Answered step by step
Verified Expert Solution
Question
1 Approved Answer
This is in java. All starter code given below. Part 3.3 is the implementation instructions. Please show that it works, thank you! I cannot import
This is in java. All starter code given below. Part 3.3 is the implementation instructions. Please show that it works, thank you! I cannot import any packages
IntStack.java:
import java.util.EmptyStackException; public class IntStack { /* instance variables, feel free to add more if you need */ private int[] data; private int nElems; private double loadFactor; private double shrinkFactor; private int arrSizeAkaCapacity; // new public static final double defaultShrinkF = 0.25; public static final double defaultLoadF = 0.75; public static final double defaultCapacity = 5; public IntStack(int capacity, double loadF, double shrinkF) { if (capacity 1) throw new IllegalArgumentException(); if (shrinkF 0.33) throw new IllegalArgumentException(); this.arrSizeAkaCapacity = capacity; this.nElems = 0; this.data = new int[capacity]; this.loadFactor = loadF; this.shrinkFactor = shrinkF; } public IntStack(int capacity, double loadF) { if (capacity 1) throw new IllegalArgumentException(); this.arrSizeAkaCapacity = capacity; this.loadFactor = loadF; this.shrinkFactor = defaultShrinkF; this.nElems = 0; this.data = new int[capacity]; } public IntStack(int capacity) { if (capacity = loadFactor) { int[] newData = new int[data.length * 2]; System.arraycopy(data, 0, newData, 0, nElems); data = newData; } if (nElems == data.length) { if (calcLoadFactor >= loadFactor) { int[] newData = new int[data.length * 2]; System.arraycopy(data, 0, newData, 0, nElems); data = newData; } } int lastPosition = nElems; data[lastPosition] = element; nElems += 1; } public int pop() { if (nElems == 0) throw new EmptyStackException(); int poppedElem = data[nElems - 1]; nElems = nElems - 1; double calcShrinkFactor = (double) nElems / data.length; if (calcShrinkFactorStringStack.java:
import java.util.EmptyStackException; public class StringStack { /* instance variables, feel free to add more if you need */ private String[] data; private int nElems; private double loadFactor; private double shrinkFactor; private int arrSizeAkaCapacity; public static final double defaultShrinkF = 0.25; public static final double defaultLoadF = 0.75; public StringStack(int capacity, double loadF, double shrinkF) { if (capacity 1) throw new IllegalArgumentException(); if (shrinkF 0.33) throw new IllegalArgumentException(); this.arrSizeAkaCapacity = capacity; this.nElems = 0; this.data = new String[capacity]; this.loadFactor = loadF; this.shrinkFactor = shrinkF; } public StringStack(int capacity, double loadF) { if (capacity 1) throw new IllegalArgumentException(); this.arrSizeAkaCapacity = capacity; this.loadFactor = loadF; this.shrinkFactor = defaultShrinkF; this.nElems = 0; this.data = new String[capacity]; } public StringStack(int capacity) { if (capacity = loadFactor) { String[] newData = new String[data.length * 2]; System.arraycopy(data, 0, newData, 0, nElems); data = newData; } if (nElems == data.length) { if (calcLoadFactor >= loadFactor) { String[] newData = new String[data.length * 2]; System.arraycopy(data, 0, newData, 0, nElems); data = newData; } } int lastPosition = nElems; data[lastPosition] = element; nElems += 1; } public String pop() { if (nElems == 0) throw new EmptyStackException(); String poppedElem = data[nElems - 1]; nElems = nElems - 1; // int[] newData = new int[arrSizeAkaCapacity]; // System.arraycopy(data, 0, newData, 0, nElems); // data = newData; double calcShrinkFactor = (double) nElems / data.length; if (calcShrinkFactorStarter code for part 3.3:
public class TextEditor { /* instance variables */ private String text; private IntStack undo; private StringStack deletedText; private IntStack redo; private StringStack insertedText; public TextEditor() { /* TODO */ } public String getText() { /* TODO */ return null; } public int length() { /* TODO */ return 0; } public void caseConvert(int i, int j) { /* TODO */ } public void insert(int i, String input) { /* TODO */ } public void delete(int i, int j) { /* TODO */ } public boolean undo() { /* TODO */ return false; } public boolean redo() { /* TODO */ return false; } }Part 3.1 Introduction In Part 3, you will use the stack to implement a text editor in the TextEditor.java file. The text editor will maintain a piece of text as String, and provides several editing operations along with an undo/redo functionality. The details of how these works is explained as follows: Operations: The user can use the following 3 options to edit the text. o caseConvert: Given a specific range, swap the cases of all letters within . this range. o . insert: Insert a new piece of text into the specified location. delete: Remove all characters within a specific range from the text. Undo/Redo: Undo the previous operation or redo the next operation. You will implement these features by making use of IntStack and StringStack. o Undo: The undo IntStack will maintain a history of each operation. deleted Text String Stack will maintain deleted texts. Whenever one of the above operations is made, you should push the 3 integer values of that operation (start, end, and a number code implying the type of an operation) to the undo stack before performing the operation. The code records which operation is performed. Use number 0 for caseConvert, 1 for insert, and 2 for delete. If the operation is Delete", you should also push the deleted text in the deleted Text stack. Here are some examples: caseConvert(2, 5): push (2,5,0) to the undo stack insert(1, "text"): push (1,5, 1) to the undo stack. 