Question
/*THIS IS COMPLETED CLASS * CSC115 Assignment 6 : Emergency Room * ER_Patient.java * Created for use by CSC115 Summer 2018. */ import java.time.LocalTime; import
/*THIS IS COMPLETED CLASS
* CSC115 Assignment 6 : Emergency Room
* ER_Patient.java
* Created for use by CSC115 Summer 2018.
*/
import java.time.LocalTime;
import java.util.Random;
/**
* ER_Patient is a class that represents a patient who signs into the Emergency Room
* to be assessed and treated by an ER Physician.
* Each patient has :
*
- an Id number, either accepted or generated,
- a time of admission, either accepted or generated,
- and a specific symptomCategory that determines the priority number [1,4], inclusive,
* calculated within the program.
*
*
*
*
*/
public class ER_Patient implements Comparable
/* Static variables necessary to randomize Id numbers */
private static final int numDigits = 2;
private static int range = (int)(Math.pow(10,numDigits));
private static boolean[] used = new boolean[range];
private static int[] fresh;
private static int currIndex = 0;
private static boolean open = false;
/* instance variables */
private String patientId;
private String symptomCategory;
private int priorityNum;
private LocalTime admitTime;
/**
* Create ER_Patient admission information.
* @param admitTime The time given in hh:mm:ss format.
* @param patientNumber A number given to the patient.
* If this number is unique, then it is applied to the Patient Id.
* If it is not unique, then a random unique Id is attributed to the
* patient.
* @param symptomCategory The category that determines the priority.
* Acceptable categories are:
- Life-threatening
- Chronic
- Major fracture
- Walk-in
*
*
*
*
*
*/
public ER_Patient(String admitTime, int patientNumber, String symptomCategory) {
if (!open) {
createUniqueIdList();
}
this.admitTime = LocalTime.parse(admitTime);
patientId = setUniqueId(patientNumber);
this.symptomCategory = symptomCategory;
priorityNum = determinePriority();
}
/**
* Create ER_patient admission information.
* A patient Id number is generated.
* @param admitTime The time given in hh:mm:ss format.
* @param symptomCategory The category that determines the priority.
* Acceptable categories are:
- Life-threatening
- Chronic
- Major fracture
- Walk-in
*
*
*
*
*
*/
public ER_Patient(String admitTime, String symptomCategory) {
if (!open) {
createUniqueIdList();
}
this.admitTime = LocalTime.parse(admitTime);
patientId = createUniqueId();
this.symptomCategory = symptomCategory;
priorityNum = determinePriority();
}
/**
* Create ER_patient dmission information.
* A patient Id number is generated.
* The admission time is the current time.
* @param symptomCategory The category that determines the priority.
* Acceptable categories are:
- Life-threatening
- Chronic
- Major fracture
- Walk-in
*
*
*
*
*
*/
public ER_Patient(String symptomCategory) {
if (!open) {
createUniqueIdList();
}
admitTime = LocalTime.now();
admitTime = admitTime.minusNanos(admitTime.getNano());
patientId = createUniqueId();
this.symptomCategory = symptomCategory;
priorityNum = determinePriority();
}
/*
* Given the symptom, assigns a priority number.
* Assumes that the
*/
private int determinePriority() {
switch(symptomCategory) {
case "Life-threatening":
return 1;
case "Chronic":
return 2;
case "Major fracture":
return 3;
case "Walk-in":
return 4;
default:
throw new NoSuchCategoryException("Category does not exist.");
}
}
/**
* @return The id number of the patient.
*/
public String getId() {
return patientId;
}
/**
* @return The priority number of the patient.
*/
public int getPriority() {
return priorityNum;
}
/**
* @return The patient's symptom category.
*/
public String getSymptomCategory() {
return symptomCategory;
}
/**
* Determines equality: If this patient has the same id, then
* equality is true.
* @param other The patient to compare to this one.
* @return True if the paitent id of this patient is the same
* as the other pateint's id.
*/
public boolean equals(ER_Patient other) {
return this.patientId.equals(other.patientId);
}
/**
* Determines whether this patient should be seen before the other patient.
* Order is determined first by priority number and secondarily by the admission
* time.
* @param other The patient to compare to this one.
* @return
- a number less than 0 if this patient has a higher priority,
- 0 if the priorities are equal (which should only happen if it is the same patient), or
- a number greater than 0 if the other patient has a higher priority.
*
*
*
*
* @throws ClassCastException if the parameter object is not of type ER_Patient.
*/
public int compareTo(ER_Patient other) {
int priorityDiff = this.priorityNum - other.priorityNum;
if (priorityDiff != 0) {
return priorityDiff;
} else {
return this.admitTime.compareTo(other.admitTime);
}
}
/**
* The string representation of a Patient.
* Format:
*
* patientId: priorityNum admission time symptomCategory
*
* @return Information about this patient as a String.
*/
public String toString() {
StringBuilder s = new StringBuilder(range+2+2+8+symptomCategory.length());
s.append(patientId+": "+priorityNum+" ");
s.append(admitTime+" ");
s.append(symptomCategory);
return s.toString();
}
/**
* A private method that shuffles an array of integers between 0 and range.
* Used to determine the unique values.
* This method is only called once for the first time a ER_Patient is created.
