Question
This is the solution to the question of branch a in the picture. I want to solve branch b, please /* * Click nbfs:/bhost/SystemFileSystem/Templates/Licenses/license-default.txt to
This is the solution to the question of branch a in the picture. I want to solve branch b, please /* * Click nbfs:/bhost/SystemFileSystem/Templates/Licenses/license-default.txt to change this license * Click nbfs:/bhost/SystemFileSystem/Templates/Classes/Class.java to edit this template */ package com.mycompany.project;
/** * * */ class Delivery extends PizzaOrder { private double tripRate; private int zone;
public Delivery() { }
public Delivery(String customerName, int pizzaSize, int numberOfToppings, double toppingPrice, double tripRate, int zone) { super(customerName, pizzaSize, numberOfToppings, toppingPrice); this.tripRate = tripRate; this.zone = zone; }
public double getTripRate() { return tripRate; }
/* * Click nbfs:/bhost/SystemFileSystem/Templates/Licenses/license-default.txt to change this license * Click nbfs:/bhost/SystemFileSystem/Templates/Classes/Class.java to edit this template */
package com.mycompany.project;
/** * * * */
import java.util.ArrayList; import java.util.Collections; class Driver { public static void main(String[] args) { ArrayList
sortOrders(orders); printSortedOrders(orders); System.out.println("Total sum all order prices: " + calculateTotalOrdersPrice(orders)); System.out.println("Report second Delivery order: " + orders.get(1)); }
public static void printSortedOrders(ArrayList
public static void sortOrders(ArrayList
public static double calculateTotalOrdersPrice(ArrayList
public void setTripRate(double tripRate) { this.tripRate = tripRate; }
public int getZone() { return zone; }
public void setZone(int zone) { this.zone = zone; }
@Override public double calculateOrderPrice() { return (super.calculateOrderPrice() + (tripRate/100 * super.calculateOrderPrice() * zone)); }
@Override public String toString() { return "Delivery[" +"customerName= " + super.getCustomerName() + " "+" tripRate=" + tripRate +" zone=" + zone +"]"; } }
/* * Click nbfs:/bhost/SystemFileSystem/Templates/Licenses/license-default.txt to change this license * Click nbfs:/bhost/SystemFileSystem/Templates/Classes/Class.java to edit this template */ package com.mycompany.project;
/** * * */
import java.util.Date;
class PizzaOrder implements Comparable
/* * Click nbfs:/bhost/SystemFileSystem/Templates/Licenses/license-default.txt to change this license * Click nbfs:/bhost/SystemFileSystem/Templates/Classes/Class.java to edit this template */ package com.mycompany.project;
/** * * */ class Seated extends PizzaOrder { private double serviceCharge; private int numberOfPeople;
public Seated() { }
public Seated(String customerName, int pizzaSize, int numberOfToppings, double toppingPrice, double serviceCharge, int numberOfPeople) { super(customerName, pizzaSize, numberOfToppings, toppingPrice); this.serviceCharge = serviceCharge; this.numberOfPeople = numberOfPeople; }
public double getServiceCharge() { return serviceCharge; }
public void setServiceCharge(double serviceCharge) { this.serviceCharge = serviceCharge; }
public int getNumberOfPeople() { return numberOfPeople; }
public void setNumberOfPeople(int numberOfPeople) { this.numberOfPeople = numberOfPeople; }
@Override public double calculateOrderPrice() { return (super.calculateOrderPrice() + (serviceCharge * numberOfPeople)); }
@Override public String toString() { return "Seated[" +"customerName= " + super.getCustomerName() + " " +" serviceCharge=" + serviceCharge +" numberOfPeople=" + numberOfPeople + "["; } }
public PizzaOrder() { }
public PizzaOrder(String customerName, int pizzaSize, int numberOfToppings, double toppingPrice) { this.customerName = customerName; this.pizzaSize = pizzaSize; this.numberOfToppings = numberOfToppings; this.toppingPrice = toppingPrice; }
public String getCustomerName() { return customerName; }
public void setCustomerName(String customerName) { this.customerName = customerName; }
public Date getDateOrdered() { return dateOrdered; }
public void setDateOrdered(Date dateOrdered) { this.dateOrdered = dateOrdered; }
public int getPizzaSize() { return pizzaSize; }
public void setPizzaSize(int pizzaSize) { this.pizzaSize = pizzaSize; }
public int getNumberOfToppings() { return numberOfToppings; }
public void setNumberOfToppings(int numberOfToppings) { this.numberOfToppings = numberOfToppings; }
public double getToppingPrice() { return toppingPrice; }
public void setToppingPrice(double toppingPrice) { this.toppingPrice = toppingPrice; }
public double calculateOrderPrice() { return (numberOfToppings * toppingPrice) * pizzaSize; }
