Question
THIS IS MY CODE : public class WrongQuizGradeException extends Exception { WrongQuizGradeException(){ System.err.println(Quizz Grade Must Be Between 0 and 10!!!!); } } ===== import java.io.Serializable;
THIS IS MY CODE :
public class WrongQuizGradeException extends Exception {
WrongQuizGradeException(){
System.err.println("Quizz Grade Must Be Between 0 and 10!!!!");
}
}
=====
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Iterator;
public class Student implements Comparable
private String name;
private Integer ID;
private Double GPA;
private ArrayList
Student (String name, Integer ID, Double GPA){
this.name=name;
this.ID=ID;
this.GPA=GPA;
}
public void SetName(String n) {name=n;}
public void SetID(Integer id) {ID=id;}
public void SetGPA(Double GPA) { this.GPA=GPA; }
public String getName() {return name;}
public Integer getID() {return ID;}
public Double getGPA() { return GPA; }
public void addQuiz (double grade) throws WrongQuizGradeException {
if (grade10) {
throw new WrongQuizGradeException();
}
GradesList.add(grade);
}
public double quizzesAvg() {
Iterator
double sum=0.0;
while (I.hasNext()) {
sum+=I.next();
}
return sum/GradesList.size();
}
public void Print() {
System.out.println("Name: "+name+" ID: "+ID+" GPA: "+GPA);
System.out.println("The Quizes Grades:");
Iterator
while (I.hasNext()) {
System.out.print(I.next()+" ");
}
System.out.println(" The Avg: "+quizzesAvg()+" ");
}
/*
public String toString() {
return "Name: "+name+", ID:"+ID+" ,GPA:"+GPA+" ,The Quizz Avg: "+this.quizzesAvg()+" ";
}
*/
public int compareTo(Student s) {
return this.ID - s.ID;
}
}
======
class Node > {
//package access members
Node leftNode; // left node
T data; // node value
Node rightNode; // right node
//constructor initializes data and makes this a leaf node
public Node( T nodeData ) {
data = nodeData;
leftNode = rightNode = null; // node has no children
} // end TreeNode constructor
//locate insertion point and insert new node; ignore duplicate values
public void insert( T insertValue ) {
// insert in left subtree
if ( insertValue.compareTo( data )
// insert new TreeNode
if ( leftNode == null )
leftNode = new Node( insertValue );
else // continue traversing left subtree recursively
leftNode.insert( insertValue );
} // end if
// insert in right subtree
else if ( insertValue.compareTo( data ) > 0 ) {
// insert new TreeNode
if ( rightNode == null )
rightNode = new Node( insertValue );
else // continue traversing right subtree recursively
rightNode.insert( insertValue );
} // end else if
} // end method insert
} // end class TreeNode
=====
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.util.Formatter;
import java.util.FormatterClosedException;
class StudentTree > {
private static final String Student = null;
private Node root;
//constructor initializes an empty Tree of integers
public StudentTree() {
root = null;
} // end Tree no-argument constructor
//insert a new node in the binary search tree
public void insertNode( T insertValue ) {
if ( root == null )
root = new Node( insertValue ); // create root node
else
root.insert( insertValue ); // call the insert method
} // end method insertNode
//begin inorder traversal
public void inorderTraversal() {
inorderHelper( root );
} // end method inorderTraversal
//recursive method to perform inorder traversal
private void inorderHelper( Node node ) {
if ( node == null )
return;
inorderHelper( node.leftNode ); // traverse left subtree
((Student)(node.data)).Print(); // output node data
inorderHelper( node.rightNode ); // traverse right subtree
} // end method inorderHelper
public boolean search(T X) {
return searchHelper( root , X );
}
private boolean searchHelper( Node node, T X ) {
if ( node == null )
return false;
else
if (node.data==X)
return true;
return (searchHelper( node.leftNode, X) || searchHelper( node.rightNode, X));
}
public double MaxQuizzAvg(){
return MaxQAvgHelper( root );
}
double MAX=0;
private double MaxQAvgHelper(Node node) {
if (node==null)
return 0;
double X=((Student)(node.data)).quizzesAvg();
if ( X > MAX )
MAX = X;
MaxQAvgHelper( node.leftNode );
MaxQAvgHelper( node.rightNode );
return MAX;
}
public void QuizAvg(){
QuizAvgHelper( root );
}
private void QuizAvgHelper(Node node) {
if (node==null)
return;
double X=((Student)(node.data)).quizzesAvg();
System.out.println(X);
QuizAvgHelper( node.leftNode );
QuizAvgHelper( node.rightNode);
}
public void ChangeGPA(int ID, double GPA){
ChangeGPAHelper( root ,ID, GPA);
}
private void ChangeGPAHelper(Node node, int ID, double GPA) {
if (node==null)
return;
int X=((Student)(node.data)).getID();
if( ID == X ){
((Student)(node.data)).SetGPA(GPA);
}
ChangeGPAHelper( node.leftNode ,ID, GPA);
ChangeGPAHelper( node.rightNode ,ID ,GPA);
}
public double gpaAvg(){
return gpaSum( root) / NumOfStudent(root );
}
private double gpaSum(Node node) {
