Answered step by step
Verified Expert Solution
Question
1 Approved Answer
This is the code. You should 1)Modify the program in which the maze changes everytime we run the program 2) Find the time it takes
This is the code. You should 1)Modify the program in which the maze changes everytime we run the program
2) Find the time it takes from the beginning of the maze till the end.
3)Make competition where you execute the time it takes for each player to reach the end of the maze.
#include
#include
#include
#include
#include
#include
using namespace std;
const int KEY_LEFT = 'a', KEY_RIGHT = 'd',KEY_UP = 'w', KEY_DOWN ='s';
const int HEIGHT =25, WIDTH = 40;
bool hasntWon = true;
int hP = 100;
enum DirectionX {Right,Left};
enum DirectionY {Up,Down};
struct Player {
char sprite;
int x;
int y;
};
struct Enemy{
char sprite;
int x;
int y;
DirectionX pastDirX;
DirectionY pastDirY;
};
unsigned char MoveEnemy(Enemy& enemy, unsigned char maze[HEIGHT][WIDTH], int i , int j , string& smove, bool& nc){
if (nc){
bool psbUp = false;
bool psbDown = false;
bool psbRight = false;
bool psbLeft = false;
if (maze[enemy.y][enemy.x+1] != '#' ){
psbRight = true;
}
if (maze[enemy.y][enemy.x-1] != '#' ){
psbLeft = true;
}
if (maze[enemy.y+1][enemy.x] != '#' ){
psbLeft = true;
}
if (maze[enemy.y-1][enemy.x] != '#') {
psbLeft = true;
}
enum Mv {up,right,down,left,NA};
Mv move = NA;
if (smove =="up"){
move = up;
}
else if (smove =="right"){
move = right;
}
else if (smove =="down"){
move = down;
}
else if (smove =="left"){
move = left;
}
else {
move = NA;
}
if (enemy.pastDirX == Right && !psbRight){
if(enemy.pastDirY == Up){
if (psbUp){
move = up;
}
else if (psbDown && move != up){
move = down;
}
else if (psbLeft){
move = left;
}
else {
move = down;
}
}
else{
if(psbDown){
move = down ;
}
else if(psbUp && move != down){
move = up;
}
else if (psbLeft){
move = left;
}
else{
move = up;
}
}
}
else if (enemy.pastDirX == Right && psbRight){
move = right;
}
else if (enemy.pastDirX == Left && !psbLeft){
if(enemy.pastDirY == Up) {
if (psbUp){
move = up;
}
else if(psbDown && move != up){
move = down;
}
else if (psbRight){
move = right;
}
else {
move = down;
}
}
else {
if(psbDown){
move = down;
}
else if (psbUp && move != down){
move = up;
}
else if (psbRight){
move = right;
}
else {
move = up;
}
}
}
else if (enemy.pastDirX == Left && psbLeft){
move = left;
}
else if(enemy.pastDirY == Up && !psbUp){
if (enemy.pastDirX == Right){
if (psbRight){
move = right;
}
else if (psbLeft && move != right){
move = left;
}
else if (psbDown){
move = down;
}
else {
move = left;
}
}
else {
if (psbLeft ){
move = left;
}
else if (psbRight && move != left){
move = right;
}
else if (psbDown){
move = down;
}
else {
move = right;
}
}
}
else if (enemy.pastDirY == Up && psbUp){
move = up;
}
else if (enemy.pastDirY == Down && !psbDown){
if (enemy.pastDirX == Right){
if (psbRight){
move = right;
}
else if (psbLeft && move != right){
move = left;
}
else if (psbUp){
move = up;
}
else {
move = left;
}
}
else {
if (psbLeft){
move = left;
}
else if (psbRight && move != left ){
move = right ;
}
else if (psbRight){
move = up;
}
else {
move = right ;
}
}
}
else if (enemy.pastDirY == Down && psbDown){
move = down;
}
switch (move) {
case up:
maze[enemy.y][enemy.x] = ' ';
enemy.pastDirY = Up;
smove = "up";
enemy.y--;
break ;
case right:
maze[enemy.y][enemy.x] = ' ';