5 is the ending position (exclusive) of the inserted text in the original text. delete(2,4): suppose the original text is "text". Push (2, 4, 2) to the undo stack and "xt" to the deleted Text stack. When you try to undo the delete operation, get the deleted text from the deleted Text stack. This way, you will be able to undo an operation by popping out the 3 values from the undo stack and change the text back to its original. When you undo an insertion, you should push the inserted part of the text to the inserted Text stack. This way, when you try to redo o the insert operation, you will know what text to insert. The redo section below will cover the details. Redo is also considered as applying an operation, so you need to update the undo stack when redoing as well. Redo: The redo IntStack will maintain a series of operations that have been undone. The inserted Text stack will keep track of inserted text from when an insert operation is undo. Before undoing, you should push the 3 integers (start, end, and a number code implying the type of operation) to the redo stack. This way, you will be able to redo an undone operation by popping out the 3 values from the redo stack and redo the operation. The redo stack should be emptied before any operation is newly made. Don't forget to update the deleted Text stack when you redo a delete operation. o Why not record every version of text with a stringStack? Although this makes undo and redo much easier (just replace the current text with the last version), it takes a lot more memory to do so. Imagine you have ten pages of writing and you have to store a copy of it every time you type a character. Exceptions: Again you will need to throw certain exceptions under several circumstances (as described in the implementation details). Do NOT include throws in the method headers since they are runtime exceptions. However, you should include the exceptions in the docstrings with descriptions of when they will be thrown. . Part 3.2 StringStack You may find that having a stack class that works with String objects will be helpful to implement the undo and redo operations. In the String Stack.java file, copy and paste your implementation of IntStack and make necessary changes to reflect the change of data type. We won't test against this stack directly since the logics are the same as your integer stack, but you should make sure it works properly. Part 3.3 Implementation Constructors and methods to implement in TextEditor.java public TextEditor() Initialize a text editor. Make sure to initialize the text and the stacks you need to use. public String getText() Returns the text in the editor. public String length() Returns the length of the text in the editor. public void caseConvert(int i, int j) Swap the case of all characters of the text from position i (inclusive) to position i (exclusive). If any character is not a letter, keep it unchanged. Examples: Original text: Dsc.dSc.dsC" caseConvert(0, 1) -> "dsc.dSc.dsc" caseConvert(1, 4) -> "DSC.dSc.dsC" caseConvert(0, 11) -> "dSC.DsC.DSC" @throws IllegalArgumentException if i orj is out of bound, or i is not smaller than j public void insert(int i, String input) Insert the string input to the position i of the text. In other word, after the insertion, the start of the inserted string input should be at position i. Examples: Original text: "Dsc.dSc.dsc" insert(0, "CSE") -> "CSEDsc.dSc.dsC" insert(4, "CSE") -> "Dsc.CSEdSc.dsc" insert(11, "CSE") -> "Dsc.dSc.dsCCSE" @throws NullPointerException if input is null @throws IllegalArgumentException if i is out of bound public void delete (int i, int j) Remove the characters from position i (inclusive) to j (exclusive). Original text: "Dsc.dSc.dsc" remove(0, 1) -> "sc.dSc.dsc" remove(1, 4) -> "DdSc.dsc" remove(0, 11) -> @throws IllegalArgumentException if i orj is out of bound, or i is not smaller than j public boolean undo () Updates the text by undoing the latest operation. Returns false if there's no operation to undo, and true if undo was successful. Make sure it does not throw an Empty StackException. public boolean redo () Updates the text by redoing the next operation. Returns false if there's no operation to redo, and true if redo was successful. Make sure it does not throw an EmptyStackException
Step by Step Solution
There are 3 Steps involved in it
Step: 1
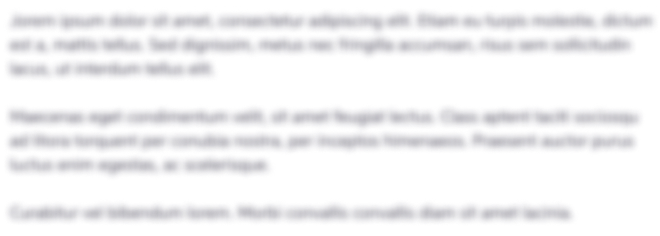
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started