if (node==null)
return 0;
double GPASum= ((Student)node.data).getGPA() +gpaSum( node.leftNode) + gpaSum( node.rightNode );
return GPASum;
}
private int NumOfStudent(Node node) {
if (node==null)
return 0;
return 1+NumOfStudent( node.leftNode) + NumOfStudent( node.rightNode );
}
public void SaveTree(String FileName) {
FileOutputStream F=null;
ObjectOutputStream Out=null;
try {
F=new FileOutputStream(FileName);
Out=new ObjectOutputStream( F );
}catch( IOException e1 ) {
System.err.println("Error opening or creating file.");
}
SaveTreeHelper( root , Out );
}
private void SaveTreeHelper( Node
if (node==null)
return;
Student S = (Student)node.data;
try {
Out.writeObject(S);
} catch (IOException e) {
e.printStackTrace();
}
SaveTreeHelper( node.rightNode ,Out);
SaveTreeHelper( node.leftNode ,Out);
}
} // end class Tree
=====
public class Test {
public static void main(String[] args) {
Student S=new Student ("Ali",67,3.0);
try {
S.addQuiz(9);
} catch (WrongQuizGradeException e) {
e.getMessage();
}
try {
S.addQuiz(11);
} catch (WrongQuizGradeException e) {
e.getMessage();
}
try {
S.addQuiz(7);
} catch (WrongQuizGradeException e) {
e.getMessage();
}
try {
S.addQuiz(-1);
} catch (WrongQuizGradeException e) {
e.getMessage();
}
try {
S.addQuiz(5);
} catch (WrongQuizGradeException e) {
e.getMessage();
}
//System.out.println(S.quizzesAvg());
S.Print();
System.out.println(S);
}
}
======
import java.io.EOFException;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
public class BTest {
static void printFile(String f1) {
FileInputStream File=null;
ObjectInputStream In=null;
try {
File=new FileInputStream(f1);
In=new ObjectInputStream(File);
while( true ) {
Student S = (Student) In.readObject();
S.Print();
}
} catch (EOFException e1) {
System.out.println("The end of file was reached..");
} catch ( IOException ioException ) {
System.err.println("Error opening file.");
} catch ( ClassNotFoundException e2 ) {
System.err.println("class 'Student not found!'");
}
}
//In.close();
static StudentTree
FileInputStream File=null;
ObjectInputStream In=null;
StudentTree
try {
File=new FileInputStream(f1);
In=new ObjectInputStream(File);
while (true){
Student S = (Student) In.readObject();
//System.out.println("\t>> "+S);
STree.insertNode( S );
}
} catch (EOFException e1) {
System.out.println("The end of file was reached..");
} catch ( IOException ioException ) {
System.err.println("Error opening file.");
} catch ( ClassNotFoundException e2 ) {
System.err.println("class 'Student not found!'");
}
return STree;
}
public static void main(String[] args) {
Student []S=new Student[7];
S[0]=new Student("Ali",1,100.0);
S[1]=new Student("Haya",2,5.0);
S[2]=new Student("Nora",3,100.0);
S[3]=new Student("Sara",4,5.0);
S[4]=new Student("Nasir",5,100.0);
S[5]=new Student("Ahmed",6,5.0);
S[6]=new Student("Arwa",7,100.0);
try {
S[0].addQuiz(3);
S[0].addQuiz(3);
S[0].addQuiz(3);
System.out.println(S[0].quizzesAvg());
S[1].addQuiz(9);
S[1].addQuiz(7);
S[1].addQuiz(6);
System.out.println(S[1].quizzesAvg());
S[2].addQuiz(9);
S[2].addQuiz(2);
S[2].addQuiz(10);
S[3].addQuiz(9);
S[3].addQuiz(1);
S[3].addQuiz(10);
S[4].addQuiz(6);
S[4].addQuiz(6);
S[4].addQuiz(6);
S[5].addQuiz(4);
S[5].addQuiz(9);
S[5].addQuiz(8);
S[6].addQuiz(10);
S[6].addQuiz(10);
S[6].addQuiz(8);
S[6].addQuiz(9);
S[6].addQuiz(9);
} catch (WrongQuizGradeException e) {
e.printStackTrace();
}
FileOutputStream file = null;
ObjectOutputStream Output = null;
try {
file = new FileOutputStream("student1.ser");
Output = new ObjectOutputStream( file );
for(int i=0; i Output.writeObject( S[i] ); } } catch (EOFException e1) { System.out.println("The end of file was reached.."); } catch ( IOException ioException ) { System.err.println("Error opening file."); } System.out.println("====== The File ======="); printFile("student1.ser"); StudentTree STT=loadStudents("student1.ser"); System.out.println(" ========= In Order ========== "); STT.inorderTraversal(); System.out.println("The Students Gpa Avg= "+STT.gpaAvg()); STT.ChangeGPA(5, 300); System.out.println(" In Order After changing The GPA "); STT.inorderTraversal(); System.out.println("The Students Gpa Avg= "+STT.gpaAvg()); //GPAAvg Method System.out.println("============= "); System.out.println("The Maximum Quizzes Average Grade= "+STT.MaxQuizzAvg()); System.out.println(" ============="); STT.SaveTree("MA.ser"); System.out.println(" The new File Data "); printFile("MA.ser"); /* STT.insertNode(S2); STT.insertNode(S3); STT.insertNode(S1); STT.insertNode(S4); STT.insertNode(S5); STT.insertNode(S6); STT.insertNode(S7); */ } } AND I NEED TO DO THE FOLLOWING ( what in the photo ) with my above code pls help (in java programming language )
Step by Step Solution
There are 3 Steps involved in it
Step: 1
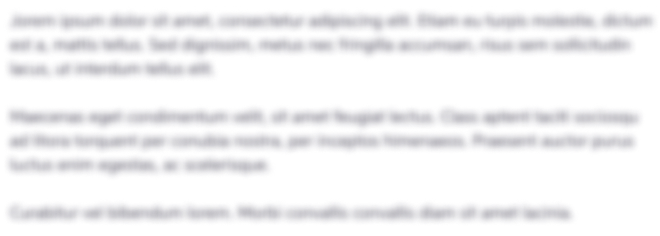
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started