enemy.pastDirX = Right;
smove = "right";
enemy.x++;
break ;
case down:
maze[enemy.y][enemy.x] = ' ';
enemy.pastDirY = Down;
smove = "down";
enemy.y++;
break ;
case left:
maze[enemy.y][enemy.x] = ' ';
enemy.pastDirX = Left;
smove = "left";
enemy.x--;
break ;
case NA:
smove = "NA";
break;
}
}
nc = false;
return maze[i][j];
}
int main()
{
int x,y,h,i,j;
srand(time(NULL));
unsigned char maze [HEIGHT][WIDTH]={{'#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#'},
{' ',' ',' ',' ',' ',' ',' ','#',' ','#',' ',' ',' ','#','#',' ','#',' ',' ',' ',' ',' ','#',' ',' ',' ','#',' ',' ',' ',' ',' ',' ',' ','#',' ',' ',' ',' ','#'},
{'#','#','#','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ','#',' ','#','#','#',' ','#','#','#',' ','#',' ','#','#','#',' ','#',' ','#',' ','#','#',' ','#'},
{'#',' ',' ',' ',' ','#',' ',' ',' ','#',' ','#',' ','#',' ','#','#',' ',' ',' ','#',' ',' ',' ',' ',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ','#'},
{'#',' ','#','#',' ','#','#','#',' ','#',' ','#',' ','#',' ',' ',' ',' ','#',' ','#','#','#','#','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ','#'},
{'#',' ','#',' ',' ','#',' ','#',' ','#',' ','#',' ','#','#','#','#','#','#',' ',' ',' ',' ',' ','#',' ',' ',' ',' ',' ','#',' ',' ',' ','#',' ','#',' ',' ','#'},
{'#',' ','#',' ','#','#',' ','#',' ','#',' ',' ',' ',' ',' ',' ',' ',' ','#',' ','#','#','#',' ','#','#','#','#','#','#','#','#','#',' ','#',' ','#','#',' ','#'},
{'#',' ','#',' ','#',' ',' ','#',' ','#','#','#','#',' ','#','#','#',' ','#',' ',' ',' ',' ',' ',' ',' ','#',' ',' ',' ',' ',' ',' ',' ',' ',' ',' ',' ',' ','#'},
{'#',' ','#',' ','#','#',' ','#',' ','#',' ',' ',' ',' ','#',' ',' ',' ','#','#','#','#','#',' ','#',' ','#',' ','#',' ','#','#',' ','#','#',' ','#',' ','#','#'},
{'#',' ','#',' ',' ',' ',' ','#',' ','#','#','#','#',' ','#','#','#','#','#',' ',' ',' ','#',' ','#',' ','#',' ','#',' ','#','#',' ',' ','#',' ','#',' ',' ','#'},
{'#',' ','#','#','#','#',' ','#',' ',' ',' ',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ',' ','#',' ','#',' ',' ','#',' ','#','#','#','#','#',' ','#'},
{'#',' ',' ',' ',' ','#',' ','#',' ','#','#','#','#',' ','#',' ','#',' ','#',' ','#',' ','#','#','#',' ','#',' ','#','#','#','#',' ',' ',' ','#',' ','#',' ','#'},
{'#',' ','#','#',' ',' ',' ','#',' ',' ',' ',' ','#',' ','#',' ','#',' ','#',' ',' ',' ',' ',' ','#',' ','#',' ',' ',' ',' ','#',' ','#',' ','#',' ',' ',' ','#'},
{'#',' ','#',' ',' ','#',' ','#',' ','#','#',' ','#',' ','#',' ','#',' ','#','#','#','#','#',' ','#',' ','#','#','#','#',' ','#',' ','#',' ','#','#','#','#','#'},
{'#',' ','#',' ','#','#',' ','#',' ','#',' ',' ',' ',' ','#',' ','#',' ',' ','#',' ',' ',' ',' ','#',' ',' ',' ',' ',' #',' ','#',' ','#',' ',' ',' ','#',' ','#'},
{'#',' ','#',' ','#',' ',' ','#',' ','#','#','#','#','#','#',' ','#',' ','#','#',' ','#','#','#','#','#','#','#',' ','#',' ','#',' ',' ',' ','#',' ','#',' ','#'},
{'#',' ','#',' ','#',' ','#','#',' ','#',' ',' ',' ',' ',' ',' ','#',' ',' ','#',' ',' ',' ',' ',' ',' ',' ','#',' ','#',' ','#','#','#','#','#',' ','#',' ','#'},
{'#',' ','#',' ','#',' ',' ','#',' ','#',' ','#','#','#','#','#','#','#',' ','#',' ','#','#','#','#','#',' ','#',' ','#',' ',' ',' ','#',' ','#',' ',' ',' ','#'},
{'#',' ','#','#','#','#','#','#',' ','#',' ',' ',' ',' ',' ',' ',' ','#',' ','#',' ','#',' ',' ',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#','#','#','#','#'},
