Question
This is the code for how to crete an alarm clock. All is set but I need a bit difference like the alarm beep util
This is the code for how to crete an alarm clock. All is set but I need a bit difference like the alarm beep util the user stops it means if we create a button like stop button which will stop the alarm when user press the button. and another thing if we can make it possible like when the user ask the input for the alarm it also ask for the small note which will pop up when alarm went up.
Please help me in this as soon as possible. I really need that. Thank you.
All the code is down but just want to make it beep util user stop it and also it ask for the note
// AlarmClock.java
import java.awt.Color;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import javax.sound.sampled.AudioInputStream;
import javax.sound.sampled.AudioSystem;
import javax.sound.sampled.Clip;
import javax.sound.sampled.LineUnavailableException;
import javax.sound.sampled.UnsupportedAudioFileException;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.Timer;
public class AlarmClock extends JFrame {
private static int HEIGHT = 200, WIDTH = 700;
private boolean alarmSet;
Date currentTime;
int alarmHours, alarmMinutes;
SimpleDateFormat sdf;
JLabel timeText;
Timer clockTimer;
/**
* replace the file with your file name, make sure the sound exist in same
* project directory
*/
File soundFile = new File("alarm_sound.wav");
public AlarmClock() {
// setting up attributes
setDefaultCloseOperation(EXIT_ON_CLOSE);
setSize(WIDTH, HEIGHT);
alarmSet = false;
currentTime = Calendar.getInstance().getTime();
sdf = new SimpleDateFormat("hh:mm:ss");// date to time formatter
setLayout(new BoxLayout(getContentPane(), BoxLayout.Y_AXIS));
add(createClockPanel());// digital clock
add(createAlarmPanel());// textfield, button for alarm
setupTimer();// to update the time in every second
setVisible(true);
}
/**
* method to setup the panel for digital clock and return it
*/
private JPanel createClockPanel() {
JPanel clockPanel = new JPanel();
timeText = new JLabel();
timeText.setFont(new Font(Font.SANS_SERIF, Font.PLAIN, 70));
timeText.setText(sdf.format(currentTime));
clockPanel.add(timeText);
clockPanel.setBackground(Color.CYAN);
return clockPanel;
}
/**
* method to setup the panel for alarm scheduling and return it
*/
private JPanel createAlarmPanel() {
JPanel panel = new JPanel();
panel.setLayout(new FlowLayout());
JLabel label = new JLabel("Enter Alarm Time(HH:MM format): ");
label.setForeground(Color.WHITE);
final JTextField inputField = new JTextField(10);
JButton scheduleBtn = new JButton("Schedule Alarm");
panel.add(label);
panel.add(inputField);
panel.add(scheduleBtn);
// adding listener for schedule button
scheduleBtn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent arg0) {
try {
/**
* verifying the input before setting alarm
*/
String txt = inputField.getText();
String fields[] = txt.split(":");
alarmHours = Integer.parseInt(fields[0]);
alarmMinutes = Integer.parseInt(fields[1]);
if (alarmHours >= 0 && alarmHours < 24 && alarmMinutes >= 0
&& alarmMinutes < 60) {
/**
* Alarm is set
*/
alarmSet = true;
JOptionPane.showMessageDialog(null, "Alarm set!");
inputField.setText("");
} else {
JOptionPane.showMessageDialog(null, "Invalid time");
}
} catch (Exception e) {
JOptionPane.showMessageDialog(null, "Invalid time");
}
}
});
panel.setBackground(Color.GRAY);
return panel;
}
/**
* @return the current hours in integer format
*/
public int getHours() {
String time = sdf.format(currentTime);
return Integer.parseInt(time.split(":")[0]);
}
/**
* @return the current minutes in integer format
*/
public int getMinutes() {
String time = sdf.format(currentTime);
return Integer.parseInt(time.split(":")[1]);
}
/**
* method to setup the timer which will update the digital clock every
* second
*/
private void setupTimer() {
clockTimer = new Timer(1000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent arg0) {
currentTime = Calendar.getInstance().getTime();
timeText.setText(sdf.format(currentTime));
if (alarmSet) {
/**
* checking if alarm time has been crossed
*/
if (getHours() >= alarmHours
&& getMinutes() >= alarmMinutes) {
alarmSet = false;
playSound();//playing sound
//showing a message
JOptionPane.showMessageDialog(null, "Alarm!!!");
}
}
}
});
clockTimer.start();//starting the timer
}
/**
* method to play a short sound when alarm goes up
*/
private void playSound() {
try {
AudioInputStream audioInputStream = AudioSystem
.getAudioInputStream(soundFile);
Clip clip = AudioSystem.getClip();
clip.open(audioInputStream);
clip.start();
} catch (UnsupportedAudioFileException e) {
System.err.println(e);
} catch (IOException e) {
System.err.println(e);
} catch (LineUnavailableException e) {
System.err.println(e);
}
}
public static void main(String[] args) {
new AlarmClock();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
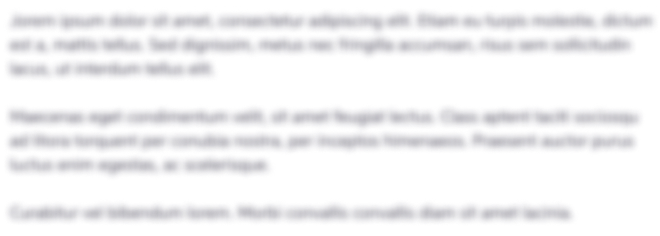
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started