Question
This is the HW guide: A Classy Account We will use classes to represent bank accounts and money respectively, and write functions that allow us
This is the HW guide:
A Classy Account
We will use classes to represent bank accounts and money respectively, and write functions that allow us to interact with our accounts. Requirements:
You will write your code where prompted to do so, and the main() and testing functions are not to be altered Create two classes and name their types Account and Money Use initialization sections where possible for all constructors With the sole exceptions being the print() functions, no other functions should be printing to the screen unless an error message must be displayed Money Class Requirements Negative amounts of Money shall be stored by making both the dollars and cents negative The Money class shall have 4 constructors * A default constructor that initializes your Money object to $0.00 * A constructor that takes two integers, the first for the dollars and the second for the cents * A constructor that takes one integer, for a dollar amount and no cents * A constructor that takes one double, and can assign dollars and cents accordingly bool isNegative() const * Returns true if your amount of money is negative void add(const Money &m) * The sum shall be stored in the calling object void subtract(const Money &m) * The difference shall be stored in the calling object bool isEqual(const Money &m) const void print() const * This function prints the amount of Money you have; it must print a $, and always show two decimal places * Negative amounts of Money shall be represented in the following manner: ($X.XX) The Money class shall have two private data members AND one helper function * An integer that represents how many dollars you have. * An integer that represents how many cents you have. 1 * A function getPennies() that returns the equivalent amount of pennies for the calling object Account Class Requirements The Account class shall have 4 constructors * A default constructor that initializes your Account object with a name Savings, an interest rate of 0.00%, and an initial balance of $0.00 * A constructor that takes a string, double, and Money object to initialize your Accounts name, interest rate, and balance respectively * A constructor that takes a string, double, and an integer that represents your initial balance (a clean dollar amount) * A constructor that takes a string, double, and a double that represents your initial balance std::string getName() const double getRate() const const Money getBalance() const void setName(std::string newName) void deposit(const Money &m) void deposit(int d, int c) void deposit(int d) void deposit(double d) * For all versions of the deposit function, the function shall not accept negative amounts of money * For all versions of the deposit function, the balance of the account shall be updated accordingly const Money withdraw(const Money &m) const Money withdraw(int d, int c) const Money withdraw(int d) const Money withdraw(double d) * For all versions of the withdraw function, the function shall not accept negative amounts of money * For all versions of the withdraw function, the user shall not overdraw by more than $50.00 * For all versions of the withdraw function, the balance of the account shall be updated accordingly void accrue() * The accrued interest shall be calculated and added to the balance void print() const * This function shall only print the balance; formatting requirements are identical to that of the Money class * This function prints the amount of Money you have; it must print a $, and always show two decimal places * Negative amounts of Money shall be represented in the following manner: ($X.XX) 2 void transferTo(Account &dest, const Money &amount) * The function shall not transfer negative amounts of money * The balances of both accounts shall be updated accordingly The Account class shall have 3 private data members * A Money object that will represent how much money is in the account * A double to represent the interest rate as a decimal value * A string object to represent the name of the account. (Checking, Savings, CD, etc.) Define the following non-member function: const Account createAccount(); There are minor changes to this function over the previous assignment It will prompt the user for the account name, interest rate, and starting