Question
This is C++... This is an exercise to try out the textbooks UnsortedType class with the integer ItemType, from chapter 3. The code is taken
This is C++...
This is an exercise to try out the textbooks UnsortedType class with the integer ItemType, from chapter 3. The code is taken from the textbook website: http://www.jblearning.com/catalog/9781284089189/ -- click on the Sample Materials tab and then the Source Code link to get the code (which is in the Chapter 3 subfolder of the PlusCode6 subfolder. More generally, it will give you practice working with abstract data types. We will thus avoid modifying the classes from the text. The fact that it will be quite awkward to do so will show you that the code is not great.
We will modify a class for a list of test scores so it uses the abstract data type code to get the job done. The test scores code is based on an example from the Gaddis text that many of you used for CS 1160/2360/2370. The code was written by Adam Allard and can be found on one of his github repositories at: https://github.com/Amallard/cplusplus3/tree/master/test-scores
Make sure your code builds and runs. Use an IDE to do your work.
The assignment:
Modify the TestScores class as follows:
Instead of storing an array of scores, use an UnsortedType
int numScores() return the number of scores
void addScore(int score) add a new score.
o This is where you throw the NegativeScore or TooLargeScore exceptions if necessary.
o Also, throw a TooManyScores exception if the UnsortedType is already full (define TooManyScores along with NegativeScore and TooLargeScore)
double getAverage() return the average of all scoresModify Main.cpp to use the modified TestScores class. Throw a NoScores exception if there are no scores to average. Add the NoScoresdefinition too.
Modify Main.cpp:
Use the modified TestScores class
Check for the NoScores and TooManyScores exceptions put cases to try to get the average of the week 1 scores before they have been added and to add more than 5 scores (the maximum size of an UnsortedType)
Details and comments (long, but I think you need to go through these to work around the limitations of the code):
For TestScores:
no credit if you use use both an array and an UnsortedType, or keep track of the sum or average in a separate field
addScore() -- This is where things start to get a little awkward. For at least Visual C++, you need to construct an ItemType object and set the value so you have something of the right type to put in the UnsortedType
getAverage() -- You need to use the iterator functions of the UnsortedType, ResetList() and GetNextItem() to retrieve the values. This is where things get awful. ItemType does not have a way to extract the value, so you need to take the value returned by GetNextItem() and:
o take its address (the & operator) so you have the address of an ItemType
o cast that to a pointer to an int (the ItemTypes only field is actually an int)
o and finally dereference the pointer (use *) to get the int value
o [We get the value we want without modifying ItemType, and it is clear the ItemType is an int, but this should feel wrong. We are breaking the encapsulation of the private field. We will only do this for this assignment.]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
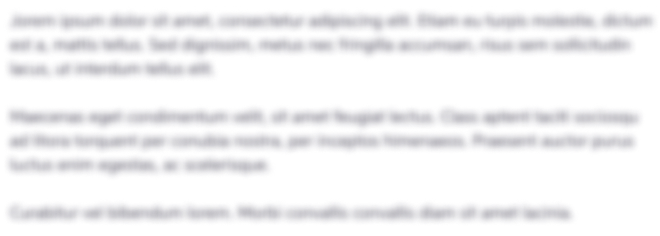
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started