Question
This is for Java can someone please solve this. import java.util.*; public class BagelsGame { // constants public static final int NUM_DIGITS = 4; //
This is for Java can someone please solve this.
import java.util.*;
public class BagelsGame {
// constants
public static final int NUM_DIGITS = 4; // number digits to guess
public static final String NONE_CORRECT = "bagels";
public static final String CORRECT_DIGIT = "fermi";
public static final String CORRECT_POSITION = "pico";
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
directions();
do {
playOneGame(console);
} while (playAgain(console));
}
/**
* This method gives the directions for the Bagels Game.
*/
public static void directions() {
System.out.println("Welcome to Bagels! ");
System.out.println("I'm thinking of a " + NUM_DIGITS + " digit number. Each digit is");
System.out.println("between 1 and 9. Try to guess my number. ");
System.out.println("I'll say \"fermi\" for each correct digit in the correct position.");
System.out.println("I'll say \"pico\" for each correct digit in the wrong position.");
System.out.println("I'll say \"bagels\" if all of the digits are wrong. ");
}
/**
*
* @param console
*/
public static void playOneGame(Scanner console) {
getUserGuess(console);
}
/**
*
*/
public static boolean playAgain(Scanner console){
return true;
}
/**
* Requires the user to continue to enter a guess until the guess is
* an integer with the correct number of digits
* @param console - facilitates input from the console window
* @return the user's guess
*/
public static int getUserGuess(Scanner console) {
int guess = 0;
boolean invalidGuess = true;
do {
System.out.print("Your guess? ");
try {
guess = console.nextInt();
if (guess int) Math.log10(guess) != NUM_DIGITS - 1) {
System.out.println("You must enter a positive integer with " + NUM_DIGITS + " digits. Try again.");
} else {
invalidGuess = false;
}
} catch (InputMismatchException e) {
System.out.println("You must enter an integer. Try again.");
}
console.nextLine();
} while (invalidGuess);
return guess;
}
}
public static void directions) System.out.println("Welcome to Bagels!n") System.out.println("I'm thinking of a " + NUM DIGITS + digit number. Each digit is"); System.out.println("between 1 and 9. Try to guess my number. "); System.out.println("I'1l say \"fermi\" for each correct digit in the correct position.") System.out.println("I'1l say \"pico\" for each correct digit in the wrong position.") System.out.println("I'1l say \"bagels\" if all of the digits are wrong.In") @param console public static void playOneGame (Scanner console) f getUserGuess((console); public static boolean playAgain(Scanner console) return true; Requires the user to continue to enter a guess until the guess is an integer with the correct number of digits @param console facilitates input from the console window @return the user's guess public static int getUserGuess (Scanner console) f int guess0; boolean invalidGuess = true; 0 System.out.print("Your guess? "); try guessconsole.nextInt); if (guess Once the user guesses the answer, the program reports the number of guesses nceded and prompts the user to play again. The user must enter "y" or "n" before the program either begins another game or the game ends. guessDigits Be sure you look at all of the sample output posted in Moodle and do your own testing. Notice that the digit 5 at index 0 of the guess is neither fermi match nor a pica match; there is digit 5 m the correct answer, but it is already claimed by its fermi match with index 1. Notice also that the digit 7 at index 3 of the guess only matches with the 7 at index in the answer and not also with te 7 at index 2. Implementation Details Do not modify the main method that has been provided. In this case, the computer's hint is "fermi pica pica" All of the fermi hints are reported first so as not to give any extra information to the user Four constants have been provided in the BagelsGamejava start file. Use those constants in your program. To determine fermi and pica hints, I recommend doing two passes over the array of digits: the first one to identify fermi matches (matching digits at matching positions) and the second one to identify pica matches (matching digits at different positions). You must be sure that the same digit is never used for two different matches. You w therefore need to mark digits as "used" so that they will not be used again in another match. For example, when the fermi match of 5" is found in the above example, those digits are marked used. Then looking for pica matches, the 7 in the answer at index 0and the 7 in the guess at index 3 are both marked so that when the 7 in the answer at index 2 is looking for a pica match, it can't find one among the unmarked values. Your program must have the following methods. You will likely need to use other methods to further decompose the program. No method can have more than 20 lines of code (not ounting blank lines and lines with only curly braces). public static void directions ) This method gives the directions for the game and has been provided in the start fi public static void playOneGame (Scanner console) This method plays one round of the game from the first "Your guess?" line through blank line following "You got it in X guesses. You must be sure that marking digits doesnt change the correct answer that the user needs to guess. Consider making a copy of the answer in a temporary array so that the temporary array can be "marked" Development Strategy public static boolean playAgain (Scanner console) This method asks the user if they want to play again and requires the user to enter "" or "n" before returning true when the user enteredy" orY" and false when the user entered "n" or "N". If the user enters any String other than "y", "Y". "n", or "N", the user is given an eror message and the is required to re-enter their answer until y". "Y". "T". or "N". See samples in Moodle I suggest starting by writing code to play a single game with the user. Initially, use a fixed value for the correct answer until you have the guessing and the hints working, Get the fermi hints working before attempting the pica hints. Then, make the answer randomly generated, but prin it out at the start of the game so that you can check the his that the game is giving You will likely want to use the Arrays class. In particular, the toString method to help with debugging and the equals method. public static int getUserGuess (Scanner console) This method requires the user to enter a positive integer with the correct number of digits and retums that positive integer. This method has been provided in the start file and will likely be called by the method playOneGame Style Guidelines Do not change the main method. Use all of the required methods. No method should have more than 20 lines of code not counting blank lines and lines with only curly braces. If any method has more than 20 lines of code, it should be decomposed into more metbods. public static int[] generateAnswer ) This method creates and returns a one dimensional array of length NUM DIGITS. It generates a random integer 1-9 for each element in the array. The elements of this array represent the number that the user is trying to guess. This method wi likely be called by playOneGame Follow good general Java style guidelines such as: appropriately using control structures like loops and ifelse statements; descriptive variable names; follow naming conventions; consistent indenting; using named constants. Don't use a break statement. Complete the program beader comment and write a comment for each method. public static String createHints (int] answerDigits, intl guessDigits) This method accepts two int arrays as parameters. The elements of one array represent the number the user is trying to guess, the answer. The clements of the other array represent the user's guess. The method should return a String which is the hints using the words "bagels", "fermi", and/or "pico" as describe previously Finishing Up Post Bagels.java to Moodle and give me a printout
Step by Step Solution
There are 3 Steps involved in it
Step: 1
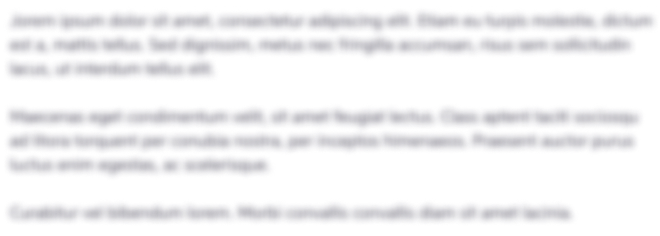
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started