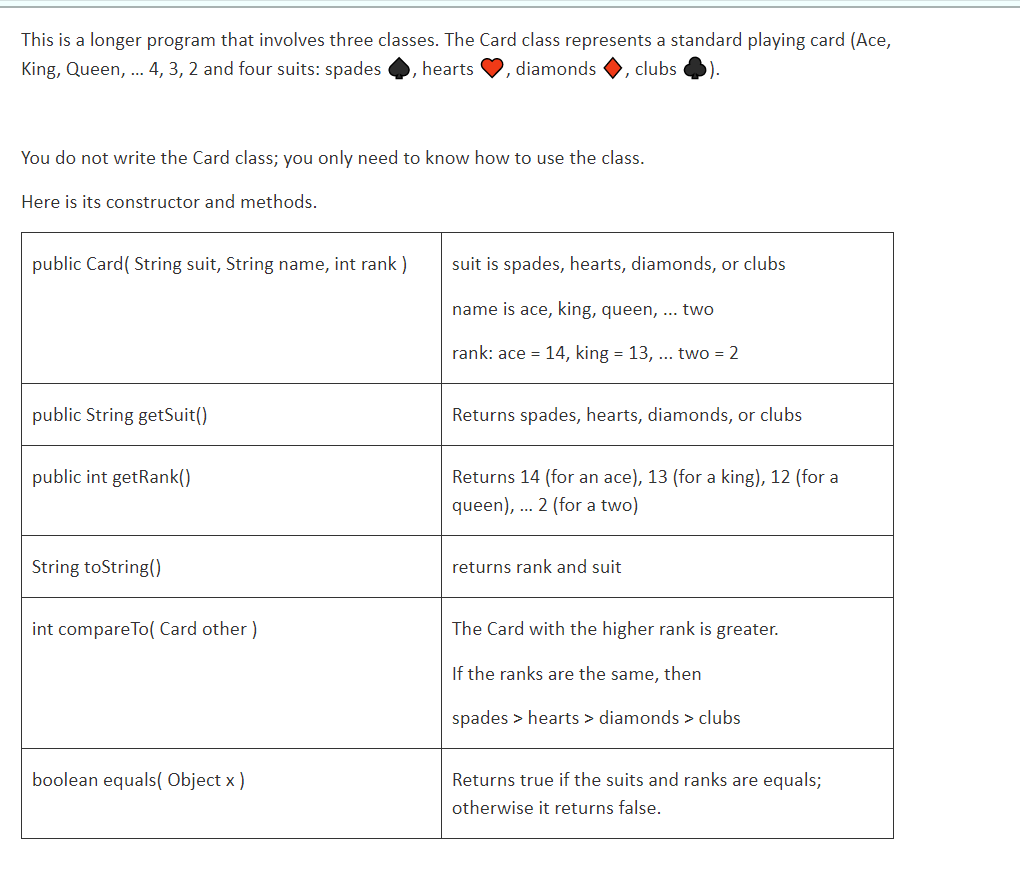
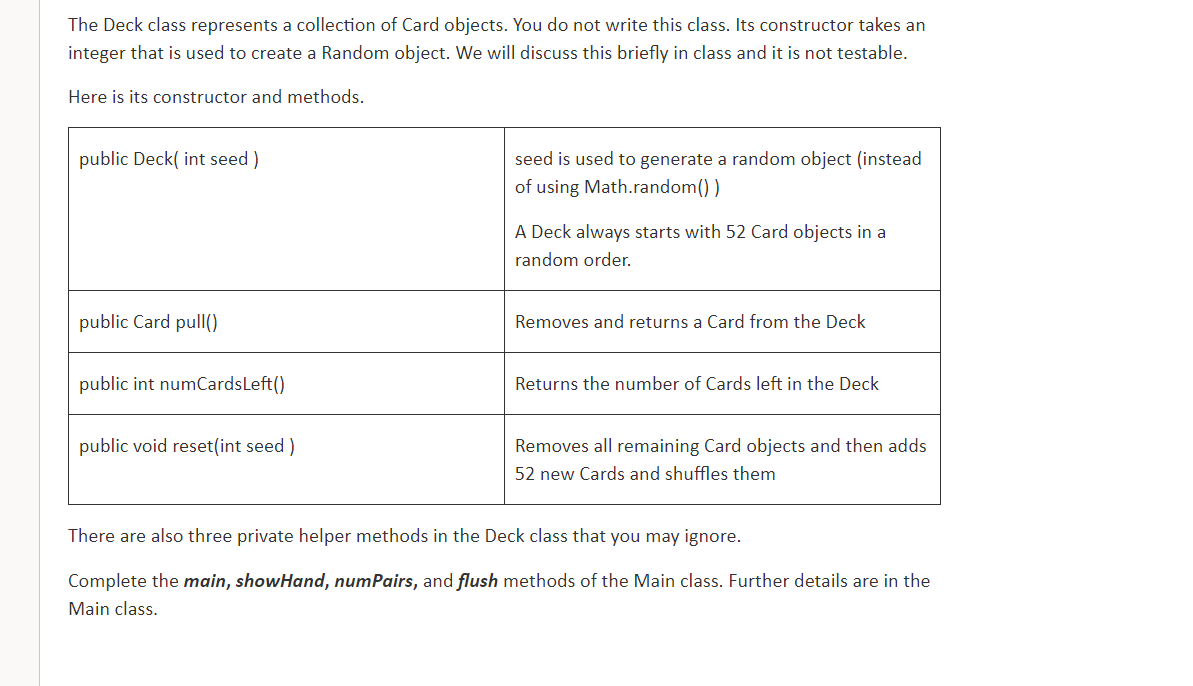
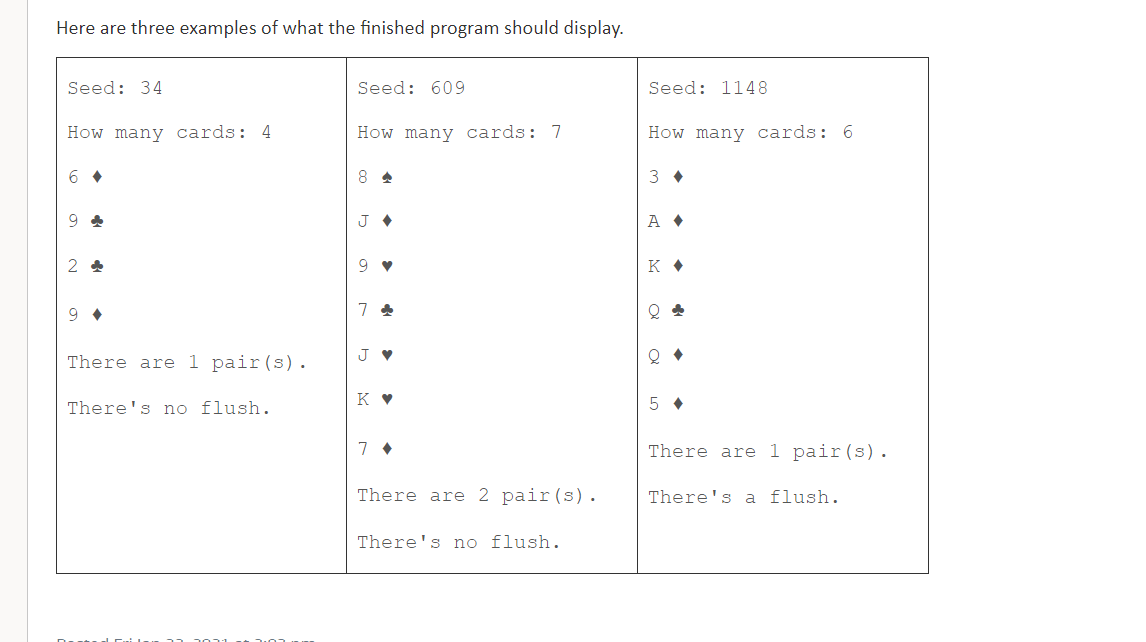
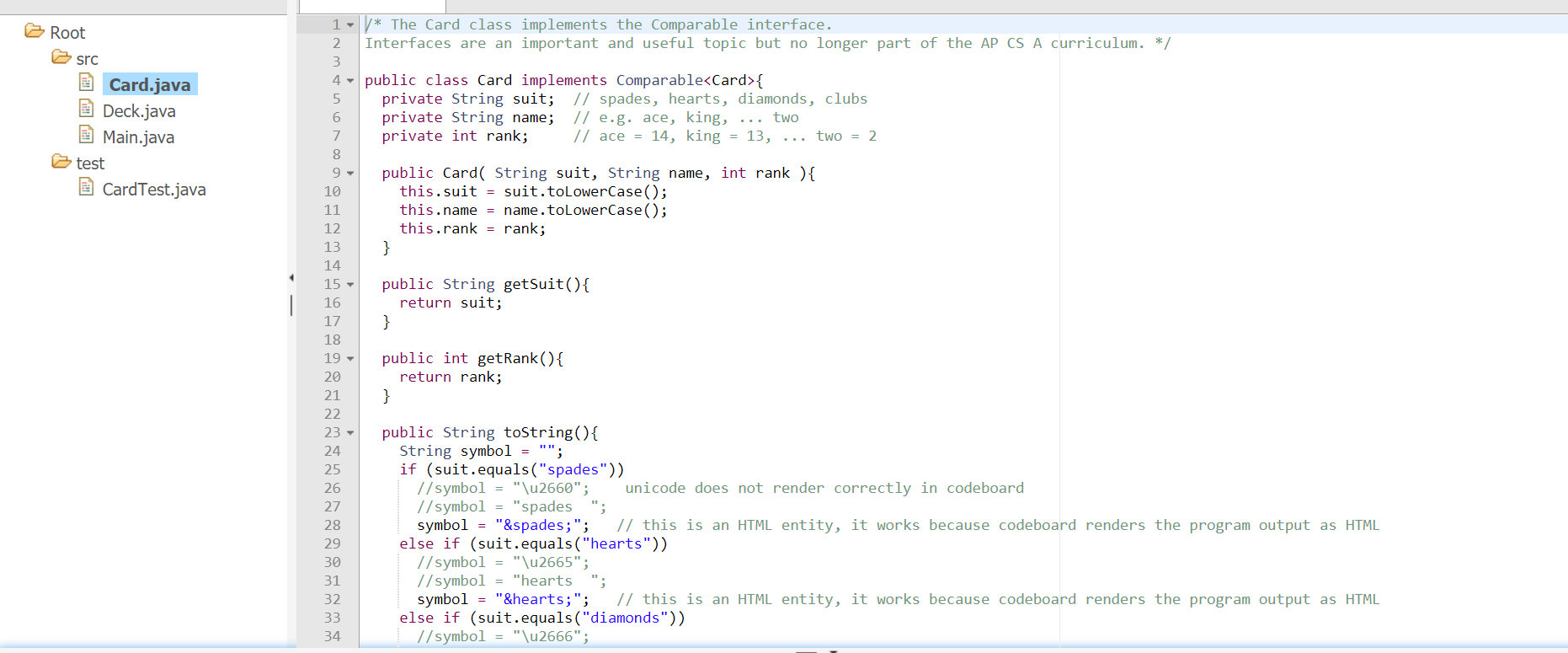
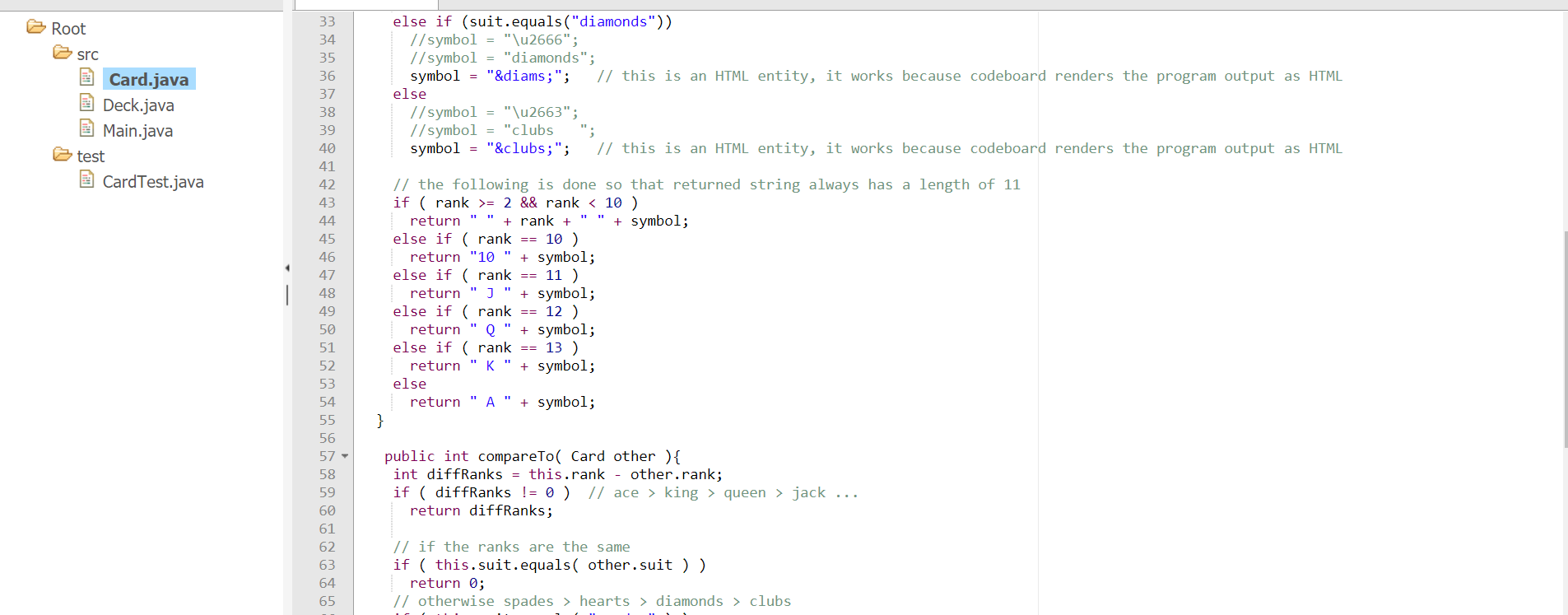
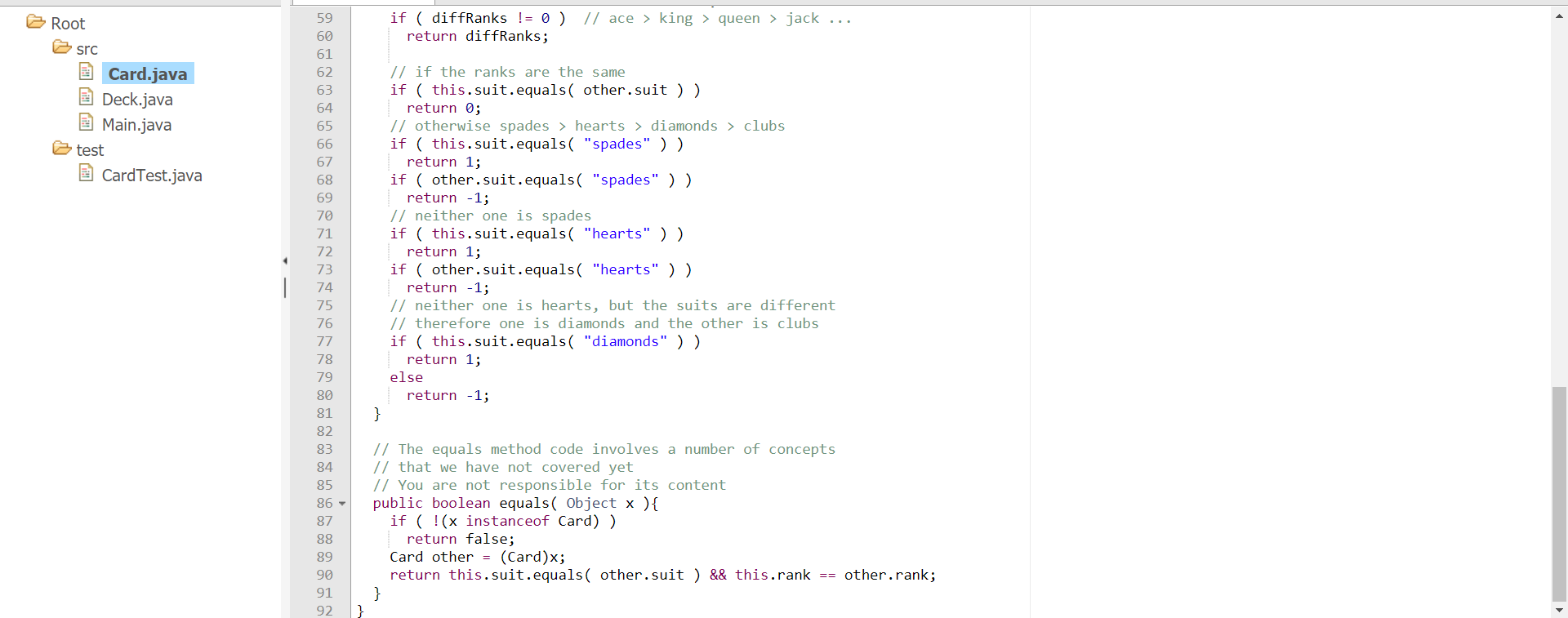
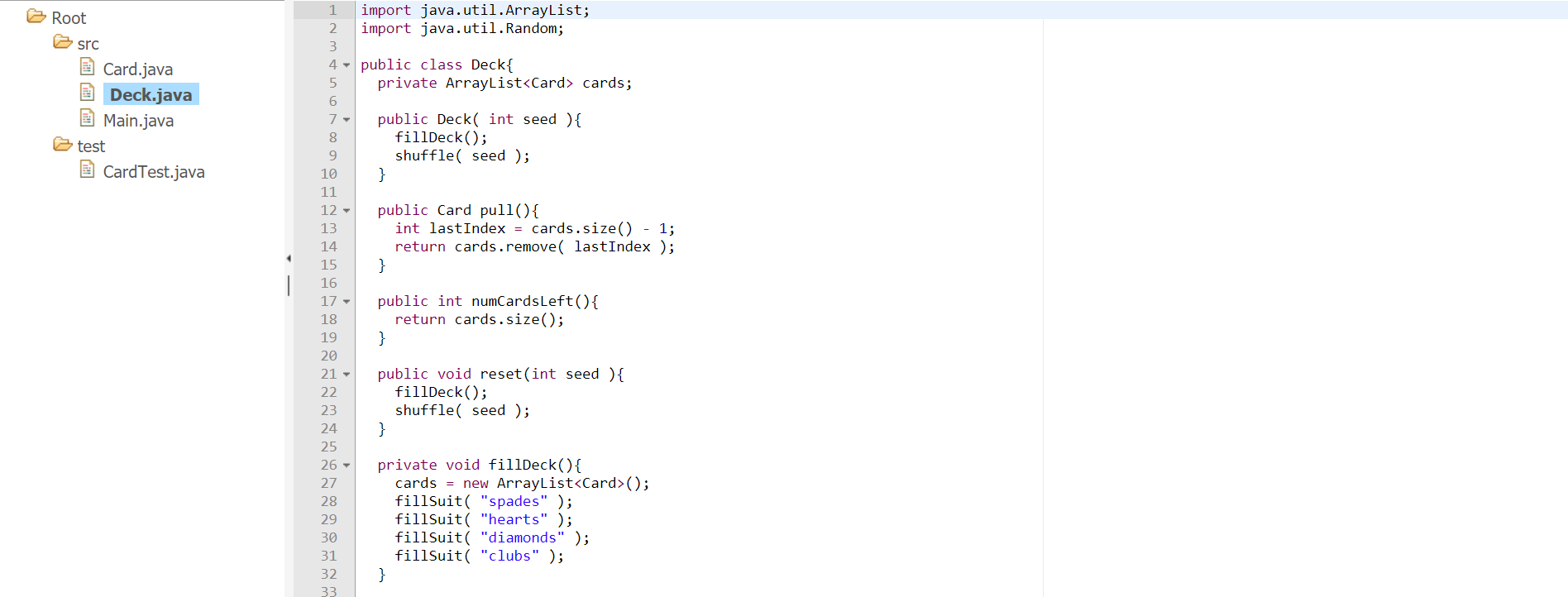
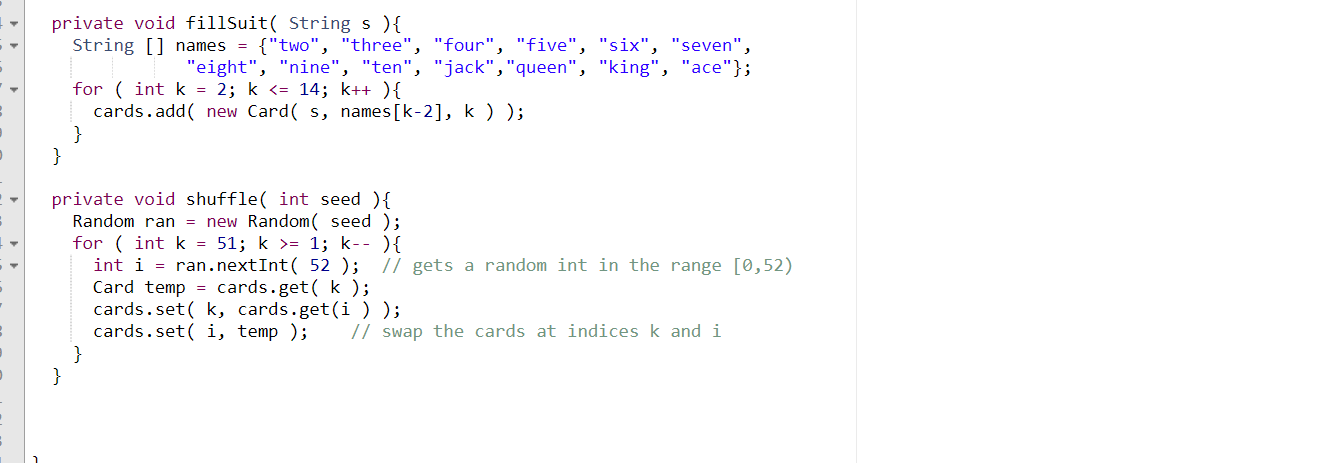
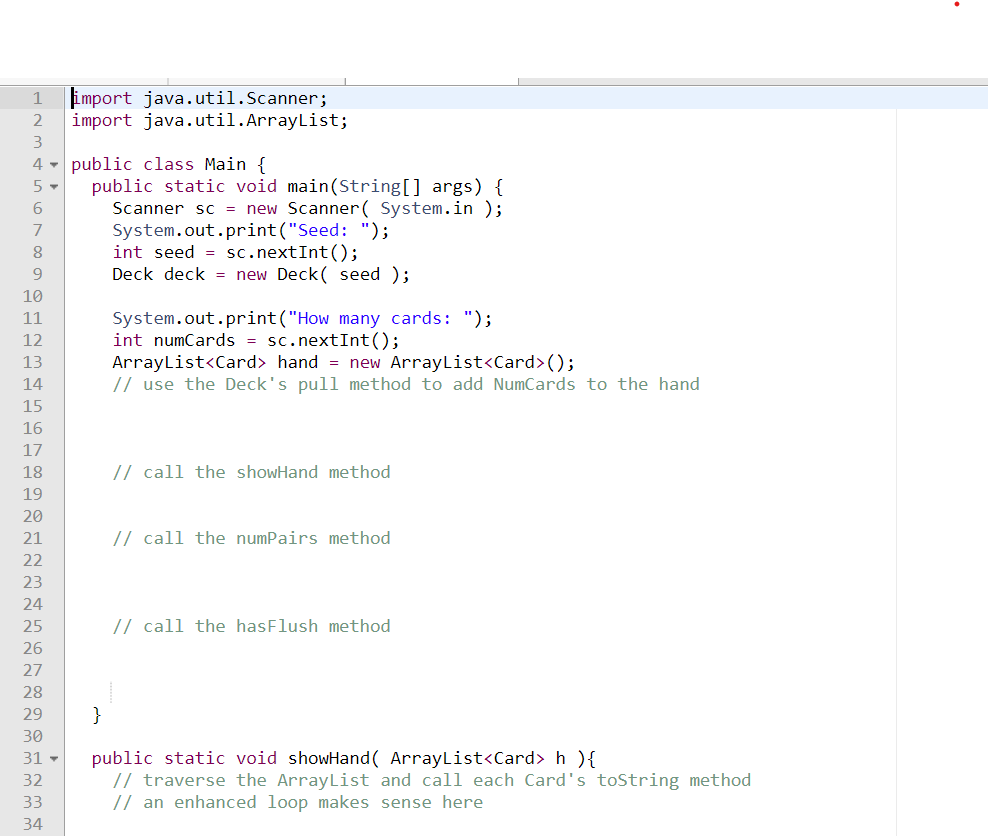
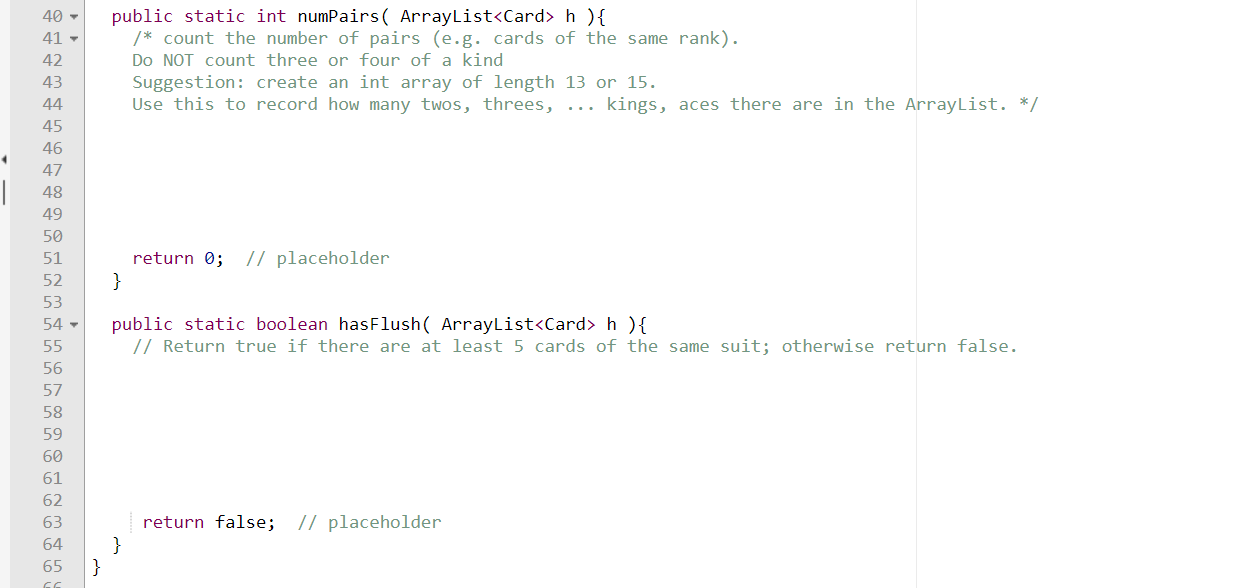
This is a longer program that involves three classes. The Card class represents a standard playing card (Ace, King, Queen, ... 4, 3, 2 and four suits: spades hearts diamonds clubs ). You do not write the Card class; you only need to know how to use the class. Here is its constructor and methods. public Card( String suit, String name, int rank) suit is spades, hearts, diamonds, or clubs name is ace, king, queen, ... two rank: ace = 14, king = 13, ... two = 2 public String getSuit() Returns spades, hearts, diamonds, or clubs public int getRank() Returns 14 (for an ace), 13 (for a king), 12 (for a queen), ... 2 (for a two) String toString() returns rank and suit int compare Tol Card other) The Card with the higher rank is greater. If the ranks are the same, then spades > hearts > diamonds > clubs boolean equals( Object x) Returns true if the suits and ranks are equals; otherwise it returns false. The Deck class represents a collection of Card objects. You do not write this class. Its constructor takes an integer that is used to create a Random object. We will discuss this briefly in class and it is not testable. Here is its constructor and methods. public Deck( int seed) seed is used to generate a random object (instead of using Math.random()) A Deck always starts with 52 Card objects in a random order. public Card pull() Removes and returns a Card from the Deck public int numCardsLeft() Returns the number of Cards left in the Deck public void reset(int