Question
This is a LinkedList class, but I am having a bit of trouble with some methods, can you see the problem? public class LinkedList implements
This is a LinkedList class, but I am having a bit of trouble with some methods, can you see the problem?
public class LinkedList
private class Node
private T data;
private Node
public Node (T data) {
this.data = data;
this.link = link;
}
}
private Node
private Node
private Node
private Node
private boolean found;
private int data;
private LinkedList
private void find(T target) {
targetNode = firstNode;
trailTargetNode = null;
found = false;
while(targetNode !=null && !targetNode.data.equals(target)) {
trailTargetNode= targetNode;
targetNode= targetNode.link;
}
if(targetNode == null)
return;
else
found = true;
return;
}
public LinkedList() {
firstNode = lastNode = null;
}
public LinkedList(LinkedList
if(!otherList.isEmpty()) {
this.reset();
T dataValue;
Node
while(currentNode != null) {
dataValue = currentNode.data;
this.insert(dataValue);
currentNode = currentNode.link;
}
}
}
/**
*
* Reverse list.
*
*/
/* Function to reverse the linked list */
void reverse() {
Node prev = null;
Node current = firstNode;
Node next = null;
while (current != null) {
next = current.link;
current.link = prev;
prev = current;
current = next;
}
firstNode = prev;
}
/*
* N th Item on List
*/
public Object nthItem(int n)
{
Node current = firstNode;
int count = 0;
while (current != null)
{
if (count == n)
return current.data;
count++;
current = current.link;
}
if(count < n ){
System.out.println("There is no "+n+"th item on the list");
}
return 0;
}
@Override
public int size() {
int nodeCount = 0;
Node
if(isEmpty())
return nodeCount;
while(currentNode != null) {
nodeCount++;
currentNode = currentNode.link;
}
return nodeCount;
}
@Override
public void reset() {
firstNode = lastNode= null;
return;
}
@Override
public boolean isEmpty() {
return (firstNode == null);
}
public boolean insert(T element) {
Node
if(this.isEmpty()) {
firstNode = lastNode = newNode;
return true;
}
lastNode.link = newNode;
lastNode = lastNode.link;
return true;
}
public void insertAtFront(T element ) {
Node
if(this.isEmpty()) {
firstNode = lastNode = newNode;
return;
}
newNode.link = firstNode;
firstNode = newNode;
return;
}
public Node sort(Node headOriginal) {
if (headOriginal == null || headOriginal.link == null)
return headOriginal;
Node a = headOriginal;
Node b = headOriginal.link;
while ((b != null) && (b.link != null)) {
headOriginal = headOriginal.link;
b = (b.link).link;
}
b = headOriginal.link;
headOriginal.link = null;
return merge(sort(a), sort(b));
}
public Node merge(Node a, Node b) {
Node temp = new Node();
Node head = temp;
Node c = head;
while ((a != null) && (b != null)) {
if (a.data <= b.data) {
c.link = a;
c = a;
a = a.link;
} else {
c.link = b;
c = b;
b = b.link;
}
}
c.link = (a == null) ? b : a;
return head.link;
}
public void divide(LinkedList otherList, int value) {
Node temp = this.getFirst();
if (temp != null && temp.data == data) {
this.setFirst(null);
otherList.setFirst(temp);
return;
}
while (temp.link != null) {
if(temp.link.data == data) {
otherList.setFirst(temp.link);
temp.link = null;
return;
}
temp = temp.link;
}
}
public Node getFirst() {
return firstNode;
}
public void setFirst(Node first) {
this.firstNode = firstNode;
}
@Override
public boolean remove(T target) {
find(target);
if (!found)
return false;
if(target == firstNode) {
firstNode = firstNode.link;
return true;
}
if(size()==1) {
reset();
return true;
}
if(targetNode == lastNode) {
lastNode = trailTargetNode;
lastNode.link = null;
return true;
}
return false;
}
@Override
public boolean contains(T target) {
find(target);
return found;
}
public String toString() {
String str = "";
if (isEmpty()) {
str = "List is Empty";
return str;
}
Node
while(current!=null) {
str = str + current.data + "\t";
current = current.link;
}
return str;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
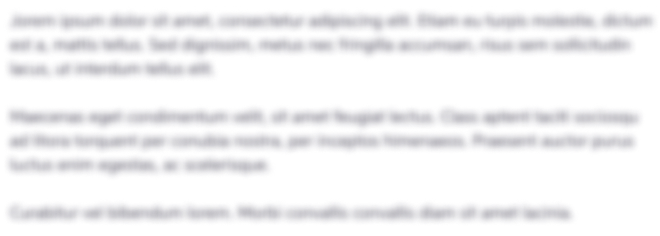
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started