This is an intro class, please write the code in an easy to understand way(if you are able to of course)
This is the starter code
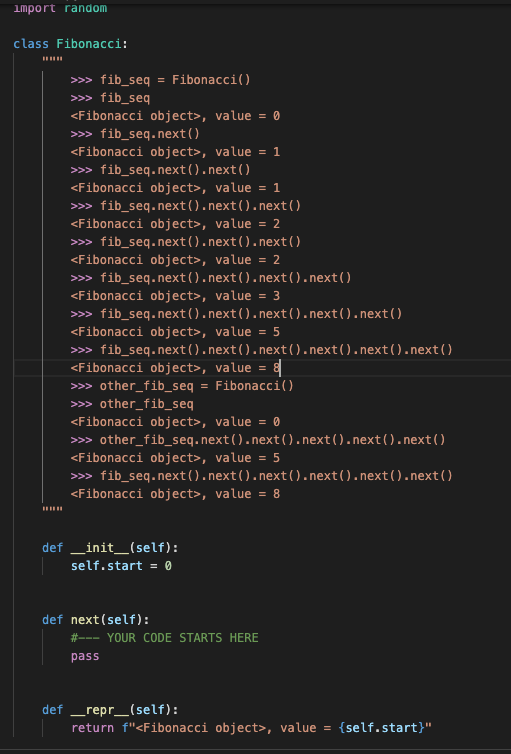
import random
class Fibonacci:
"""
>>> fib_seq = Fibonacci()
>>> fib_seq
, value = 0
>>> fib_seq.next()
, value = 1
>>> fib_seq.next().next()
, value = 1
>>> fib_seq.next().next().next()
, value = 2
>>> fib_seq.next().next().next()
, value = 2
>>> fib_seq.next().next().next().next()
, value = 3
>>> fib_seq.next().next().next().next().next()
, value = 5
>>> fib_seq.next().next().next().next().next().next()
, value = 8
>>> other_fib_seq = Fibonacci()
>>> other_fib_seq
, value = 0
>>> other_fib_seq.next().next().next().next().next()
, value = 5
>>> fib_seq.next().next().next().next().next().next()
, value = 8
"""
def __init__(self):
self.start = 0
def next(self):
#--- YOUR CODE STARTS HERE
pass
def __repr__(self):
return f", value = {self.start}"
Section 1: The Fibonacci class (2 pts) The Fibonacci sequence is the series of numbers where each number is the sum of the two preceding numbers. For example: 0,1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, ... Mathematically we can describe this as X = Xn-1 + xn-2 The Fibonacci class has a value attribute that represents a number in the Fibonacci sequence. Write the code to implement the next method that returns an instance of the Fibonacci class whose value is the next Fibonacci number. You are not allowed to modify the constructor and the _repr_ methods. Methods Return Type Name Fibonnaci next(self) Description Next number in the Fibonacci sequence Legible representation for Fibonacci objects. Already implemented for you in the starter code str repr_(self) next(self) Returns a Fibonaci instance whose value is the next Fibonacci number. Method should not compute all previous Fibonnaci numbers each time is called in other words, no loops or recursion required). Instead, keep track of the previous number by setting a new instance attribute inside this method. Remember from this week's lectures that we can create instance attributes for objects at any point, even outside the constructor. Output Fibonnaci Next Fibonacci number. Note that Fibonacci objects are displayed based on the return value of the repr_method in the Fibonacci class. Examples: >>> fib_seq = Fibonacci() >>> fib_seq
, value = 0 >>> fib_seq.next() , value = 1 >>> fib_seq.next().next() , value = 1 >>> fib_seq.next().next().next() , value = 2 >>> fib_seq.next().next().next() , value = 2 import random class Fibonacci: HE >>> fib_seq = Fibonacci() >>> fib_seq , value = 0 >>> fib_seq.next() , value = 1 >>> fib_seq.next().next() , value = 1 >>> fib_seq.next().next().next() , value = 2 >>> fib_seq.next().next().next() , value = 2 >>> fib_seq.next().next().next().next() , value = 3 >>> fib_seq.next().next().next().next().next() , value = 5 >>> fib_seq.next().next().next().next().next().next() , value = >>> other_fib_seq = Fibonacci() >>> other_fib_seq , value = 0 >>> other_fib_seq.next().next().next().next().next() , value = 5 >>> fib_seq.next().next().next() .next().next().next() , value = 8 def __init__(self): self.start = 0 def next(self): #--- YOUR CODE STARTS HERE pass def _repr_(self): return f", value = {self.start}