Question
This is using MARS (MIPS Simulator) Even though you should never use the bubble-sort algorithm, it is a nice simple algorithm that still require you
This is using MARS (MIPS Simulator)
Even though you should never use the bubble-sort algorithm, it is a nice simple algorithm that still require you to manage multiple loops and conditionals :)
So, lets do it!
We will start by asking the user for a list of 10 numbers. A program is only useful it someone can use it for something! Then, using bubble-sort, the program will order those numbers in ascending order, and print the ordered numbers to the user.
This is what the code would look like in a high-level language (e.g., C):
void main(void) { static int list[10]; int i; // Ask the user for 10 numbers to fill the array for(i = 0; i < 10; i++) { print_string(" Insert a number: "); // syscall list[i] = read_integer(); // syscall } // Order them using bubble-sort int swapped, i, aux; do { swapped = 0; for (i = 0; i < 9; i++) { if (list[i] > list[i+1]) { aux = list[i]; list[i] = list[i+1]; list[i+1] = aux; swapped = 1; } } } while (swapped == 1); // Print the ordered list print_string(" The contents of the array are: "); // syscall for(i = 0; i < 10; i++) { print_integer(list[i]); // syscall print_char(' '); // syscall } }
Note the implementation of bubble-sort algorithm that orders the elements of the array list in ascending order. The bubble sort algorithm iterates the list of numbers, and compares each element in the list with the next one. If they are in the wrong order, then it swaps them. Then, it iterates the list repeatedly until no elements are swapped: i.e. they are in the desired order.
Hint: Note that we are comparing each element with the next, so the for cycle does not iterate All the elements ( i<9 ).
An equivalent assembly code is:
.data list: .word 0:10 request_for_input: .asciiz " Insert a number: " .text .globl main main: # variable i is in register s0 move s0, zero # for( i = 0; _main_forInsert: bge s0, 10, _main_endForInsert # i < 10; # i++) (*) check before main_endForInsert ## For implementation # Print request for input li v0, 4 la a0, request_for_input syscall # print_string(" Insert a number: "); // syscall # Get user input li v0, 5 syscall move s1, v0 # Copy the result of the syscall (v0) containing the user input into s1 # write list[i] # Calculate the memory address of list[i] la t1, list # Load the address of the list li t2, 4 # Load the size of a word mul t3, s0, t2 # Multiply i by the size of a word (i*4) add t4, t1, t3 # Calculate the memory address of element i (list +i*4) # Store the user value sw s1, 0(t4) # list[i] = s1 addi s0, s0, 1 # (*) i++ j _main_forInsert _main_endForInsert: # Exit li v0, 10 syscall
Task:
Write the C program above using MIPS assembly. Remember, after asking the user for the list of numbers, order them, and print them. |
The output should look something like:
Insert a number: 10 Insert a number: 9 Insert a number: 8 Insert a number: 7 Insert a number: 6 Insert a number: 5 Insert a number: 7 Insert a number: 2 Insert a number: 3 Insert a number: 4 The contents of the array are: 2 3 4 5 6 7 7 8 9 10
Step by Step Solution
There are 3 Steps involved in it
Step: 1
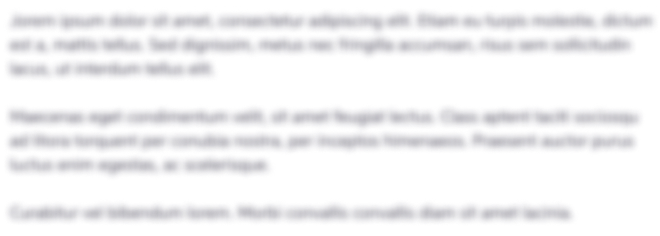
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started