Question
This is Player class for my battleship program. How should I Ignore adding more ships if there are already five ships? public class Player {
This is Player class for my battleship program. How should I Ignore adding more ships if there are already five ships?
public class Player { private static final int[] SHIP_LENGTHS = {2, 3, 3, 4, 5}; private Ship[] ships; private Grid own = new Grid(); private Grid opp = new Grid(); public Player() { ships = new Ship[SHIP_LENGTHS.length]; for (int i = 0; i < SHIP_LENGTHS.length; i++){ ships[i] = new Ship(SHIP_LENGTHS[i]); } } public void printMyShips() { own.printShips(); } public void printOpponentGuesses() { opp.printStatus(); } public void printMyGuesses() { own.printStatus(); } public void chooseShipLocation(Ship s, int row, int col, int direction) { s.setLocation(row, col); s.setDirection(direction); own.addShip(s); } public void recordOpponentGuess(int row, int col) { if (own.hasShip(row, col)) { opp.markHit(row, col); } else opp.markMiss(row, col); } }
// The ship class.
public class Ship { public static final int UNSET=-1; public static final int HORIZONTAL=0; public static final int VERTICAL=1;
private int row=UNSET; private int col=UNSET; private int length=UNSET; private int direction = UNSET; public Ship(int length) { this.length = length; } // Has the location been initialized public boolean isLocationSet() { return row != UNSET && col != UNSET; } // Has the direction been initialized public boolean isDirectionSet() { return direction != UNSET; } // Set the location of the ship public void setLocation(int row, int col) { this.row = row; this.col = col; } // Set the direction of the ship public void setDirection(int direction) { this.direction = direction; } // Getter for the row value public int getRow() { return row; } // Getter for the column value public int getCol() { return col; } // Getter for the length of the ship public int getLength() { return length; } // Getter for the direction public int getDirection() { return direction; } // Helper method to get a string value from the direction private String directionToString() { if( direction == UNSET) return "unset direction"; else if (direction == VERTICAL) return "vertical"; else return "horizontal"; } // Helper method to get a (row, col) string value from the location private String locationToString() { if( row == UNSET || col == UNSET) return "(unset location)"; else return "(" + row +", " + col+")"; } // toString value for this Ship public String toString() { return directionToString() + " ship of length " + length + " at " + locationToString(); } }
// Location class
public class Location { //Implement the Location class here public static final int UNGUESSED = 0; public static final int HIT = 1; public static final int MISSED = 2; private int status; private boolean shipPresent; // Location constructor. public Location() { status=UNGUESSED; shipPresent = false; } // Was this Location a hit? public boolean checkHit() { return status == HIT; } // Was this location a miss? public boolean checkMiss() { return status == MISSED; } // Was this location unguessed? public boolean isUnguessed() { return status == UNGUESSED; } // Mark this location a hit. public void markHit() { status = HIT; } // Mark this location a miss. public void markMiss() { status = MISSED; } // Return whether or not this location has a ship. public boolean hasShip() { return shipPresent; } // Set the value of whether this location has a ship. public void setShip(boolean val) { shipPresent = val; } // Set the status of this Location. public void setStatus(int status) { this.status = status; } // Get the status of this Location. public int getStatus() { return status; } }
// Grid class
public class Grid { // Write your Grid class here
private Location[][] grid;
// Constants for number of rows and columns. public static final int NUM_ROWS = 10; public static final int NUM_COLS = 10; // Create a new Grid. Initialize each Location in the grid // to be a new Location object. public Grid() { grid = new Location[10][10]; for(int row=0; row // Get the status of this location in the grid public int getStatus(int row, int col) { return grid[row][col].getStatus(); } // Return whether or not this Location has already been guessed. public boolean alreadyGuessed(int row, int col) { return ! grid[row][col].isUnguessed(); } // Set whether or not there is a ship at this location to the val public void setShip(int row, int col, boolean val) { grid[row][col].setShip(val); } // Return whether or not there is a ship here public boolean hasShip(int row, int col) { return grid[row][col].hasShip(); } // Get the Location object at this row and column position public Location get(int row, int col) { return grid[row][col]; } // Return the number of rows in the Grid public int numRows() { return NUM_ROWS; } // Return the number of columns in the grid public int numCols() { return NUM_COLS; } public void printStatus() { System.out.println(" 1 2 3 4 5 6 7 8 9 10"); for( int row=0; row }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
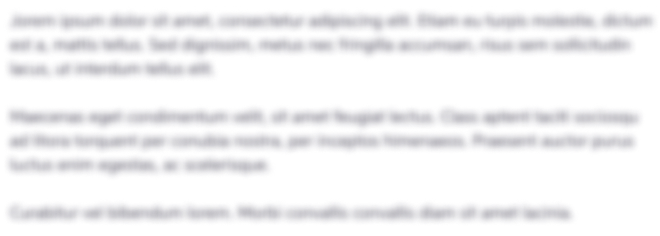
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started