Answered step by step
Verified Expert Solution
Question
1 Approved Answer
This is new homework: In this assignment you should turn your previously built data structure to use templates. With this, it should be possible to
This is new homework:
In this assignment you should turn your previously built data structure to use templates. With this, it should be possible to use your data structure with any type. In addition, you should also implement move constructors and assignments to your data structure too. Finally, demonstrate the use of your data structure using different types
This code:
StackInterface.h:
#ifndef _STACKINTERFACE_H_ #define _STACKINTERFACE_H_ class StackInterface { public: // absolutely necessary functions for a stack virtual void push(const int element) = 0; virtual int pop() = 0; virtual int peek() = 0; virtual bool isempty() const = 0; }; #endif // _STACKINTERFACE_H_
Stack.h:
#ifndef _STACK_H_ #define _STACK_H_ #include "StackInterface.h" #include#include class Stack : public StackInterface { public: // overriden methods void push(const int element) override; int pop() override; int peek() override; bool isempty() const override; // constructor Stack(); // additional methods int size() const; private: // member variable std::vector data; public: // part 2 // copy constructor Stack(const Stack& stack); // copy assignment operator Stack& operator=(const Stack& stack); // move constructor Stack(Stack&& stack); // move assignment operator Stack& operator=(Stack&& stack); // stream inserter friend std::ostream& operator<<(std::ostream& stream, const Stack& stack); // access operators int operator[](const int index) const; int operator()(const int index) const; // comparison operators bool operator==(const Stack& stack) const; bool operator!=(const Stack& stack) const; }; #endif // _STACK_H_
Stack.cpp:
#include "Stack.h" // overriden methods void Stack::push(const int element) { data.emplace_back(element); } int Stack::pop() { const int element{data.back()}; data.pop_back(); return element; } int Stack::peek() { return data.back(); } bool Stack::isempty() const { return data.empty(); } // constructor Stack::Stack() : data{} { } // additional methods int Stack::size() const { return data.size(); } // part 2 // copy constructor Stack::Stack(const Stack& stack) : data{stack.data} { } // copy assignment operator Stack& Stack::operator=(const Stack& stack) { data = stack.data; return *this; } // move constructor Stack::Stack(Stack&& stack) :data{stack.data} { stack.data.clear(); } // move assignment operator Stack& Stack::operator=(Stack&& stack) { data = stack.data; stack.data.clear(); return *this; } // stream inserter std::ostream& operator<<(std::ostream& stream, const Stack& stack) { stream << '['; for(const int element : stack.data) { stream << ' ' << element; } stream << " ]"; return stream; } // access operators int Stack::operator[](const int index) const { return data[index]; } int Stack::operator()(const int index) const { return data[index]; } // comparison operators bool Stack::operator==(const Stack& stack) const { return data == stack.data; } bool Stack::operator!=(const Stack& stack) const { return data != stack.data; }
Main.cpp:
#include "Stack.h" #include#include int main() { // testing StackInterface { StackInterface *stack{new Stack}; // [] assert(stack->isempty() == true); stack->push(1); stack->push(2); stack->push(3); stack->push(4); // [1, 2, 3, 4] assert(stack->isempty() == false); assert(stack->pop() == 4); assert(stack->pop() == 3); // [1, 2] stack->push(5); stack->push(6); // [1, 2, 5, 6] assert(stack->pop() == 6); assert(stack->pop() == 5); assert(stack->pop() == 2); assert(stack->pop() == 1); // [] assert(stack->isempty() == true); delete stack; } // testing part2 { Stack stack; stack.push(1); stack.push(2); stack.push(3); // copy constructor Stack stackCopy{stack}; assert(stackCopy.peek() == 3); assert(stack.peek() == 3); // copy assignment Stack stackCopyAssignment; stackCopyAssignment = stack; assert(stackCopyAssignment.peek() == 3); assert(stack.peek() == 3); // move constructor Stack stackMove{std::move(stack)}; assert(stackMove.peek() == 3); assert(stack.isempty() == true); // refilling stack stack.push(1); stack.push(2); stack.push(3); // move assignment operator Stack stackMoveAssignment{std::move(stack)}; assert(stackMoveAssignment.peek() == 3); assert(stack.isempty() == true); // refilling stack stack.push(1); stack.push(2); stack.push(3); // stream inserter std::stringstream ss; ss << stack; assert(ss.str() == "[ 1 2 3 ]"); // access operators assert(stack[0] == 1); assert(stack[1] == 2); assert(stack[2] == 3); assert(stack(0) == 1); assert(stack(1) == 2); assert(stack(2) == 3); // comparison operators Stack stack1; stack1.push(1); stack1.push(2); stack1.push(3); Stack stack2; stack2.push(1); stack2.push(2); stack2.push(4); assert((stack == stack1) == true); assert((stack != stack2) == true); } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
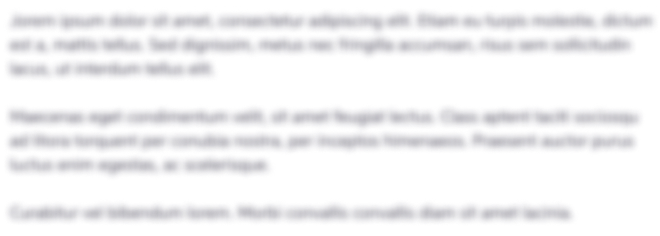
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started