Question
This is in Java. Here is the assignment: 1. Customer Inserter Write an application that connects to the CoffeeDB database, and allows the user to
This is in Java.
Here is the assignment:
1. Customer Inserter
Write an application that connects to the CoffeeDB database, and allows the user to insert a new row into the Customer table.
2. Customer Updater
Write an application that connects to the CoffeeDB database, and allows the user to select a customer, then change any of that customer's information. (You should not attempt to change the customer number, because it is referenced by the UnpaidOrder table.)
3. Unpaid Order Sum
Write an application that connects to the CoffeeDB database, then calculates and displays the total amount owed in unpaid orders. This will be the sum of each row s Cost column.
Here is my answer:
1.Program:
Customer Inserter:
import java.util.Scanner; import java.sql.*;
/** This program lets the user insert a row into the CoffeeDB database's Coffee table. */
public class CoffeeInserter { public static void main(String[] args) { String description; String prodNum; double price; final String DB_URL = "jdbc:derby:CoffeeDB"; Scanner keyboard = new Scanner(System.in); try { Connection conn = DriverManager.getConnection(DB_URL); System.out.print("Enter the coffee description: "); description = keyboard.nextLine(); System.out.print("Enter the product number: "); prodNum = keyboard.nextLine(); System.out.print("Enter the price: "); price = keyboard.nextDouble(); Statement stmt = conn.createStatement(); String sqlStatement = "INSERT INTO Coffee " + "(ProdNum, Price, Description) " + "VALUES ('" + prodNum + "', " + price + ", '" + description + "')"; int rows = stmt.executeUpdate(sqlStatement); System.out.println(rows + " row(s) added to the table."); conn.close(); } catch(Exception ex) { System.out.println("ERROR: " + ex.getMessage()); } } }
2. Customer Updater:
import java.util.Scanner; import java.sql.*;
/** This program lets the user change the price of a coffee in the CoffeeDB database's Coffee table. */
public class CoffeePriceUpdater { public static void main(String[] args) { String prodNum; double price;
final String DB_URL = "jdbc:derby:CoffeeDB";
Scanner keyboard = new Scanner(System.in);
try { Connection conn = DriverManager.getConnection(DB_URL);
Statement stmt = conn.createStatement();
System.out.print("Enter the product number: "); prodNum = keyboard.nextLine();
if (findAndDisplayProduct(stmt, prodNum)) { System.out.print("Enter the new price: "); price = keyboard.nextDouble();
updatePrice(stmt, prodNum, price); } else { System.out.println("That product was not found."); }
conn.close(); } catch(Exception ex) { System.out.println("ERROR: " + ex.getMessage()); } }
/** The findAndDisplayProduct method finds a specified coffee's data and displays it. */
public static boolean findAndDisplayProduct(Statement stmt, String prodNum) throws SQLException { boolean productFound;
String sqlStatement = "SELECT * FROM Coffee WHERE ProdNum = '" + prodNum + "'";
ResultSet result = stmt.executeQuery(sqlStatement);
if (result.next()) { System.out.println("Description: " + result.getString("Description")); System.out.println("Product Number: " + result.getString("ProdNum")); System.out.println("Price: $" + result.getDouble("Price"));
productFound = true; } else { productFound = false; }
return productFound; }
/** The updatePrice method updates a specified coffee's price. */
public static void updatePrice(Statement stmt, String prodNum, double price) throws SQLException {
String sqlStatement = "UPDATE Coffee " + "SET Price = " + Double.toString(price) + "WHERE ProdNum = '" + prodNum + "'";
int rows = stmt.executeUpdate(sqlStatement);
System.out.println(rows + " row(s) updated."); } }
3.Unpaid Order Sum:
public class Order implements unpaidordersum {
public List
if (status.equals("unpaid")) { control.relation("latest").uses(OrderingController.class).get(this); control.relation("cancel").uses(OrderingController.class).cancel(this); control.relation("payment").uses(OrderingController.class).pay(this,null); } return control.getRelations(); }
}
What is wrong with my last program? I get multiple errors, as shown in this screenshot:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
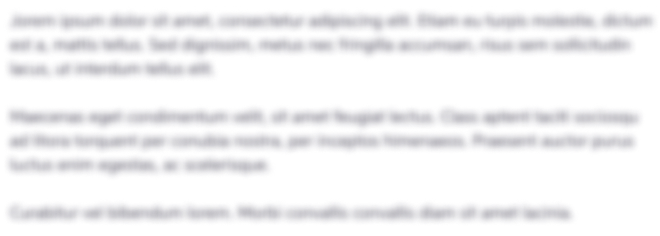
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started