Question
This is in java. Below is the interface in a file call RPSInterface.java. Please make sure you match the exact output needed. Sorry the write
This is in java. Below is the interface in a file call RPSInterface.java. Please make sure you match the exact output needed. Sorry the write up is quite long. Thank you!
/**
* An interface that specifies the functionality of RPS (Rock Paper Scissors)
*/
public interface RPSInterface {
/**
* Generates next cpu move
* @return String representing the move, depending on random int
*/
public String genCPUMove();
/**
* Takes the user move, the CPU move, and determines who wins.
* @param playerMove - move of the player
* @param cpuMove - move of the CPU
* @return -1 for invalid move, 0 for tie, 1 for player win, 2 for cpu win
*/
public int determineWinner(String playerMove, String cpuMove);
/**
* Determine if the move is valid
* @param move The move
* @return true if the move is valid, false otherwise
*/
public boolean isValidMove(String move);
/**
* Play one game of RPS.
* Also adds appropriately to the number of games, wins and ties played.
* and records the most recent moves.
* @param playerMove - move of the player
* @param cpuMove - move of the CPU
*/
public void play(String playerMove, String cpuMove);
/**
* Print out the end game stats: moves played and win percentages
*/
public void end();
}
To be clear: Below are what the methods to implement
Required output:
Inheritance hierarchy In this part of the assignment, you will create a computer game to play the game of Rock-Paper-Scissors between the computer and a user. We have split this into an interface, an abstract class, and a concrete class so you can get comfortable again with inheritance. The inheritance hierarchy is shown below. Please read through the code and make sure you understand how RPSInterface, RPSAbstract and RPS work together. Do not change the interface or the inheritance hierarchy. Interface RPSInterface Abstract class Concrete class RPSAbstract implements extends RPS Game Play Your job is to implement the missing methods to complete the implementation of the Rock-Paper-Scissors game. If you are unfamiliar with this game, you can read about it on Wikipedia here (though we will be implementing a slightly generalized version of the game). When the user starts your game, it should play the game of Rock Paper Scissors with the user until the user types 'q'. Here is an example run. User input is after the "(Type the move or q to quit)" $ javac RPS.java s java RPS Let's play! What's your move? (Type the move or q to quit) rock I chose scissors. You win. Let's play! What's your move? (Type the move or q to quit) rock I chose rock. It's a tie. Let's play! What's your move? (Type the move or q to quit) rock I chose paper. I win. Let's play! What's your move? (Type the move or q to quit) q Thanks for playing! Our most recent games were: Me: paper, You: rock Me: rock, You: rock Me: scissors, You: rock Our overall stats are: I won: 33.33% You won: 33.33% We tied: 33.33% Additional output examples can be viewed at the end of the writeup under "Example Output for Part 4". What to Implement Your task is to implement the following methods in the following files: RPSAbstract.java . boolean isValidMove (String move): Returns true if the move is valid and false otherwise. An invalid move is a move that is not in the possibleMoves array. . o o public void play (String playerMove, String cpuMove): Play one game of RPS using the moves provided. This method should: Determine the game outcome (hint: use determineWinner (see below)). Print an appropriate message as shown above (e.g. I chose scissors. You win."). Please use the provided static member variables for this purpose. Record the moves made by the CPU and the Player in the appropriate member variables (cpuMoves and playerMoves). Increment the number of games played and either the number of ties, CPU wins or player wins, depending on the outcome in the appropriate member variables (numPlayerWins, numCPUWins, numTies, and numGames) o O RPS.java public int determineWinner (String playerMove, String cpuMove): Given the two moves, determine the outcome of the game (-1 for invalid input, o for tie, 1 for RPS.java . o public int determineWinner (String playerMove, String cpuMove): Given the two moves, determine the outcome of the game (-1 for invalid input, o for tie, 1 for player win and 2 for cpu win). But this is not as simple as "rock beats scissors, "scissors beats paper" and "paper beats rock"! Here is how you should determine the outcome: All of the possible moves are stored in the array possibleMoves. Each element in the array beats the next element in the array, and the end wraps around to the beginning. All other pairings lead to a tie. This means each move beats one move and loses to one move. For example, { "elephant", "alligator", "hedgehog", "mouse" } indicates that elephant beats alligator, alligator beats hedgehog, hedgehog beats mouse and mouse beats elephant. (All other pairings tie). Your code should be able to handle any array of possible moves with at least 3 elements. Make sure you