{'#',' ',' ',' ',' ','#',' ',' ',' ','#',' ','#',' ','#','#','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ',' ','#',' ','#',' ','#',' ',' ',' ',' ',' ','#'},
{'#',' ','#','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#','#','#','#','#',' ',' ',' ','#',' ','#','#','#',' ','#'},
{'#',' ',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ',' ',' ',' ','#','#','#',' ','#',' ','#',' ','#',' ','#'},
{'#',' ','#','#',' ','#',' ','#','#','#',' ','#','#','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#','#','#',' ','#',' ',' ',' ','#',' ','#',' ','#',' ','#'},
{'#',' ',' ',' ',' ','#',' ',' ',' ',' ',' ',' ',' ',' ',' ','#',' ',' ',' ',' ',' ',' ',' ','#',' ',' ',' ','#',' ','#',' ','#',' ',' ',' ',' ',' ','#',' ',' '},
{'#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#'},
};
struct Player player;
player.sprite ='@';
player.x = 0;
player.y = 1;
string prevmove1 = "NA";
string prevmove2 = "NA";
while(hasntWon){
if (maze[player.y][player.x] == ' '){
maze[player.y][player.x] = player.sprite;
}
for (y=0;y
cout <
for (x = 0; x
cout << maze[y][x];
}
}
char key = getch();
switch(key){
case KEY_LEFT :
if (maze[player.y][player.x-1] != '#'){
maze[player.y][player.x] = ' ';
player.x--;
}
break;
case KEY_UP :
if (maze[player.y-1][player.x] != '#'){
maze[player.y][player.x] = ' ';
player.y--;
}
break;
case KEY_DOWN :
if (maze[player.y+1][player.x] != '#'){
maze[player.y][player.x] = ' ';
player.y++;
}
break;
case KEY_RIGHT :
if (maze[player.y][player.x+1] != '#'){
maze[player.y][player.x] = ' ';
player.x++;
}
break;
case 'q':
exit(0);
break;
}
bool once1 = true;
bool once2 = true;
bool once3 = true;
bool once4 = true;
if (player.x == WIDTH -1){
cout <
system("PAUSE");
hasntWon = false;
}
system("CLS");
}
}
#include
#include
#include
#include
#include
#include
using namespace std;
const int KEY_LEFT = 'a', KEY_RIGHT = 'd',KEY_UP = 'w', KEY_DOWN ='s';
const int HEIGHT =25, WIDTH = 40;
bool hasntWon = true;
int hP = 100;
enum DirectionX {Right,Left};
enum DirectionY {Up,Down};
struct Player {
char sprite;
int x;
int y;
};
struct Enemy{
char sprite;
int x;
int y;
DirectionX pastDirX;
DirectionY pastDirY;
};
unsigned char MoveEnemy(Enemy& enemy, unsigned char maze[HEIGHT][WIDTH], int i , int j , string& smove, bool& nc){
if (nc){
bool psbUp = false;
bool psbDown = false;
bool psbRight = false;
bool psbLeft = false;
if (maze[enemy.y][enemy.x+1] != '#' ){
psbRight = true;
}
if (maze[enemy.y][enemy.x-1] != '#' ){
psbLeft = true;
}
if (maze[enemy.y+1][enemy.x] != '#' ){
psbLeft = true;
}
if (maze[enemy.y-1][enemy.x] != '#') {
psbLeft = true;
}
enum Mv {up,right,down,left,NA};
Mv move = NA;
if (smove =="up"){
move = up;
}
else if (smove =="right"){
move = right;
}
else if (smove =="down"){
move = down;
}
else if (smove =="left"){
move = left;
}
else {
move = NA;
}
if (enemy.pastDirX == Right && !psbRight){
if(enemy.pastDirY == Up){
if (psbUp){
move = up;
}
else if (psbDown && move != up){
move = down;
}
else if (psbLeft){
move = left;
}
else {
move = down;
}
}
else{
if(psbDown){
move = down ;
}
else if(psbUp && move != down){
move = up;
}
else if (psbLeft){
move = left;
}
else{
move = up;
}
}
}
else if (enemy.pastDirX == Right && psbRight){
move = right;
}
else if (enemy.pastDirX == Left && !psbLeft){
if(enemy.pastDirY == Up) {