seed) Removes all remaining Card objects and then adds 52 new Cards and shuffles them There are also three private helper methods in the Deck class that you may ignore. Complete the main, showHand, num Pairs, and flush methods of the Main class. Further details are in the Main class. Here are three examples of what the finished program should display. Seed: 34 Seed: 609 Seed: 1148 How many cards: 4 How many cards: 7 How many cards: 6 3 A K. 74 There are 1 pair(s). There's no flush. 5 7 There are 1 pair(s). There are 2 pair(s). There's a flush. There's no flush. Root src Card.java Deck.java Main.java test CardTest.java 1- /* The Card class implements the Comparable interface. 2 Interfaces are an important and useful topic but no longer part of the AP CS A curriculum. */ 3 4- public class Card implements Comparable
{ 5 private String suit; // spades, hearts, diamonds, clubs 6 private String name; // e.g. ace, king, ... two 7 private int rank; // ace = 14, king = 13, ... two = 2 8 9- public Card( String suit, String name, int rank ) { 10 this.suit = suit.toLowerCase(); 11 this.name = name.toLowerCase(); 12 this.rank = rank; 13 } 14 15 - public String getSuit(){ 16 return suit; 17 } 18 19 - public int getRank() { 20 return rank; 21 } 22. 23 - public String toString(){ 24 String symbol = ""; 25 if (suit.equals("spades")) 26 //symbol = "\u2660"; unicode does not render correctly in codeboard 27 //symbol = "spades "; 28 symbol = "♠"; // this is an HTML entity, it works because codeboard renders the program output as HTML 29 else if (suit.equals("hearts")) 30 //symbol = "\u2665"; 31 //symbol = "hearts 32 symbol = "♥"; // this is an HTML entity, it works because codeboard renders the program output as HTML 33 else if (suit.equals("diamonds")) 34 //symbol = "\u2666"; Root src Card.java Deck.java Main.java test CardTest.java else if (suit.equals("diamonds")) //symbol = "\u2666"; // symbol = "diamonds"; symbol = "♦"; // this is an HTML entity, it works because codeboard renders the program output as HTML else // symbol = "\u2663"; //symbol = "clubs "; symbol = "♣"; // this is an HTML entity, it works because codeboard renders the program output as HTML 4 | 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 - 58 59 60 61 62 63 64 65 // the following is done so that returned string always has a length of 11 if ( rank >= 2 && rank king > queen > jack ... return diffRanks; // if the ranks are the same if ( this.suit.equals( other.suit ) ) return 0; // otherwise spades > hearts > diamonds > clubs Root if ( diffRanks != 0 ) // ace > king > queen > jack .. return diffRanks; src Card.java Deck.java Main.java test CardTest.java 59 60 61 62. 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 - 87 88 89 90 91 92 // if the ranks are the same if ( this.suit.equals( other.suit )) return 0; // otherwise spades > hearts > diamonds > clubs if ( this.suit.equals( "spades" ) ) return 1; if ( other.suit.equals( "spades" ) ) return -1; // neither one is spades if ( this.suit.equals("hearts" ) ) return 1; if ( other suit.equals("hearts" ) ) return -1; // neither one is hearts, but the suits are different // therefore one is diamonds and the other is clubs if ( this.suit.equals( "diamonds" )) return 1; else return -1; } // The equals method code involves a number of concepts // that we have not covered yet // You are not responsible for its content public boolean equals( Object x ) { if ( !(x instanceof Card) ) return false; Card other = (Card)x; return this.suit.equals( other.suit ) && this.rank } == other.rank; Root src Card.java Deck.java Main.java test CardTest.java 1 import java.util.ArrayList; 2 import java.util. Random; 3 4- public class Deck{ 5 private ArrayList cards; 6 7- public Deck( int seed ) { 8 fillDeck(); 9 shuffle( seed ); 10 } 11 12 - public Card pull() { 13 int lastIndex = cards.size() - 1; 14 return cards.remove( lastIndex ); 15 } 16 17 - public int numCardsLeft(){ 18 return cards.size(); 19 } 20 21 - public void reset(int seed ) { 22 fillDeck(); 23 shuffle( seed ); 24 } 25 26 - private void fillDeck(){ 27 cards = new ArrayList(); 28 fillSuit( "spades" ); 29 fillSuit( "hearts" ); 30 fillSuit( "diamonds" ); 31 fillSuit( "clubs" ); 32 } 33 private void fillSuit( String s ){ String [] names = {"two", "three", "four", "five", "six", "seven", "eight", "nine", "ten", "jack", "queen", "king", "ace"}; for ( int k = 2; k = 1; k--){ int i = ran.nextInt( 52 ); // gets a random int in the range [0,52) Card temp = cards.get( k ); cards.set( k, cards.get(i ) ); cards.set( i, temp); // swap the cards at indices k and i } } 1 import java.util.Scanner; 2. import java.util.ArrayList; 3 4- public class Main { 5 public static void main(String[] args) { 6 Scanner sc = new Scanner(System.in); 7 System.out.print("Seed: "); 8 int seed = sc.nextInt(); 9 Deck deck = new Deck( seed ); 10 11 System.out.print("How many cards: "); 12 int numCards = sc.nextInt(); 13 ArrayList hand = new ArrayList(); 14 // use the Deck's pull method to add NumCards to the hand 15 16 17 18 // call the showHand method 19 20 21 // call the numPairs method 22 23 24 25 // call the has Flush method 26 27 28 29 } 30 31 - public static void showHand( ArrayList h ) { 32 // traverse the ArrayList and call each Card's toString method 33 // an enhanced loop makes sense here 34 public static int numPairs( ArrayList h ) { /* count the number of pairs (e.g. cards of the same rank). Do NOT count three or four of a kind Suggestion: create an int array of length 13 or 15. Use this to record how many twos, threes, kings, aces there are in the ArrayList. */ 4 40 41 - 42 43 44 45 46 47 48 49 50 51 52 53 54 - 55 56 57 58 59 60 return 0; // placeholder } public static boolean hasFlush( ArrayList h ){ // Return true if there are at least 5 cards of the same suit; otherwise return false. 