public void printOrderInfo() { System.out.println(customerName + ": " + calculateOrderPrice()); }
@Override public int compareTo(PizzaOrder o) { return Double.compare(calculateOrderPrice(), o.calculateOrderPrice()); }
@Override public String toString() { return "PizzaOrder[" +"customerName= " + customerName + " "+" dateOrdered= " + dateOrdered +" pizzaSize=" + pizzaSize +", numberOfToppings=" + numberOfToppings +" toppingPrice=" + toppingPrice +"]"; } }
/* * Click nbfs:/bhost/SystemFileSystem/Templates/Licenses/license-default.txt to change this license * Click nbfs:/bhost/SystemFileSystem/Templates/Classes/Class.java to edit this template */ package com.mycompany.project;
/** * * */ class ToGo extends PizzaOrder { public ToGo() { }
public ToGo(String customerName, int pizzaSize, int numberOfToppings, double toppingPrice) { super(customerName, pizzaSize, numberOfToppings, toppingPrice); }
@Override public String toString() { return "ToGo[" +"customerName= " + super.getCustomerName()+"]" ; } }
Write a complete Java program that creates the following classes: For this assignment, you will use the same classes you created in Project phase I 1- class PizzaOrder which implements the Comparable interface and contains the ( PizzaOrder, Delivery, ToGo, and Seated) and build a javafx GUI to provide input and following attributes and member methods: output as follows: - customerName ( String) Your Driver class should first build and display a GUl containing the following items: - dateOrdered (Date) - customerName ( String) - default empty - pizzaSize (final static int SMALL=1, MEDIUM=2, LARGE=3) - orderType (ToGo, Delivery, or Seated) - default ToGo - numberofToppings ( int) - pizzaSize (int 1 = SMALL, 2= MEDIUM, and 3 = LARGE) default 1 (SMALL) - toppingPrice (double) - List of Toppings ( at least three: Onions, Olives, and Green Peppers ) - - Appropriate constructors ( default and non default) as well as the default none appropriate setter and getter methods. - toppingPrice (double) - default (10) - A tostring() method. - orderPrice (double) - default (0.0) - Method calculateOrderPrice() which calculates the price of the pizza order as follows: You are free to design your interface, but you need to at least use ONE OF (numberOfToppings * toppingPrice) * pizzaSize EACH of the following ( you may use more that one of each if you like): - Method printOrderinfo () which prints only the customer's name and the - Label calculated order price on one line to the screen. - Button - CheckBox 2- class Delivery which extends PizzaOrder and contains the following attribute and - RadioButton methods: - ComboBox - tripRate (double) - TextField - zone ( int 1-4) - Appropriate constructors ( default and non default) as well as the appropriate setter and getter methods. The default GUI displayed should NOT have any Labels or Text fields for 0 toString0 method that overrides the method in PizzaOrder. (int). As soon as the user selects a pizza order of type Delivery, a tripRate - A calculateOrderPrice() method which overrides the method in PizzaOrder and adds the ( tripRate /100 totalprice * zone ) to the price. 3. class ToGo which extends PizzaOrder. 4. Class Seated which extends PizzaOrder and contains the following attribute and methods: - serviceCharge (double) - numberofPeople (int) o tostring() method that overrides the method in PizzaOrder. - A calculateOrderPrice() method which overrides the method in PizzaOrder appropriately and if he/she selects a pizza order of type Seated then the and adds the ( serviceCharge * numberOfPeople ) to the price. tripRate and zone Labels and Textfields should disappear and get replaced by a serviceCharge and numberOfPeople Labels and Textfields 5- A Driver class which includes the following methods: appropriately. When the user selects a ToGo pizza order then tripRate + - main method that does the following: zone OR serviceCharge + numberOfPeople labels and textfields should disappear from the GUI. creates an ArrayList called orders of type PizzaOrder and adds five different orders to it ( two Delivery, one ToGo, and two Seated). Your GUI should include three buttons at the bottom labeled ProcessOrder, You must NOT ask the user to enter the attributes but you PrintOrders, and Reset respectively. should fill them up directly in the main method. MAKE SURE YOUR CONSTRUCTORS FOLLOW THE SAME ORDER OF ATTRIBUTES AS SPECIFIED ABOVE AS FOLLOWS: After the user fills the form and presses the button ProcessOrder your program should do the following
Step by Step Solution
There are 3 Steps involved in it
Step: 1
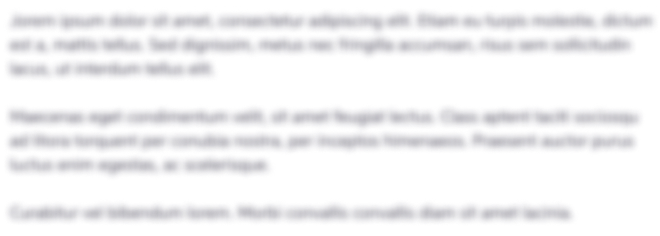
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started