Question
This is done by Java Tasks Using the Library and Book classes has been provided for you to familiarize yourself with it. The business rules
This is done by Java
Tasks
Using the Library and Book classes has been provided for you to familiarize yourself with it. The business rules that the Book is supposed to implement:
- a book must have an author (cannot be null)
- a book must have a title (cannot be null), and it should be less than 100 characters long
- a book is supposed to have between 100 and 1000 pages (fewer than 100 is considered a pamphlet, and more than 1000 is considered an encyclopaedia)
- a book in our database may not have 666 pages (that is considered bad luck)
Create a BookTest class in your project. The goal of your BookTest class is to decide whether or not the Book class is behaving properly. If it isn't, then your class should say why Book fails the tests (hint: this would be in the messages displayed for a failure).
We want to test all of the constructor and mutator methods for Book. Why don't we call our methods testConstructor, testAuthor, testTitle and testPages.
Use assertEquals and fail method mostly.
EX)
@Test public void testPages() throws Exception { int[] good = {100, 101, 500, 999, 1000}; Book book = new Book(); for (int value : good) { book.setPages(value); assertEquals("Positive #pages test", value, book.getPages()); } int[] bad = {-1, 0, 99, 1001, 123456}; for (int value : bad) { try { book.setPages(value); fail("Didn't catch bad #pages value - " + value); } catch (Exception e) { // the mutator method caught the bad value and threw an exception } } }
Although I don't know if this works correctly.
Book Class
package com.company; /** * Model for a Book that might be found in a library. */ public class Book { public final int MIN_PAGES = 100; // min # of pages public final int MAX_PAGES = 1000; // max # of pages private String author; // who wrote it private String title; // what it is called private int pages; // how long it is /** * Default constructor */ public Book() { author = ""; title = ""; pages = 0; } /** * Constructor with all fields specified. */ public Book(final String who, final String what, int howLong) { setAuthor(who); setTitle(what); setPages(howLong); } /** * Get the author. */ public String getAuthor() { return author; } /** * Set the author. **/ public void setAuthor(String who) { if (who != null) { author = who; } else { author = "Unknown"; } } /** * Get the title. */ public String getTitle() { return title; } /** * Set the title. */ public void setTitle(String what) { title = what; } /** * Get the page count. */ public int getPages() { return pages; } /** * Set the page count. */ public void setPages(int howMany) { pages = howMany; if (pages < MIN_PAGES) { pages = MIN_PAGES; } if (pages >= MAX_PAGES) { pages = MIN_PAGES; } } /** * Format the data nicely. */ public String toString() { return author + ": " + title + " (" + pages + " pages)"; } }
Library Class
package com.company; import java.util.*; /** * Manage a collection of books */ public class Library { /** * Create & show a sample collection of books */ public static void main(final String[] args) { SortedMap library = new TreeMap(); library.put("0-345-33858-8", new Book("Piers Anthony", "On A Pale Horse", 325)); library.put("0-06-105640-5", new Book("Greg Bear", "Foundation and Chaos", 408)); library.put("0-446-61161-1", new Book("Michael Connelly", "City Of Bones", 432)); library.put("0-441-11773-2", new Book("William Gibson", "Count Zero", 246)); library.put("0-812-51106-9", new Book("Larry Niven", "Destiny's Road", 433)); library.put("0-671-31974-4", new Book("Spider Robinson", "By Any Other Name", 429)); library.put("0-451-19969-3", new Book("Michael Slade", "Burnt Bones", 408)); System.out.println("--- Jim's Library ---"); for (String key : library.keySet()) { Book b = library.get(key); System.out.println(key + ": " + b.toString()); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
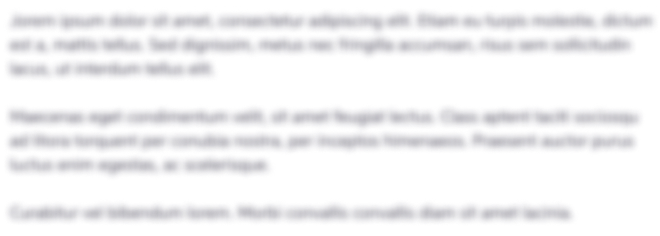
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started