61 62 63 64 return false; // placeholder } 65 } This is a longer program that involves three classes. The Card class represents a standard playing card (Ace, King, Queen, ... 4, 3, 2 and four suits: spades hearts diamonds clubs ). You do not write the Card class; you only need to know how to use the class. Here is its constructor and methods. public Card( String suit, String name, int rank) suit is spades, hearts, diamonds, or clubs name is ace, king, queen, ... two rank: ace = 14, king = 13, ... two = 2 public String getSuit() Returns spades, hearts, diamonds, or clubs public int getRank() Returns 14 (for an ace), 13 (for a king), 12 (for a queen), ... 2 (for a two) String toString() returns rank and suit int compare Tol Card other) The Card with the higher rank is greater. If the ranks are the same, then spades > hearts > diamonds > clubs boolean equals( Object x) Returns true if the suits and ranks are equals; otherwise it returns false. The Deck class represents a collection of Card objects. You do not write this class. Its constructor takes an integer that is used to create a Random object. We will discuss this briefly in class and it is not testable. Here is its constructor and methods. public Deck( int seed) seed is used to generate a random object (instead of using Math.random()) A Deck always starts with 52 Card objects in a random order. public Card pull() Removes and returns a Card from the Deck public int numCardsLeft() Returns the number of Cards left in the Deck public void reset(int seed) Removes all remaining Card objects and then adds 52 new Cards and shuffles them There are also three private helper methods in the Deck class that you may ignore. Complete the main, showHand, num Pairs, and flush methods of the Main class. Further details are in the Main class. Here are three examples of what the finished program should display. Seed: 34 Seed: 609 Seed: 1148 How many cards: 4 How many cards: 7 How many cards: 6 3 A K. 74 There are 1 pair(s). There's no flush. 