Question
This is the code I have so far, Need decode and main function done ASAP, DUE IN 1 HOUR ''' Insert heading comments here.''' MENU
This is the code I have so far, Need decode and main function done ASAP, DUE IN 1 HOUR
''' Insert heading comments here.'''
MENU = ''' Please choose one of the options below:
A. Convert a decimal number to another base system
B. Convert decimal number from another base.
C. Convert from one representation system to another.
E. Encode an image with a text.
D. Decode an image.
M. Display the menu of options.
X. Exit from the program.'''
def numtobase(N, B):
'''Insert docstring here.'''
if N == 0:
return''
digits = []
while N > 0:
digits.append(str(N % B))
N //= B
digits += ['0'] * (-len(digits) % 8)
return ''.join(digits[::-1])
pass # insert your code here
def basetonum(S, B):
'''Insert docstring here.'''
if S == '':
return 0
else:
decimal = 0
power = 0
for digit in S[::-1]:
decimal += int(digit) * (B ** power)
power += 1
return decimal
def basetobase(B1, B2, s_in_B1):
'''Insert docstring here.'''
decimal = basetonum(s_in_B1, B1)
if decimal == 0:
return ''
output = []
quotient = decimal
while quotient > 0:
remainder = quotient % B2
quotient //= B2
output.append(str(remainder))
output += ['0'] * (-len(output) % 8)
return ''.join(output[::-1])
def encode_image(image, text, N):
"""
Embeds a text message in a binary image using the LSB algorithm.
Parameters:
image (str): The binary string representation of the image.
text (str): The text message to be hidden in the image.
N (int): The number of bits used to represent each pixel.
Returns:
str: The encoded binary string representation of the image with the text message embedded.
If the image is empty, an empty string is returned.
If the text is empty, the original image is returned.
If the image is not big enough to hold all the text, None is returned.
"""
if not image:
return ""
if not text:
return image
# Calculate the maximum length of the text that can be embedded in the image
max_text_len = len(image) // N
if len(text) > max_text_len:
return None
# Convert the text message into binary
text_binary = "".join(numtobase(ord(c),2) for c in text)
# Embed the binary text message in the image
encoded_image = list(image)
for i in range(len(text_binary)):
# Calculate the index of the pixel where the current bit of the text message should be embedded
pixel_index = i * N + (N - 1)
# Check if the LSB of the pixel is the same as the current bit of the text message
if encoded_image[pixel_index] != text_binary[i]:
# If not, replace the LSB of the pixel with the current bit of the text message
encoded_image[pixel_index] = text_binary[i]
return "".join(encoded_image)
def decode_image(sego, N):
'''Insert docstring here.'''
# Calculate the length of the hidden text in bits
text_length = int(sego[:32], 2)
# Convert the stego string to a list of integers representing the pixel values
pixels = [int(sego[i:i+N], 2) for i in range(32, len(sego), N)]
# Convert the pixel values to a binary string and pad with zeros if necessary
binary_text = ''.join(format(pixel, f'0{N}b') for pixel in pixels)
binary_text = binary_text[:text_length]
# Convert the binary string to the hidden text
text = ''.join(chr(int(binary_text[i:i+8], 2)) for i in range(0, len(binary_text), 8))
return text
def main():
BANNER = '''
A long time ago in a galaxy far, far away...
A terrible civil war burns throughout the galaxy.
~~ Your mission: Tatooine planet is under attack from stormtroopers,
and there is only one line of defense remaining
It is up to you to stop the invasion and save the planet~~
'''
print(BANNER)
pass # insert your code here
# These two lines allow this program to be imported into other code
# such as our function tests code allowing other functions to be run
# and tested without 'main' running. However, when this program is
# run alone, 'main' will execute.
# DO NOT CHANGE THESE 2 lines
if __name__ == '__main__':
main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
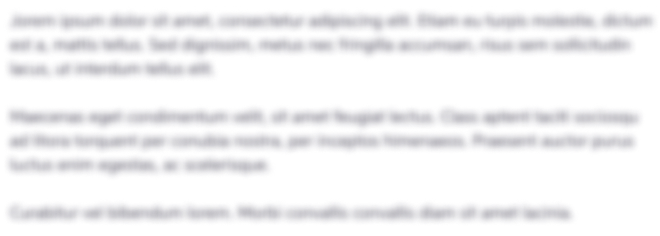
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started