == 3) { for (int j = 0; j < myRecipesBox.listOfRecipes.size(); j++) {
System.out.println((j + 1) + ": " + myRecipesBox.listOfRecipes.get(j).getRecipeName());
}
} else {
System.out.println(" Menu " + "1. Add Recipe " + "2. Print Recipe " + "3. Adjust Recipe Servings " + " Please select a menu item:"); } * */
System.out.println("Menu " + "1. Add Recipe " + "2. Print All Recipe Details " + "3. Print All Recipe Names " + " Please select a menu item:"); } } } /** * *
Final Project Details: * * For your final project submission, you should add a menu item and a method * to access the custom method you developed for the Recipe class * based on the Stepping Stone 5 Lab. * */
Here is my code:
package ultimaterecipebuilder;
import java.util.Scanner; import java.util.ArrayList;
/** * * @author
*/
public class UltimateRecipeBuilder {
private String recipeName;
private double totalRecipeCalories;
private ArrayList recipeIngredients;
public void add(int index, String element) {
listOfRecipes.add(0, recipeName);
}
private ArrayList listOfRecipes;
public UltimateRecipeBuilder(ArrayList listOfRecipes) {
this.listOfRecipes = listOfRecipes;
}
public ArrayList getListOfRecipes() {
return listOfRecipes;
}
public void setListOfRecipes(ArrayList listOfRecipes) {
this.listOfRecipes = listOfRecipes;
}
public void printListOfRecipes() {
for (String recipeName : listOfRecipes) {
System.out.println(recipeName);
}
}
public void printAllRecipeDetails() {
listOfRecipes.stream().forEach((_item) -> { printRecipe(); });
}
private double servings;
private double ingredientAmount;
public UltimateRecipeBuilder() {
this.recipeName = "";
this.servings = 0;
this.recipeIngredients = new ArrayList<>();
this.totalRecipeCalories = 0;
this.ingredientAmount = 0;
}
public UltimateRecipeBuilder(String recipeName, double servings, ArrayList recipeIngredients, double totalRecipeCalories) {
// for final project add ingredient amount per serving
this.recipeName = recipeName;
this.servings = servings;
this.recipeIngredients = recipeIngredients;
this.totalRecipeCalories = totalRecipeCalories;
this.ingredientAmount = ingredientAmount;
}
public String getRecipeName() {
return recipeName;
}
public void setRecipeName(String recipeName)
{
this.recipeName = recipeName;
}
public double getTotalRecipeCalories()
{
return totalRecipeCalories;
}
public void setTotalRecipeCalories(double totalRecipeCalories)
{
this.totalRecipeCalories = totalRecipeCalories;
}
public ArrayList getRecipeIngredients()
{
return recipeIngredients;
}
public void setRecipeIngredients(ArrayList recipeIngredients)
{
this.recipeIngredients = recipeIngredients;
}
public double getServings()
{
return servings;
}
public void setServings(double servings)
{
this.servings = servings;
} public double getingredientAmount()
{
return getingredientAmount();
}
public void setIngredientAmount(double ingredientAmount)
{
this.ingredientAmount = ingredientAmount;
}
public void printRecipe()
{
// print method to display recipe at end of code
double singleServingCalories = (double) (totalRecipeCalories / servings);
System.out.println("Recipe: " + recipeName);
System.out.println("Serves: " + servings);
System.out.println("Ingredients:");
for (String ingredient : recipeIngredients) {
System.out.println(ingredient);
}
System.out.println("Each serving has " + singleServingCalories + " Calories.");
}
public static void main(String[] args) {
// method creates new recipe
UltimateRecipeBuilder recipe1 = createNewRecipe();
recipe1.printRecipe();
}
public static UltimateRecipeBuilder createNewRecipe() {
Scanner scnr = new Scanner(System.in);
ArrayList recipeIngredients = new ArrayList();
ArrayList listOfRecipes = new ArrayList();
double totalRecipeCalories = 0; // total of calories from all ingredients
double ingredientCalories = 0; // # calories per each ingredient
double ingredientAmount = 0; // amount of ingredient defined by unit of measure (cup, tsp, etc.)