5 7 There are 1 pair(s). There are 2 pair(s). There's a flush. There's no flush. Root src Card.java Deck.java Main.java test CardTest.java 1- /* The Card class implements the Comparable interface. 2 Interfaces are an important and useful topic but no longer part of the AP CS A curriculum. */ 3 4- public class Card implements Comparable{ 5 private String suit; // spades, hearts, diamonds, clubs 6 private String name; // e.g. ace, king, ... two 7 private int rank; // ace = 14, king = 13, ... two = 2 8 9- public Card( String suit, String name, int rank ) { 10 this.suit = suit.toLowerCase(); 11 this.name = name.toLowerCase(); 12 this.rank = rank; 13 } 14 15 - public String getSuit(){ 16 return suit; 17 } 18 19 - public int getRank() { 20 return rank; 21 } 22. 23 - public String toString(){ 24 String symbol = ""; 25 if (suit.equals("spades")) 26 //symbol = "\u2660"; unicode does not render correctly in codeboard 27 //symbol = "spades "; 28 symbol = "♠"; // this is an HTML entity, it works because codeboard renders the program output as HTML 29 else if (suit.equals("hearts")) 30 //symbol = "\u2665"; 31 //symbol = "hearts 32 symbol = "♥"; // this is an HTML entity, it works because codeboard renders the program output as HTML 33 else if (suit.equals("diamonds")) 34 //symbol = "\u2666"; Root src Card.java Deck.java Main.java test CardTest.java else if (suit.equals("diamonds")) //symbol = "\u2666"; // symbol = "diamonds"; symbol = "♦"; // this is an HTML entity, it works because codeboard renders the program output as HTML else // symbol = "\u2663"; //symbol = "clubs "; symbol = "♣"; // this