balance in that order The prompts shall appear as follows: Let's set up your account. First, what's the name of the account? What is the interest rate of your [NAME] account? Finally, what is the starting balance of your [NAME] account? You may NOT assume that the name of the account contains only a single word You may assume the user will always type an interest rate as a decimal value (e.g. 0.05) The user is allowed to type their amount of money with or without a $ A sample run of your program shall look like this: $ ./hw02 < inputs *** TESTING MONEY CLASS FUNCTIONS *** 3. Testing Money class IsNegative:.......................1 4. Testing Money class IsNegative:.......................1 5. Testing Money class Add:..............................1 6. Testing Money class Subtract:.........................1 7. Testing Money class Subtract:.........................1 8. Testing Money class Print ( Must match $5.44 ):.......$5.44 9. Testing Money class Print ( Must match ($5.44) ):.....($5.44) *** TESTING ACCOUNT CLASS FUNCTIONS *** 1. Testing Account class GetName:.......................1 2. Testing Account class GetRate:.......................1 3. Testing Account class GetBalance:....................1 4. Testing Account class SetName:.......................1 5. Testing Account class Deposit #1:....................1 6. Testing Account class Deposit #2:....................1 7. Testing Account class Deposit #3:....................1 8. Testing Account class Deposit #4:....................1 9. Testing Account class Deposit (ERROR):...............Cannot deposit negative amount. 10. Testing Account class Withdraw #1:...................1 11. Testing Account class Withdraw #2:...................1 12. Testing Account class Withdraw #3:...................1 13. Testing Account class Withdraw #4:...................1 14. Testing Account class Withdraw (OVERDRAW):...........1 15. Testing Account class Withdraw (PARTIAL):............1 3 16. Testing Account class Withdraw (ERROR):..............Cannot withdraw negative amount. 17. Testing Account class Accrue: .......................1 18. Testing Account class Transfer: .....................1 19. Testing Account class Print ( Must match $50.00 ):...$50.00 20. Testing Account class Print ( Must match ($50.00) ):.($50.00) Let's set up your account. First, what's the name of the account? What is the interest rate of your Roth IRA account? Finally, what is the starting balance of your Roth IRA account? *** TESTING NON-CLASS FUNCTION CREATEACCOUNT *** 1. Account Name:...Mutual Fund 2. Interest Rate:..0.05 3. Balance:........$2500.00 $ Hints: For the overloaded deposit and withdraw functions, you do not need to re-write the function bodies multiple times The hints from the previous homework also apply here and are repeated You are free to write any extra helper functions that might make your life easier The functions listed in the Requirements are required (shocker!), but you may find it useful to write other helper functions Converting a double to a Money object can cause rounding errors * You may want to look up the round() function Converting an amount of money to an equivalent amount of pennies makes a lot of logical work go away You can create a file that holds user inputs and use it to streamline your testing. http://linuxcommand.org/lc3_lts0070.php Reminders: Be sure to include a comment block at the top of the file with the required information Refer to the General Homework Requirements handout on Blackboard Provide meaningful comments If you think a comment is redundant, it probably is If you think a comment is helpful, it probably is Remember that you are writing comments for other programmers, not people who know nothing (obligatory Jon Snow) about coding There will be no extensions 4
And here below the starting code:
/*
* Replace
* With
* Comment
* Block
* *<|8^)
*/
// Add Or Subtract Libraries As Needed
#include
#include
#include
#include
// Place Money Class Definition Here
// Place Account Class Definition Here
// Define Non-Member Function Here
// Test Functions; *** DO NOT ALTER ***
bool moneyIsNegative(bool expected);
bool moneyAdd();
bool moneySubtractPos();
bool moneySubtractNeg();
void moneyPrint(double arg);
bool acctGetName();
bool acctGetRate();
bool acctGetBalance();
bool acctSetName();
bool acctDep01();
bool acctDep02();
bool acctDep03();
bool acctDep04();
void acctBadDep();
bool acctWith01();