if (psbUp){
move = up;
}
else if(psbDown && move != up){
move = down;
}
else if (psbRight){
move = right;
}
else {
move = down;
}
}
else {
if(psbDown){
move = down;
}
else if (psbUp && move != down){
move = up;
}
else if (psbRight){
move = right;
}
else {
move = up;
}
}
}
else if (enemy.pastDirX == Left && psbLeft){
move = left;
}
else if(enemy.pastDirY == Up && !psbUp){
if (enemy.pastDirX == Right){
if (psbRight){
move = right;
}
else if (psbLeft && move != right){
move = left;
}
else if (psbDown){
move = down;
}
else {
move = left;
}
}
else {
if (psbLeft ){
move = left;
}
else if (psbRight && move != left){
move = right;
}
else if (psbDown){
move = down;
}
else {
move = right;
}
}
}
else if (enemy.pastDirY == Up && psbUp){
move = up;
}
else if (enemy.pastDirY == Down && !psbDown){
if (enemy.pastDirX == Right){
if (psbRight){
move = right;
}
else if (psbLeft && move != right){
move = left;
}
else if (psbUp){
move = up;
}
else {
move = left;
}
}
else {
if (psbLeft){
move = left;
}
else if (psbRight && move != left ){
move = right ;
}
else if (psbRight){
move = up;
}
else {
move = right ;
}
}
}
else if (enemy.pastDirY == Down && psbDown){
move = down;
}
switch (move) {
case up:
maze[enemy.y][enemy.x] = ' ';
enemy.pastDirY = Up;
smove = "up";
enemy.y--;
break ;
case right:
maze[enemy.y][enemy.x] = ' ';
enemy.pastDirX = Right;
smove = "right";
enemy.x++;
break ;
case down:
maze[enemy.y][enemy.x] = ' ';
enemy.pastDirY = Down;
smove = "down";
enemy.y++;
break ;
case left:
maze[enemy.y][enemy.x] = ' ';
enemy.pastDirX = Left;
smove = "left";
enemy.x--;
break ;
case NA:
smove = "NA";
break;
}
}
nc = false;
return maze[i][j];
}
int main()
{
int x,y,h,i,j;
srand(time(NULL));
unsigned char maze [HEIGHT][WIDTH]={{'#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#'},
{' ',' ',' ',' ',' ',' ',' ','#',' ','#',' ',' ',' ','#','#',' ','#',' ',' ',' ',' ',' ','#',' ',' ',' ','#',' ',' ',' ',' ',' ',' ',' ','#',' ',' ',' ',' ','#'},
{'#','#','#','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ','#',' ','#','#','#',' ','#','#','#',' ','#',' ','#','#','#',' ','#',' ','#',' ','#','#',' ','#'},
{'#',' ',' ',' ',' ','#',' ',' ',' ','#',' ','#',' ','#',' ','#','#',' ',' ',' ','#',' ',' ',' ',' ',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ','#'},
{'#',' ','#','#',' ','#','#','#',' ','#',' ','#',' ','#',' ',' ',' ',' ','#',' ','#','#','#','#','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ','#'},
{'#',' ','#',' ',' ','#',' ','#',' ','#',' ','#',' ','#','#','#','#','#','#',' ',' ',' ',' ',' ','#',' ',' ',' ',' ',' ','#',' ',' ',' ','#',' ','#',' ',' ','#'},
{'#',' ','#',' ','#','#',' ','#',' ','#',' ',' ',' ',' ',' ',' ',' ',' ','#',' ','#','#','#',' ','#','#','#','#','#','#','#','#','#',' ','#',' ','#','#',' ','#'},
{'#',' ','#',' ','#',' ',' ','#',' ','#','#','#','#',' ','#','#','#',' ','#',' ',' ',' ',' ',' ',' ',' ','#',' ',' ',' ',' ',' ',' ',' ',' ',' ',' ',' ',' ','#'},
{'#',' ','#',' ','#','#',' ','#',' ','#',' ',' ',' ',' ','#',' ',' ',' ','#','#','#','#','#',' ','#',' ','#',' ','#',' ','#','#',' ','#','#',' ','#',' ','#','#'},
{'#',' ','#',' ',' ',' ',' ','#',' ','#','#','#','#',' ','#','#','#','#','#',' ',' ',' ','#',' ','#',' ','#',' ','#',' ','#','#',' ',' ','#',' ','#',' ',' ','#'},