is an HTML entity, it works because codeboard renders the program output as HTML 4 | 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 - 58 59 60 61 62 63 64 65 // the following is done so that returned string always has a length of 11 if ( rank >= 2 && rank king > queen > jack ... return diffRanks; // if the ranks are the same if ( this.suit.equals( other.suit ) ) return 0; // otherwise spades > hearts > diamonds > clubs Root if ( diffRanks != 0 ) // ace > king > queen > jack .. return diffRanks; src Card.java Deck.java Main.java test CardTest.java 59 60 61 62. 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 - 87 88 89 90 91 92 // if the ranks are the same if ( this.suit.equals( other.suit )) return 0; // otherwise spades > hearts > diamonds > clubs if ( this.suit.equals( "spades" ) ) return 1; if ( other.suit.equals( "spades" ) ) return -1; // neither one is spades if ( this.suit.equals("hearts" ) ) return 1; if ( other suit.equals("hearts" ) ) return -1; // neither one is hearts, but the suits are different // therefore one is diamonds and the other is clubs if ( this.suit.equals( "diamonds" )) return 1; else return -1; } // The equals method code involves a number of concepts // that we have not covered yet // You are not responsible for its content public boolean equals( Object x ) { if ( !(x instanceof Card) ) return false; Card other = (Card)x; return this.suit.equals( other.suit ) && this.rank } == other.rank; Root src Card.java Deck.java Main.java test CardTest.java 1 import java.util.ArrayList; 2 import java.util. Random; 3 4- public class Deck{ 5 private ArrayList cards; 6 7- public Deck( int seed ) { 8 fillDeck(); 9 shuffle( seed ); 10 } 11 12 - public Card pull() { 13 int lastIndex = cards.size() - 1; 14 return cards.remove( lastIndex ); 15 } 16 17 - public int numCardsLeft(){ 18 return cards.size(); 19 } 20 21 - public void