bool acctWith02();
bool acctWith03();
bool acctWith04();
bool acctWithPart01();
bool acctWithPart02();
void acctBadWith();
bool acctAccrue();
void acctPrint(double arg);
bool acctTransfer();
// Main Function; *** DO NOT ALTER ***
int main()
{
char prev = std::cout.fill('.');
std::cout << "*** TESTING MONEY CLASS FUNCTIONS *** ";
std::cout << std::setw(57) << std::left << "3. Testing Money class IsNegative:" << (moneyIsNegative(true)) << std::endl;
std::cout << std::setw(57) << std::left << "4. Testing Money class IsNegative:" << (moneyIsNegative(false)) << std::endl;
std::cout << std::setw(57) << std::left << "5. Testing Money class Add:" << (moneyAdd()) << std::endl;
std::cout << std::setw(57) << std::left << "6. Testing Money class Subtract:" << (moneySubtractPos()) << std::endl;
std::cout << std::setw(57) << std::left << "7. Testing Money class Subtract:" << (moneySubtractNeg()) << std::endl;
std::cout << std::setw(57) << std::left << "8. Testing Money class Print ( Must match $5.44 ):"; (moneyPrint(5.44)); std::cout << std::endl;
std::cout << std::setw(57) << std::left << "9. Testing Money class Print ( Must match ($5.44) ):"; (moneyPrint(-5.44)); std::cout << std::endl;
std::cout << " *** TESTING ACCOUNT CLASS FUNCTIONS *** ";
std::cout << std::setw(4) << std::left << std::setfill(' ') << "1. " << std::setw(53) << std::left << std::setfill('.') << "Testing Account class GetName:" << (acctGetName()) << std::endl;
std::cout << std::setw(4) << std::left << std::setfill(' ') << "2. " << std::setw(53) << std::left << std::setfill('.') << "Testing Account class GetRate:" << (acctGetRate()) << std::endl;
std::cout << std::setw(4) << std::left << std::setfill(' ') << "3. " << std::setw(53) << std::left << std::setfill('.') << "Testing Account class GetBalance:" << (acctGetBalance()) << std::endl;
std::cout << std::setw(4) << std::left << std::setfill(' ') << "4. " << std::setw(53) << std::left << std::setfill('.') << "Testing Account class SetName:" << (acctSetName()) << std::endl;
std::cout << std::setw(4) << std::left << std::setfill(' ') << "5. " << std::setw(53) << std::left << std::setfill('.') << "Testing Account class Deposit #1:" << (acctDep01()) << std::endl;
std::cout << std::setw(4) << std::left << std::setfill(' ') << "6. " << std::setw(53) << std::left << std::setfill('.') << "Testing Account class Deposit #2:" << (acctDep02()) << std::endl;
std::cout << std::setw(4) << std::left << std::setfill(' ') << "7. " << std::setw(53) << std::left << std::setfill('.') << "Testing Account class Deposit #3:" << (acctDep03()) << std::endl;
std::cout << std::setw(4) << std::left << std::setfill(' ') << "8. " << std::setw(53) << std::left << std::setfill('.') << "Testing Account class Deposit #4:" << (acctDep04()) << std::endl;
std::cout << std::setw(4) << std::left << std::setfill(' ') << "9. " << std::setw(53) << std::left << std::setfill('.') << "Testing Account class Deposit (ERROR):"; acctBadDep();
std::cout << std::setw(4) << std::left << "10. " << std::setw(53) << std::left << "Testing Account class Withdraw #1:" << (acctWith01()) << std::endl;
std::cout << std::setw(4) << std::left << "11. " << std::setw(53) << std::left << "Testing Account class Withdraw #2:" << (acctWith02()) << std::endl;
std::cout << std::setw(4) << std::left << "12. " << std::setw(53) << std::left << "Testing Account class Withdraw #3:" << (acctWith03()) << std::endl;
std::cout << std::setw(4) << std::left << "13. " << std::setw(53) << std::left << "Testing Account class Withdraw #4:" << (acctWith04()) << std::endl;
std::cout << std::setw(4) << std::left << "14. " << std::setw(53) << std::left << "Testing Account class Withdraw (OVERDRAW):" << (acctWithPart01()) << std::endl;
std::cout << std::setw(4) << std::left << "15. " << std::setw(53) << std::left << "Testing Account class Withdraw (PARTIAL):" << (acctWithPart02()) << std::endl;
std::cout << std::setw(4) << std::left << "16. " << std::setw(53) << std::left << "Testing Account class Withdraw (ERROR):"; acctBadWith();
std::cout << std::setw(4) << std::left << "17. " << std::setw(53) << std::left << "Testing Account class Accrue: " << (acctAccrue()) << std::endl;
std::cout << std::setw(4) << std::left << "18. " << std::setw(53) << std::left << "Testing Account class Transfer: " << (acctTransfer()) << std::endl;
std::cout << std::setw(4) << std::left << "19. " << std::setw(53) << std::left << "Testing Account class Print ( Must match $50.00 ):"; (acctPrint(50.00)); std::cout << std::endl;