double servings = 0; // # servings per recipe
String unitMeasurement = ""; // how ingredient is measured
String ingredientName = ""; // name of ingredient
boolean addMoreIngredients = false: //could not get this to work in code
System.out.println("Please enter the recipe name: ");
String recipeName = scnr.nextLine(); // gets name of recipe
while (!recipeName.matches("[a-zA-Z_]+")) {
System.out.println("Invalid name, use letters only");
recipeName = scnr.nextLine(); // validates user input is letters, repeats statement until letters entered
}
System.out.println("Please enter the number of servings: "); // gets # servings
while (!scnr.hasNextDouble()) { // verifies input is pos #
System.out.println("Please enter the number of servings" + ". Use numbers only "); // validates user inputs numbers for servings, repeats until numbers entered
scnr.nextLine();
} servings = scnr.nextDouble();
scnr.nextLine();
System.out.println("Please enter an ingredient name using letters only. Enter 'e' if you are finished"); // gets first ingredient
ingredientName = scnr.next();
while (!ingredientName.matches(".*[a-z].*")) {
System.out.println("Please enter an ingredient name. Use letters only");
ingredientName = scnr.next(); // validates user input is letters, repeats until letters entered
}
recipeIngredients.add(ingredientName); // adds ingredient to array list
System.out.println("Please enter the unit of measure for the" + " ingredient (ex.- cup, oz, tsp, tbsp, pt). Use only" + " letters, no numbers: "); // gets unit of measurement for ingredient (cup, oz, tsp. etc.)
unitMeasurement = scnr.nextLine();
while (!unitMeasurement.matches( ".*[a-z].*")) { //validates input is letters
System.out.println("Please enter the unit of measure for the" + " ingredient (ex.- cup, oz, tsp, tbsp, pt). Use only" + " letters, no numbers: "); //validates user input is letters, repeats until letters entered
unitMeasurement = scnr.nextLine(); }
System.out.println("How many " + unitMeasurement + "s of " + ingredientName + "?. Enter numbers only!"); // gets ingredient amount
while (!scnr.hasNextDouble()) {
System.out.println("How many " + unitMeasurement + "s of " + ingredientName + "?. Enter numbers only!"); // validates user input is numbers for amount, repeats until numbers entered
scnr.nextLine();
}
ingredientAmount = scnr.nextDouble();
System.out.println("Please enter the number of calories per " + unitMeasurement + ". Use numbers only!"); // gets # calories per amount of ingredient
while (!scnr.hasNextDouble()) { System.out.println("Please enter the number of calories per " + unitMeasurement + ". Use numbers only!"); // validates user input # calories is numbers, repeats until number entered
scnr.nextLine();
}
ingredientCalories = scnr.nextDouble();
scnr.nextLine();
totalRecipeCalories += (ingredientCalories * ingredientAmount); // calculates total calories for this ingredient-
// pseudocode for final project: CALCULATE ingredient amount per serving = ingredientAmount / servings
System.out.println("Please enter an ingredient name. Use letters only");
ingredientName = scnr.nextLine();
while (!ingredientName.matches(".*[a-z].*"))
{
System.out.println("Please enter an ingredient name. Use letters only");
ingredientName = scnr.nextLine();
}
recipeIngredients.add(ingredientName);
System.out.println("Please enter the unit of measure for the" + " ingredient (ex.- cup, oz, tsp, tbsp, pt). Use only" + " letters, no numbers: "); unitMeasurement = scnr.nextLine();
while (!unitMeasurement.matches(".*[a-z].*")) { //validates input is letters
System.out.println("Please enter the unit of measure for the" + " ingredient (ex.- cup, oz, tsp, tbsp, pt). Use only" + " letters, no numbers: "); unitMeasurement = scnr.nextLine();
}
System.out.println("How many " + unitMeasurement + "s of " + ingredientName + "?. Enter numbers only!");
while (!scnr.hasNextDouble()) {
System.out.println("How many " + unitMeasurement + "s of " + ingredientName + "?. Enter numbers only!");
scnr.nextLine();
}
ingredientAmount = scnr.nextDouble();
scnr.nextLine();
System.out.println("Please enter the number of calories per " + unitMeasurement + ". Use numbers only!");
while (!scnr.hasNextDouble())
{
System.out.println("Please enter the number of calories per " + unitMeasurement + ". Use numbers only!");
scnr.nextLine();
}
ingredientCalories = scnr.nextDouble();
scnr.nextLine();
totalRecipeCalories += (ingredientAmount * ingredientCalories); // pseudocode for final project: CALCULATE ingredient amount per serving = ingredientAmount / servings
UltimateRecipeBuilder recipe1 = new UltimateRecipeBuilder(recipeName, servings, recipeIngredients, totalRecipeCalories); // creates recipe- name, # servings, ingredients, total calories, calories per serving
// final project will add ingredientAmount per serving
return recipe1; // prints recipe }
}
Thanks in advance for any advice, I'm stuck!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
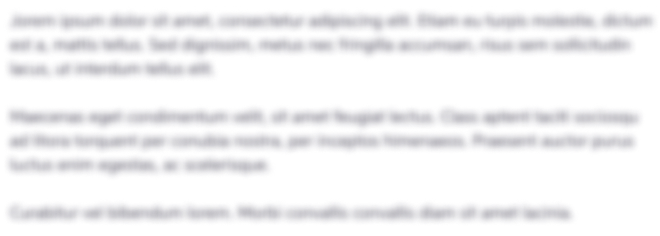
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started