reset(int seed ) { 22 fillDeck(); 23 shuffle( seed ); 24 } 25 26 - private void fillDeck(){ 27 cards = new ArrayList(); 28 fillSuit( "spades" ); 29 fillSuit( "hearts" ); 30 fillSuit( "diamonds" ); 31 fillSuit( "clubs" ); 32 } 33 private void fillSuit( String s ){ String [] names = {"two", "three", "four", "five", "six", "seven", "eight", "nine", "ten", "jack", "queen", "king", "ace"}; for ( int k = 2; k = 1; k--){ int i = ran.nextInt( 52 ); // gets a random int in the range [0,52) Card temp = cards.get( k ); cards.set( k, cards.get(i ) ); cards.set( i, temp); // swap the cards at indices k and i } } 1 import java.util.Scanner; 2. import java.util.ArrayList; 3 4- public class Main { 5 public static void main(String[] args) { 6 Scanner sc = new Scanner(System.in); 7 System.out.print("Seed: "); 8 int seed = sc.nextInt(); 9 Deck deck = new Deck( seed ); 10 11 System.out.print("How many cards: "); 12 int numCards = sc.nextInt(); 13 ArrayList hand = new ArrayList(); 14 // use the Deck's pull method to add NumCards to the hand 15 16 17 18 // call the showHand method 19 20 21 // call the numPairs method 22 23 24 25 // call the has Flush method 26 27 28 29 } 30 31 - public static void showHand( ArrayList h ) { 32 // traverse the ArrayList and call each Card's toString method 33 // an enhanced loop makes sense here 34 public static int numPairs( ArrayList h ) { /* count the number of pairs (e.g. cards of the same rank). Do NOT count three or four of a kind Suggestion: create an int array of length 13 or 15. Use this to record how many twos, threes, kings, aces there are in the ArrayList. */ 4 40 41 - 42 43 44 45 46 47 48 49 50 51 52 53 54 - 55 56 57 58 59 60 return 0; // placeholder } public static boolean hasFlush( ArrayList h ){ // Return true if there are at least 5 cards of the same suit; otherwise return false. 61 62 63 64 return false; // placeholder } 65 }