Question
THIS IS A LONG QUESTION. PLEASE READ EVERYTHING IN FULL BEFORE ANSWERING. THANK YOU. An academic advisor wants a program that will compute a student
THIS IS A LONG QUESTION. PLEASE READ EVERYTHING IN FULL BEFORE ANSWERING. THANK YOU. An academic advisor wants a program that will compute a student GPA by reading a file that contains the name of the courses taken, credit hours for each course, and grades earned from each course. The program will take, as run-time parameters, the folder directory where the grades file is stored and the first and last name of the student. For this testing your program, use the posted grades file called grade/Irving-Whitewood.cvs. HERE IS WHAT IS IN GRADE/IRVING-WHITEWOOD.CVS:
To complete this assignment, we will use class Student.java completed from Chapter 10. The purpose for this is to reuse functionality we have already created. HERE IS STUDENT.JAVA:
import java.awt.print.Printable; import java.util.Arrays;
public class Student {
private String fname; private String lname; protected static int numCourses; Course[] cArry;
public Student() { }
//Constructors Student(String firstName, String lastName, int numCourses) { this.fname = firstName; this.lname = lastName; setNumCourses(Student.numCourses + numCourses); cArry = new Course[numCourses]; }
public void createCourse(String courseName, int creditHours, char letterGrade) { //create a course and add it to cArry Course createCourse = new Course(); createCourse.setCourseName(courseName); createCourse.setCreditHours(creditHours); createCourse.setLetterGrade(letterGrade); this.cArry[cArry.length - 1] = createCourse; }
/** * @return the fname */ public String getFname() { return fname; }
/** * @param fname the fname to set */ public void setFname(String fname) { this.fname = fname; }
/** * @return the lname */ public String getLname() { return lname; }
/** * @param lname the lname to set */ public void setLname(String lname) { this.lname = lname; }
/** * @return the cArry */ public Course[] getcArry() { return cArry; }
/** * @param cArry the cArry to set */ public void setcArry(Course[] cArry) { this.cArry = cArry; }
/** * @return the numCourses */ public int getNumCourses() { return numCourses; }
/** * @param numCourses the numCourses to set */ public void setNumCourses(int numCourses) { this.numCourses = numCourses; }
public String toString() { return "Student:\t" + fname + " " + lname; } } HERE IS COURSE:
// Class Course, revised for assignment 4.
public class Course
{
// Instance members
private String courseName;
private int creditHours;
private char letterGrade;
//Constructors
public Course()
{}
public Course(String cName, int cHours, char lGrade)
{
courseName = cName;
creditHours = cHours;
letterGrade = lGrade;
}
// Setter for courseName
public void setCourseName(String courseName)
{
courseName = courseName;
}
// Setter for letterGrade
public void setLetterGrade(char letterGrade)
{
letterGrade = letterGrade;
}
// Setter for creditHours
public void setCreditHours(int creditHours)
{
creditHours = creditHours;
}
// Getter for courseName
public String getCourseName()
{
return courseName;
}
// Getter for letterGrade
public char getLetterGrade()
{
return letterGrade;
}
// Getter for creditHours
public int getCreditHours()
{
return creditHours;
}
// Method to compute GPA for courses
public static double computeGPA(Course[] clist)
{
double gpa = 0.0;
int gradePoints = 0;
int totalCredits = 0;
for(Course c: clist)
{
int numCredits = 4;
gradePoints += c.getGradePoint() * numCredits;
totalCredits += numCredits;
}
gpa = ((double) gradePoints)/totalCredits;
return gpa;
}
// Method to convert letterGrade to a grade point numerical value
private int getGradePoint()
{
int gp = 0;
switch(getLetterGrade())
{
case 'A':
gp = 4;
break;
case 'B':
gp = 3;
break;
case 'C':
gp = 2;
break;
case 'D':
gp = 1;
break;
default:
gp = 0;
}
return gp;
}
// A way to testthe class
public static void main(String[] args)
{
Course c1 = new Course();
c1.setCreditHours(3);
c1.setLetterGrade('A');
Course c2 = new Course();
c2.setCreditHours(3);
c2.setLetterGrade('C');
Course[] clist = new Course[2];
clist[0] = c1;
clist[1] = c2;
System.out.println("GPA=" + Course.computeGPA(clist));
}
}
---
HERE IS MyStudent
/*
* Class: CS 2302/02
* Term: Summer 2015
* Name: Kevin Morton
* Professor: Dr. Hisham Haddad
* Homework: Assignment 3
*/
import java.util.ArrayList;
public class MyStudent extends Student {
/** Static arrayList of courses */
private static ArrayList
/** no-arg constructor */
public MyStudent() {
super();
}
/** MyStudent constructor */
public MyStudent(String fname, String lname) {
//Determine the number of courses from the size of the courseList.
