Question
This is my code with the initial output at the bottom. I want to make the double number rounded to 2 decimals since it's representing
This is my code with the initial output at the bottom. I want to make the double number rounded to 2 decimals since it's representing money. how do I do that in this code?
package Lecture4;
public class BankAccount
{
//fields
private int ID;
private String name;
private double Saving;
private static int IDCOUNTER;
public BankAccount()
{
IDCOUNTER += 1;
ID = IDCOUNTER;
}
public BankAccount(String name)
{
IDCOUNTER += 1;
ID = IDCOUNTER;
this.name = name;
}
public BankAccount(String name, double Saving)
{
IDCOUNTER += 1;
ID = IDCOUNTER;
this.name = name;
this.Saving = Saving;
}
//Accessors
public String getName()
{
return name;
}
public int getID()
{
return ID;
}
public double getSaving()
{
return Saving;
}
//Modifiers
public void ChangeName()
{
this.name = name;
}
//toString
public String toString()
{
return "The name of this account is: " +this.name + " The ID of this account is: " + this.ID + " The Saving of this account is: " + this.Saving;
}
//Functions
public void Deposit(double M)
{
this.Saving += M;
}
public void withdraw(double M)
{
if(this.Saving >= M)
{
this.Saving -= M;
}
else System.out.println("This account " +ID+ " does not have enough money");
}
public void TransferTo(BankAccount B, double M)
{
if(this.Saving >= M)
{
B.Deposit(M);
withdraw(M);
}
else System.out.println("This Account " +ID+ " does not have enough money");
}
}
Output:
The name of this account is: A
The ID of this account is: 1
The Saving of this account is: 286.4511843177727
Step by Step Solution
There are 3 Steps involved in it
Step: 1
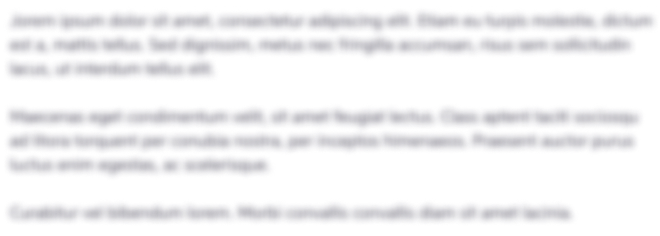
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started