Question
This is Java. Please help. I need PSEUDOCODE for the following source code: FuelGauge.java public class FuelGauge { // Declaring instance variables private int fuel_amount;
This is Java. Please help.
I need PSEUDOCODE for the following source code:
FuelGauge.java
public class FuelGauge {
// Declaring instance variables
private int fuel_amount;
final int CAPCITY = 15;
// Zero argumented constructor
public FuelGauge() {
fuel_amount = 0;
}
// This method will return the amount of fuel in the Tank
public int getFuel_amount() {
return fuel_amount;
}
// This method will fill the fuel
public void fillingFuel() {
while (fuel_amount < CAPCITY) {
fuel_amount += 1;
}
}
// This method will reduce the fuel
public void burningFuel() {
if (fuel_amount > 0)
fuel_amount -= 1;
}
}
__________________
Odometer.java
public class Odometer {
// Creating the reference of FuelGuage
FuelGauge fg;
// Parameterized constructor
public Odometer(FuelGauge fg) {
this.fg = fg;
}
public void runOdometer() {
int mileage = 0;
while (fg.getFuel_amount() > 0) {
for (int i = 1; i <= 24; i++) {
if (mileage == 99999) {
mileage = 0;
}
mileage++;
System.out.println("Mileage :" + mileage);
if (i == 24) {
fg.burningFuel();
}
System.out.println("Fuel Level :" + fg.getFuel_amount());
System.out.println("----------------------------------------");
}
}
}
}
____________________
Test.java
public class Test {
public static void main(String[] args) {
//Creating an instance of FuelGauge
FuelGauge f=new FuelGauge();
//filling fuel
f.fillingFuel();
//Creating an instance of Odometer
Odometer odo=new Odometer(f);
//calling the method on the Odometer
odo.runOdometer();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
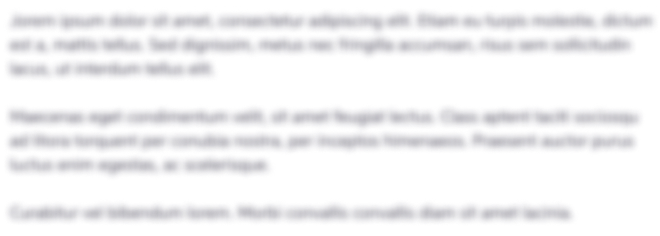
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started