Question
***This is my assignment: Project 2: Magic Square A magic square is a grid of unique numbers (i.e. no number can appear more than once)
***This is my assignment:
Project 2: Magic Square
A magic square is a grid of unique numbers (i.e. no number can appear more than once) in which the sum of all the rows, columns, and diagonals are the same. Here is an example in which all of the rows, columns and diagonals (upper left corner, center, lower right corner and upper right corner, center, and lower left corner) sum to 15. Generally, the numbers within a magic square range between 1 and n2, where n is the size of the side of the square.
4 | 9 | 2 |
3 | 5 | 7 |
8 | 1 | 6 |
Your goal for this project is to write a program that helps a user to create a magic square. Your program should meet the following requirements:
Initialization: A Magic square is a grid of numbers with n numbers along each side. For example, in the magic square above, n = 3. The value for n can technically be any positive number except 2 (because then there would be no diagonals), but due to hardware limitations it is probably a good idea to put an upper bound on n. For this program we will allow n to be at most 8. When your program begins its execution it should ask the user how big the magic square should be. For example:
Lets make a Magic Square! How big should it be? 2
That would violate the laws of mathematics!
Lets make a Magic Square! How big should it be? -4
That would violate the laws of mathematics!
Lets make a Magic Square! How big should it be? 12
Thats huge! Please enter a number less than 9.
Lets make a Magic Square! How big should it be? 4
Great!
Display: After the size has been chosen and in between each user input, your program should display the current state of the square. Use zeros to indicate empty places. For example, after the initialization above, your program should display:
The square currently looks like this:
0 0 0 0
0 0 0 0
0 0 0 0
0 0 0 0
Value entry: Allow the user to enter or change a value in the puzzle by providing the row and column of the value they want to change, along with the new value. Keep in mind that a user might want to overwrite an existing value. Also, remember that all numbers must be between 1 and n2. For example:
Where do you want to put a new value?
Row: 0
Column: 3
What value should go there? 2
The square currently looks like this:
0 0 0 2
0 0 0 0
0 0 0 0
0 0 0 0
Where do you want to put a new value?
Row: 0
Column: 3
What value should go there? 4
The square currently looks like this:
0 0 0 4
0 0 0 0
0 0 0 0
0 0 0 0
Where do you want to put a new value?
Row: 0
Column: 3
What value should go there? 191
You can only use numbers between 1 and 16 for this square.
The square currently looks like this:
0 0 0 4
0 0 0 0
0 0 0 0
0 0 0 0
Solution Recognition: When the magic square is complete, congratulate the user. For example:
The square currently looks like this:
7 12 1 14
2 13 8 11
16 3 10 5
9 6 15 4
Victory!
Your project will be evaluated according to the following rubric. Each item is worth ten points.
Initialization
Display
Value entry
Input validation (i.e. the user is never able to enter an invalid value for the size of the square, the row, the column, or the value to put in the square)
Solution recognition
Programming style and documentation
** I'll paste my code beow, but currently the two problems with my program are that I cannot get it to work whenever the user inputs a duplicate value (I want it to say something like "this is a duplicate value: please re-enter" and then have them input a new value) and then also I am supposed to allow the user to overwrite a value that they've already placed into the square, and that works; however, if they overwrite a value, it still increases the count so that in the end the square will not be completely full because the program would have stopped early. Any help would be appreciated, I've been stuck for a while.