super(fname, lname, numCourses);
//get the # of courses
int coursesCount = super.getNumCourses();
System.out.println("Number of courses " + coursesCount);
}
/** Public static method to add courses to the arrayList */
public static void addCourse(String courseName, int creditHours, char letterGrade) {
//creates a Course object and add it to the courseList member.
Course newCourse = new Course();
newCourse.setCourseName(courseName);
newCourse.setCreditHours(creditHours);
newCourse.setLetterGrade(letterGrade);
//add the new course to the courselist arrayList
courseList.add(newCourse);
}
/**
* @return the courseList
*/
private static ArrayList
return courseList;
}
/**
* @param courseList the courseList to set
*/
private static void setCourseList(ArrayList
MyStudent.courseList = courseList;
}
/**
* Get the students GPA based on all courses available in the courseList array
*
* @param clist Arraylist
* @return double value equal to students GPA
*/
public static double getComputedGPA(ArrayList
Course[] aCourse = new Course[clist.size()];
aCourse = clist.toArray(aCourse);
Double gpa = 0.0;
gpa = Course.computeGPA(aCourse);
return gpa;
}
@Override
public String toString() {
return super.toString() + " has a " + getComputedGPA(courseList) + " GPA";
}
}
----
Inside your ComputeStudentGPAProgram.java, write a main method that serves as the main launching code. This program will read the file (grade/Irving-Whitewood.cvs) that contains the grades for a student and the first and last name of the student. The program determines the GPA for the student.
Here, you will read the csv file (grade/Irving-Whitewood.cvs) to determine the students GPA. For each line in the file, you will need store this information by calling method addCourse() from class MyStudent. Once you have completed reading the file, close the file, and then proceed to do the following:
** invoke the toString() method of this object inside a System.out.println statement
The output of your code will be:
Output: Student {firstname}, {lastname} has a {GPA value} GPA
Example: John Doe has a 3.4 GPA
NOTE
You will be provided with a file grade/Irving-Whitewood.cvs. Note that you cannot hardcode the first name and last name into your program directly. Your program MUST read the filename during the code execution.
The structure of file Irving-Whitewood.cvs is:
CourseName1,creditHours,grade
CourseName2,creditHours,grade
CourseName3,creditHours,grade
CourseName4,creditHours,grade
CourseName5,creditHours,grade
Example:
Calculus,4,A
Physics,4,A
Computer Science,4,B
English,3,C
AmericanHistory,2,B
Inside your ComputeStudentGPAProgram, you will need to use a mechanism to parse each line. While there are a variety ways to accomplish this, the String.split() method is the most feasible.
1 Calculus 2 Physics 4A 4A 3 Computer Science 4B 3C AmericanHistory 2B 4 EnglishStep by Step Solution
There are 3 Steps involved in it
Step: 1
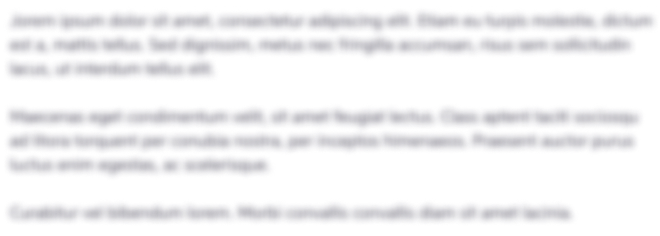
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started