{'#',' ','#','#','#','#',' ','#',' ',' ',' ',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ',' ','#',' ','#',' ',' ','#',' ','#','#','#','#','#',' ','#'},
{'#',' ',' ',' ',' ','#',' ','#',' ','#','#','#','#',' ','#',' ','#',' ','#',' ','#',' ','#','#','#',' ','#',' ','#','#','#','#',' ',' ',' ','#',' ','#',' ','#'},
{'#',' ','#','#',' ',' ',' ','#',' ',' ',' ',' ','#',' ','#',' ','#',' ','#',' ',' ',' ',' ',' ','#',' ','#',' ',' ',' ',' ','#',' ','#',' ','#',' ',' ',' ','#'},
{'#',' ','#',' ',' ','#',' ','#',' ','#','#',' ','#',' ','#',' ','#',' ','#','#','#','#','#',' ','#',' ','#','#','#','#',' ','#',' ','#',' ','#','#','#','#','#'},
{'#',' ','#',' ','#','#',' ','#',' ','#',' ',' ',' ',' ','#',' ','#',' ',' ','#',' ',' ',' ',' ','#',' ',' ',' ',' ',' #',' ','#',' ','#',' ',' ',' ','#',' ','#'},
{'#',' ','#',' ','#',' ',' ','#',' ','#','#','#','#','#','#',' ','#',' ','#','#',' ','#','#','#','#','#','#','#',' ','#',' ','#',' ',' ',' ','#',' ','#',' ','#'},
{'#',' ','#',' ','#',' ','#','#',' ','#',' ',' ',' ',' ',' ',' ','#',' ',' ','#',' ',' ',' ',' ',' ',' ',' ','#',' ','#',' ','#','#','#','#','#',' ','#',' ','#'},
{'#',' ','#',' ','#',' ',' ','#',' ','#',' ','#','#','#','#','#','#','#',' ','#',' ','#','#','#','#','#',' ','#',' ','#',' ',' ',' ','#',' ','#',' ',' ',' ','#'},
{'#',' ','#','#','#','#','#','#',' ','#',' ',' ',' ',' ',' ',' ',' ','#',' ','#',' ','#',' ',' ',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#','#','#','#','#'},
{'#',' ',' ',' ',' ','#',' ',' ',' ','#',' ','#',' ','#','#','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ',' ','#',' ','#',' ','#',' ',' ',' ',' ',' ','#'},
{'#',' ','#','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#','#','#','#','#',' ',' ',' ','#',' ','#','#','#',' ','#'},
{'#',' ',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ',' ','#',' ','#',' ','#',' ','#',' ','#',' ',' ',' ',' ',' ','#','#','#',' ','#',' ','#',' ','#',' ','#'},
{'#',' ','#','#',' ','#',' ','#','#','#',' ','#','#','#',' ','#',' ','#',' ','#',' ','#',' ','#',' ','#','#','#',' ','#',' ',' ',' ','#',' ','#',' ','#',' ','#'},
{'#',' ',' ',' ',' ','#',' ',' ',' ',' ',' ',' ',' ',' ',' ','#',' ',' ',' ',' ',' ',' ',' ','#',' ',' ',' ','#',' ','#',' ','#',' ',' ',' ',' ',' ','#',' ',' '},
{'#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#','#'},
};
struct Player player;
player.sprite ='@';
player.x = 0;
player.y = 1;
string prevmove1 = "NA";
string prevmove2 = "NA";
while(hasntWon){
if (maze[player.y][player.x] == ' '){
maze[player.y][player.x] = player.sprite;
}
for (y=0;y
cout <
for (x = 0; x
cout << maze[y][x];
}
}
char key = getch();
switch(key){
case KEY_LEFT :
if (maze[player.y][player.x-1] != '#'){
maze[player.y][player.x] = ' ';
player.x--;
}
break;
case KEY_UP :
if (maze[player.y-1][player.x] != '#'){
maze[player.y][player.x] = ' ';
player.y--;
}
break;
case KEY_DOWN :
if (maze[player.y+1][player.x] != '#'){
maze[player.y][player.x] = ' ';
player.y++;
}
break;
case KEY_RIGHT :
if (maze[player.y][player.x+1] != '#'){
maze[player.y][player.x] = ' ';
player.x++;
}
break;
case 'q':
exit(0);
break;
}
bool once1 = true;
bool once2 = true;
bool once3 = true;
bool once4 = true;
if (player.x == WIDTH -1){
cout <
system("PAUSE");
hasntWon = false;
}
system("CLS");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
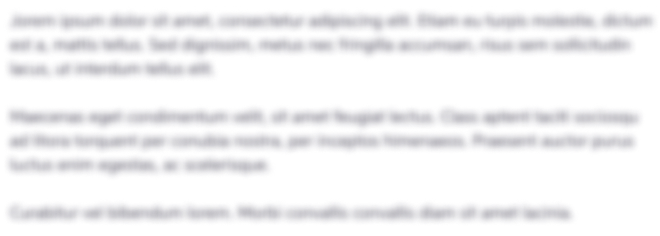
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started