package project2_kreger;
import java.util.Arrays; import java.util.Scanner;
public class Project2_Kreger {
// create a method that checks if the sum is a magic square //checks the sum of all of the rows, columns, and diagonals //returns false if any of them are not equal to the correct sum public static boolean sumCheck(int [][] square, int sumCheck){ for(int i = 0; i < square.length; i++){ int sum = 0; for(int j = 0; j < square.length; j++){ sum += square[i][j]; } if(sum != sumCheck){ return false; } } for(int j = 0; j < square.length; j++){ int sum = 0; for(int i = 0; i < square.length; i++){ sum += square[i][j]; } if(sum != sumCheck){ return false; } } int sum = 0; for(int i = 0; i < square.length; i++){ sum += square[i][square.length - 1 - i]; } if(sum != sumCheck){ return false; } sum = 0; for(int i = 0; i < square.length; i++){ sum += square[i][i]; } if(sum != sumCheck){ return false; } return true; } // create a method that checks the frequency of each number placed into the array // if the frequency is greater than 1, it will return false public static boolean checkFrequency(int [][] square, int size){ int matches = 0; boolean freq = true; //int [] frequency = new int[(size * size) + 1]; //for(int i = 1; i < frequency.length; i++) //frequency[i] = 0; for (int i = 0; i < square.length; i++) { matches = 0; for (int j = 0; j < square.length; j++) { if (square[i][j] < 1 || square[i][j] > size) { freq =false; } matches++; } } for(int i = 1; i < square.length; i++){ if(matches > 1) freq = false; } return freq; }
// create a method that checks the size and if it's less than 3, returns false public static boolean sizeCheckSmall(int sizeCheck1){ boolean size = true; if(sizeCheck1 < 3){ return false; } return true; } //create a method that checks the size and if it's greater than 8, returns false public static boolean sizeCheckBig(int sizeCheck2){ boolean size = true; if(sizeCheck2 > 8){ return false; } return true; } public static void main(String[] args) { Scanner input = new Scanner(System.in);
int count = 0; int size = 0; while (count == 0 || size < 3 || size > 8) { int valueNum = size * size; System.out.println("Let's make a magic square! How big should it be?"); size = input.nextInt();
if (size >= 3 && size <= 8) { System.out.println("Great!"); } while(sizeCheckSmall(size) == false){ System.out.println("That would violate the laws of mathematics!"); break; } while(sizeCheckBig(size) == false){ System.out.println("That's huge! Please enter a number less than 9"); break; } if (size >= 3 && size <= 8) {
System.out.println("The square currently looks like this: "); int [][] square = new int[size][size]; for (int i = 0; i < square.length; i++) { for (int j = 0; j < square.length; j++) { square[i][j] = 0; } } for (int i = 0; i < square.length; i++) { for (int j = 0; j < square.length; j++) { System.out.printf("%2d", square[i][j]);
} System.out.println(""); }
int count2 = 0; int row = 0; int column = 0; int value = 0; int i = 0; int j = 0;
while (count2 + 1 <= (Math.pow(size, 2))) { System.out.println("Where do you want to put a new value? Row:"); row = input.nextInt();
while (row > square.length - 1 || row < 0) { System.out.printf("You can only use numbers 0-%d for this " + "square:" + " Please re-enter ", square.length - 1); row = input.nextInt(); }
System.out.println("Column:"); column = input.nextInt();
while (column > square.length - 1 || column < 0) { System.out.printf("You can only use numbers 0-%d for this square: " + "" + "Please re-enter ", square.length - 1); column = input.nextInt(); }
System.out.println("What value should go there?"); value = input.nextInt(); while(square[row][column] == value){ for(i = 0; i < square.length; i++){ for(j = 0; j < square.length; j++){ if(checkFrequency(square, size) == false && count2 > 0){ System.out.println("This value is already in use, try again!"); value = input.nextInt(); } } } } if (value > size * size) { System.out.printf("You can only use numbers 1-%d for this square" + " ", valueNum); } else if (value < 1) { System.out.printf("You can only use numbers 1-%d for this square ", valueNum);
} System.out.println("The square currently looks like this: "); for (i = 0; i < square.length; i++) { for (j = 0; j < square.length; j++) { square[row][column] = value; while(checkFrequency(square, size) == false && count2 > 0){ for(i = 0; i < square.length; i++){ for(j = 0; j < square.length; j++){ System.out.println("This value is already in use, try again!"); value = input.nextInt(); } } } if (value > Math.pow(size, 2)) { square[row][column] = 0; } else if (value < 1) { square[row][column] = 0;
} else if (square[row][column] != value) { square[row][column] = 0; } System.out.printf("%2d", square[i][j]);
} System.out.println("");
} ++count2; count++; } int sumCheck = size * (size * size + 1)/ 2; while(count2 >= valueNum) if(sumCheck(square, sumCheck) == true){ System.out.println("Victory!"); break; } else if ((sumCheck(square, sumCheck) == false && checkFrequency(square, size) == false)){ System.out.println("Try again!"); break; } if(checkFrequency(square, size) == false ){ System.out.println("You cannot have more than one of the same value! "); break; } } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
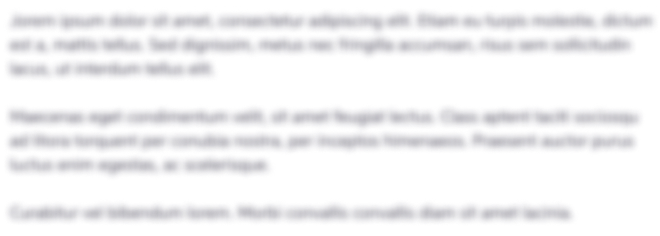
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started