use the provided static member variables from RPSAbstract (CPU_WIN_OUTCOME, PLAYER_WIN_OUTCOME, TIE_OUTCOME, INVALID_INPUT_OUTCOME) in your return statements. O 0 public static void main: main method that reads user input, generates CPU moves, and plays the game. This method is partially completed and you will fill in the rest. o The game should repeat until the player enters "q" o If the player enters "q", then the game should end and the system should print out up to the last 10 games, in reverse order. If there have not been 10 games, it should print out as many as has been played. It should also print the win and tie statistics as in the example. Use the provided end() method to achieve this. If you have been updating the member variables as described above, everything should work. You can specify different moves using command line arguments. See examples under "Example Output for Part 4". o O If there is an invalid move, do not update any instance variables in the game. Do not store the player move or the CPU move in the playerMoves and cpuMoves arrays. Do not update any of the game stats (numGames, num Ties, numPlayerWins, numCPUWins). (Hint: Check if the move is valid before you call play and if it is not, do not call play). Call genCPUMove once each time you prompt the player for a new move. Even if the previous player move is invalid, generate a new move for the CPU. Use the provided static member variables inherited from RPSAbstract (PROMPT_MOVE, INVALID_INPUT) for printing to standard output. O O Additional Implementation Requirements You may not use additional Java packages from what is given. Please do not include your own import statements. You should be able to do the assignment with what is given. You will write your code for the methods described above. Please do not alter the provided variable names in the starter code, we will test your code using these variables. Additionally, do not delete variables or change the number of arguments in the methods. Doing so will result in incorrect auto-graded results. Use all provided Strings for consistency. Example Output for Part 4 Enter 'q' immediately: $ java RPS Let's play! What's your move? (Type the move or q to quit) q Thanks for playing! Our most recent games were: Our overall stats are: I won: NaN% You won: NaN% We tied: NaN% More than 10 games: $ java RPS Let's play! What's your move? (Type the move or q to quit) rock I chose scissors. You win. Let's play! What's your move? (Type the move or q to quit) rock I chose rock. It's a tie. Let's play! What's your move? (Type the move or q to quit) rock I chose paper. I win. Let's play! What's your move? (Type the move or q to quit) paper I chose paper. It's a tie. Let's play! What's your move? (Type the move or q to quit) paper I chose paper. It's a tie. Let's play! What's your move? (Type the move or q to quit) paper I chose paper. It's a tie. Let's play! What's your move? (Type the move or q to quit) scissors I chose paper. You win. Let's play! What's your move? (Type the move or q to quit) scissors I chose rock. I win. Let's play! What's your move? (Type the move or q to quit) scissors I chose rock. I win. Let's play! What's your move? (Type the move or q to quit) rock I chose paper. I win. Let's play! What's your move? (Type the move or q to quit) paper I chose rock. You win. Let's play! What's your move? (Type the move or q to quit) q Thanks for playing! Our most recent games were: Me: rock, You: paper Me: paper, You: rock Me: rock, You: scissors Me: rock, You: scissors Me: paper, You: scissors Me: paper, You: paper Me: paper, You: paper Me: paper, You: paper Me: paper, You: rock Me: rock, You: rock Our overall stats are: I won: 36.36% You won: 27.27% We tied: 36.36% Invalid input: $ java RPS Let's play! What's your move? (Type the move or q to quit) abc That is not a valid move. Please try again. Let's play! What's your move? (Type the move or q to quit) rock I chose rock. It's a tie. Let's play! What's your move? (Type the move or q to quit) q Thanks for playing! Our most recent games were: Me: rock, You: rock Our overall stats are: I won: 0.00% You won: 0.00% We tied: 100.00% Using command line args: $ java RPS water fire ice ground electric Let's play! What's your move? (Type the move or q to quit) water I chose fire. You win. Let's play! What's your move? (Type the move or q to quit) fire I chose ice. You win. Let's play! What's your move? (Type the move or q to quit) ground I chose fire. It's a tie. Let's play! What's your move? (Type the move or q to quit) electric I chose ground. I win. Let's play! What's your move? (Type the move or q to quit) ice I chose electric. It's a tie. Let's play! What's your move? (Type the move or q to quit) q Thanks for playing! Our most recent games were: Me: electric, You: ice Me: ground, You: electric Me: fire, You: ground Me: ice, You: fire Me: fire, You: water Our overall stats are: I won: 20.00% You won: 40.00% We tied: 40.00%
Step by Step Solution
There are 3 Steps involved in it
Step: 1
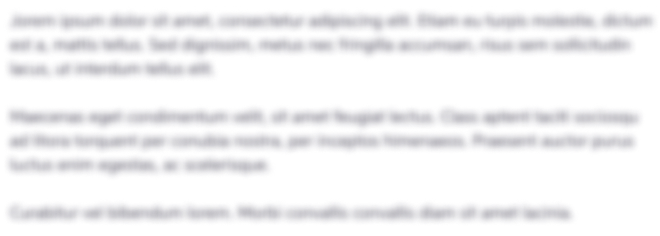
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started