*/
private void createUniqueIdList() {
fresh = new int[range];
for (int i=0; i fresh[i] = i; } // Implement the Durstenfeld shuffle Random rand = new Random(); int r, tmp; for (int i=range-1; i>0; i--) { r = rand.nextInt(i+1); tmp = fresh[i]; fresh[i] = fresh[r]; fresh[r] = tmp; } open = true; } /** * A private method that sets the number as the patient id, after * checking that it has not been used before. * If it has, then a new number is generated instead. */ private String setUniqueId(int num) { if (num range-1) { return createUniqueId(); } if (used[num]) { return createUniqueId(); } return createId(num); } /** * A private method that creates a unique patient id number. */ private String createUniqueId() { while (currIndex currIndex++; } if (currIndex >= fresh.length) { throw new RuntimeException("Cannot create the new ER_Patient: No unique id numbers available."); } return createId(fresh[currIndex++]); } /** * Converts the checked number into a String starting with 'P'. */ private String createId(int num) { StringBuilder sb = new StringBuilder(numDigits+1); sb.append('P'); used[num] = true; int base = range/10; int currDigit; while (base >= 1) { currDigit = num/base; if (currDigit != 0) { num %= 10; } sb.append(currDigit); base /= 10; } return sb.toString(); } /** * The unit tester for this class. * @param args Not used. */ public static void main(String[] args) { ER_Patient[] patients = new ER_Patient[5]; String[] complaints = {"Walk-in", "Life-threatening","Chronic","Major fracture", "Chronic"}; for (int i=0; i patients[i] = new ER_Patient(complaints[i]); // spread out the admission times by 1 second try { Thread.sleep(1000); } catch (InterruptedException e) { System.out.println("sleep interrupted"); return; } } System.out.println("Patients waiting:"); for (int i=0; i System.out.println(patients[i]); } } } /* * CSC115 Assignment 5 : Emergency Room * NoSuchCategoryException.java * Created for use by CSC115 Summer 2018. */ /** * An runtime exception indicating that a particular category was not found * for a process to continue. * In this context, it is used to indicate that a * particular ER_Patient symptom was not recognized and an ER priority number * cannot be attributed to the patient. */ public class NoSuchCategoryException extends RuntimeException { /** THIS IS A COMPLETED CLASS * Create an exception. * @param msg The message associated with this exception. */ public NoSuchCategoryException(String msg) { super(msg); } /** * Create a default exception. */ public NoSuchCategoryException() { super(); } } THIS PART NEEDS TO BE COMPLETED *** HELP import java.util.NoSuchElementException; import java.util.Vector; import java.util.Comparator; /* * The shell of the class, to be completed as part * of CSC115 Assignment 5: Emergency Room. */ public class Heap private Vector private Comparator super E> comparator; public Heap() { heapArray = new Vector } public int size(){ return heapArray.size(); } public boolean heapIsEmpty(){ if (heapArray.size() == 0){ return true; } else return false; } public void heapInsert(E item) throws NoSuchElementException { if(item == "Life-threatening" || item== "Chronic" || item == "Major fracture" || item == "Walk-in"){ heapArray.addElement(item); trickleUp(item); }else{ throw new NoSuchElementException("Category does not exist!"); } } private void trickleUp(E item){ int index = heapArray.size()-1; int parent = (index -1) / 2 ; while (parent >=0 && compareItems(heapArray.get(index), heapArray.get(parent)) E temp = heapArray.get(parent); heapArray.set(parent, heapArray.get(index)); heapArray.set(index,temp); index = parent; parent = (index -1) / 2; } } public E getRootItem() { return heapArray.get(0); } public E heapDelete(){ E rootItem = null; int index; if (!heapIsEmpty()){ rootItem = heapArray.get(0); index = heapArray.size() - 1; heapArray.set (0, heapArray.get(index)); heapArray.remove (index); heapRebuild(0); } return rootItem; } private void heapRebuild (int root){ int child = 2*root +1; if (child int rightChild = child +1; if ( (rightChild child = rightChild; } } if (compareItems(heapArray.get(root), heapArray.get(child))>0){ E temp = heapArray.get(root); heapArray.set(root,heapArray.get(child)); heapArray.set(child,temp); heapRebuild(child); } } public int compareItems(E item1, E item2){ if (comparator == null){ return (item1).compareTo(item2); } else { return comparator.compare(item1, item2); } } public static void main (String [] args){ } } import java.util.NoSuchElementException; /* THIS PART NEEDS TO BE COMPLETED HELP * The shell of the class, to be completed as part of the * CSC115 Assignment 5 : Emergency Room */ public class PriorityQueue private Heap public PriorityQueue() { heap = new Heap } public boolean pqIsEmpty(){ return heap.heapIsEmpty(); } public void pqInsert ( E newItem) { heap.heapInsert (newItem); } public E pqDelete () { return heap.heapDelete(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
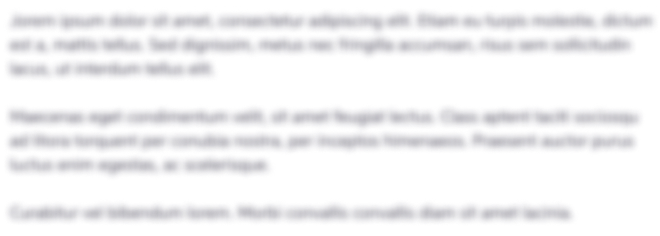
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started