std::cout << std::setw(4) << std::left << "20. " << std::setw(53) << std::left << "Testing Account class Print ( Must match ($50.00) ):"; (acctPrint(-50.00)); std::cout << std::endl;
Account account = createAccount();
std::cout << " *** TESTING NON-CLASS FUNCTION CREATEACCOUNT *** ";
std::cout << std::setw(19) << std::left << "1. Account Name:" << account.getName() << std::endl;
std::cout << std::setw(19) << std::left << "2. Interest Rate:" << account.getRate() << std::endl;
std::cout << std::setw(19) << std::left << "3. Balance:"; (account.getBalance()).print(); std::cout << std::endl;
std::cout.fill(prev);
return 0;
}
// Implement Money Class Functions Here
// Implement Account Class Functions Here
// Implement Non-Member Function Here
// Test Functions; *** DO NOT ALTER ***
bool moneyGetPennies(int arg)
{
Money money(arg / 100, arg % 100);
return money.getPennies() == arg;
}
bool moneyIsNegative(bool expected)
{
if (expected == true) {
Money money1(-5, 55);
Money money2(5, -55);
Money money3(-5);
Money money4(-5.55);
return ( ( money1.isNegative() &&
money2.isNegative() &&
money3.isNegative() &&
money4.isNegative() ) == expected );
} else {
Money money1(5, 55);
Money money2(5);
Money money3(5.55);
Money money4;
return ( !( !(money1.isNegative()) &&
!(money2.isNegative()) &&
!(money3.isNegative()) &&
!(money4.isNegative()) ) == expected );
}
}
void moneyPrint(double arg)
{
Money money(arg);
money.print();
}
bool moneyAdd()
{
Money left(4, 64), right(5, 36);
left.add(right);
return left.isEqual(10);
}
bool moneySubtractPos()
{
Money money(20);
money.subtract(10.11);
return money.isEqual(9.89);
}
bool moneySubtractNeg()
{
Money money(20);
money.subtract(30.11);
return money.isEqual(-10.11);
}
bool acctGetName()
{
Account account;
return (account.getName() == "Savings");
}
bool acctGetRate()
{
Account account("Savings", 0.01, Money(5, 0));
return (account.getRate() == 0.01);
}
void acctPrint(double arg)
{
Account account("Savings", 0.015, arg);
account.print();
}
bool acctGetBalance()
{
Money money(50);
Account account("Savings", 0.01, money);
Money bal = account.getBalance();
return (bal.isEqual(money));
}
bool acctSetName()
{
std::string name = "ROTH IRA";
Account account("Savings", 0.01, 5);
account.setName(name);
return (name == account.getName());
}
bool acctDep01()
{
Money money(5.63);
Account account;
account.deposit(money);
return ((account.getBalance()).isEqual(money));
}
bool acctDep02()
{
Account account;
account.deposit(5, 63);
return ((account.getBalance()).isEqual(5.63));
}
bool acctDep03()
{
Account account;
account.deposit(20);
return ((account.getBalance()).isEqual(20));
}
bool acctDep04()
{
Account account;
account.deposit(5.63);
return ((account.getBalance()).isEqual(5.63));
}
void acctBadDep()
{
Account account;
account.deposit(-50);
}
bool acctWith01()
{
Money money(25.44);
Account account("Savings", 0.015, 50);
Money w = account.withdraw(money);
return (w.isEqual(money) && (account.getBalance()).isEqual(24.56));
}
bool acctWith02()
{
Account account("Savings", 0.015, 50);
Money w = account.withdraw(25, 44);
return (w.isEqual(25.44) && (account.getBalance()).isEqual(24.56));
}
bool acctWith03()
{
Account account("Savings", 0.015, 50);
Money w = account.withdraw(24);
return (w.isEqual(24) && (account.getBalance()).isEqual(26));
}
bool acctWith04()
{
Account account("Savings", 0.015, 50);
Money w = account.withdraw(25.44);
return (w.isEqual(25.44) && (account.getBalance()).isEqual(24.56));
}
bool acctWithPart01()
{
Account account("Checking", 0.015, 54.33);
Money w = account.withdraw(60);
return w.isEqual(60) && (account.getBalance()).isEqual(-5.67);
}
bool acctWithPart02()
{
Account account("Checking", 0.015, 54.33);
Money w = account.withdraw(300);
return w.isEqual(104.33) && (account.getBalance()).isEqual(-50);
}
void acctBadWith()
{
Account account;
account.withdraw(-10);
}
bool acctAccrue()
{
Account account("Roth", 0.07, 1000);
account.accrue();
return (account.getBalance()).isEqual(1070);
}
bool acctTransfer()
{
Account savings;
Account checking("Checking", 0.015, 2500);
checking.transferTo(savings, 500);
return (savings.getBalance()).isEqual(500) && (checking.getBalance()).isEqual(2000);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
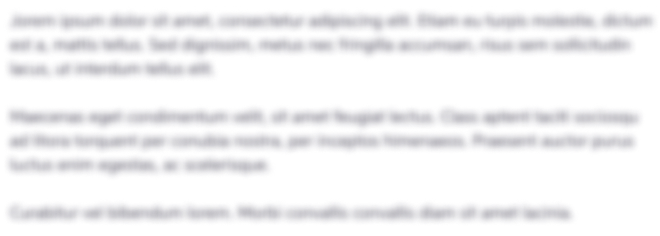
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started