Question
this is what i have so far for my java program. this needs to be added insertAnimalToWorld(String[][] animalLocations) Prompts the user to enter the x
this is what i have so far for my java program. this needs to be added
insertAnimalToWorld(String[][] animalLocations) Prompts the user to enter the x and y coordinate as well as their desired animal name and inserts it to the world in the location [x,y]as long as:
- The x and y coordinates are in the worlds boundaries
- The area [x,y] is not occupied by another animal
If the conditions are not met prompt the user to enter the coordinates as well as the animal name again until the conditions above are met.
removeAnimalFromWorld(String[][] animalLocations) Prompts the user to enter the x and y coordinate and removes the animal from the world in the location [x,y] as long as:
- The x and y coordinates are in the worlds boundaries
- The area [x,y] is not empty (contains an animal)
If the conditions are not met prompt the user to enter the coordinates until the conditions above are met.
Hint: To remove the animal you can just set the String at [x,y] to .
isEmptyBlock(String[][] animalWorld, int x, int y) Returns false if the array has an animal at [x,y] and true if [x,y] is empty.
updateobservedAnimals(String[] observedAnimals, String animal) adds animal into the observedAnimals array into the first occurrence of an empty String and returns the observedAnimals array.
printWorld(String[][] world) Prints the world in a grid/tabular format.
move(String[][] world, int x, int y, String[] observedAnimals, String[][] animalLocations) This method calls the makeWorld method to create a new world with the new user position and sets the 2D boolean array at [x,y] to be true, setting this area as explored. If [x,y] has an animal display the animal name and add it to the observed animals list and print the observed animals list, otherwise display you did not encounter anything :( . This method returns world at the end.
explore(String[][] world, String[][] animalLocations, String[] observedAnimals) Starts the exploration! This method prints out the world and then the move menu and asks the user to choose from the move menu until the user chooses to exit. In this method, make sure to declare the x and y variables that keep track of the users position in the world. When the user chooses to move forward for example, change the x and y values accordingly and make sure they are within the worlds bounds, if they are, call the move method and print the world, otherwise reset the position to 0,0 and tell the user that they went off bounds.
printobservedAnimals(String[] observedAnimals) Prints the observedAnimals list by printing each animal on one line separated by a space.
isExplored(int x, int y) Returns true if [x,y] is explored and false if [x,y] is not explored.
package assignent;
import java.util.Scanner;
/*
* PUESDOCODE:
* First you have to make the world. Using a method that assigns * to unexplored
areas and O to explored areas. Then use a different method to print a menu to the user that allows them to
choose from the menu. options are 1. insert an animal, 2. remove an animal, 3. explore the world.
If they chose to explore print the move menu with a method.If they chose to add an animal to the world add one
with a method that allows them to chose the x and y coordinate and the animal name. If they chose to remove an
animal from the world do it with a method that. asks for the x and y coordinate of the animal. Animal must be
at those coordinates. Make method to determine if an animal is at a spot by returning
true if there isn't an animal there and false if there is. Make a method that prints the game world in a grid
format. Make method that starts the exploration. Make a method that prints the observed animals
*/
public class three {
static Scanner input = new Scanner(System.in);
static int x = 0, y = 0;
static int prevX = 0, prevY = 0;
private static Object[][] updateworld;
public static void main(String[] args) {
String[][] animalLocations = { { "" }, { "" } };
String[][] observedAnimals = { { "" }, { "" } };
int posX = 0, posY = 0;
// displays instructions
instructions();
String[][] world = makeWorld();
worldCreate(world);
// display menus
updateWorld(world);
printMainMenu();
//printWorld(world);
int menuAnswer = input.nextInt();
if(menuAnswer == 1){
insertAnimalToWorld(world);
}
else if (menuAnswer == 2) {
removeAnimalFromWorld(world);
}
else {
System.out.println("Welcome to the world explorer!");
printMoveMenu();
}
}
public static String[][] makeWorld() {
// prompt user to enter length and width of the world
System.out.println("Enter the dimensions of your jungle!");
System.out.println("Enter length: ");
int column = input.nextInt();
System.out.println("Enter width: ");
int row = input.nextInt();
return new String[row][column];
}
// instructions
public static void instructions() {
System.out.println("Welcome to the jungle world creator! " +
"In this game, you will be inserting animals in certain places in the world that you create! You will also be allowed to remove animals from certain locations before you start exploring! " +
"Once you start exploring, you will navigate around the world to observe the animals that you have inserted. " +
"The game will keep track of all the animals you have observed! ");
}
// printing main menus
public static void printMainMenu() {
System.out.println("1. Insert an animal into the world");
System.out.println("2. Remove an animal from the world");
System.out.println("3. Explore the world");
}
// printing the world
public static void printWorld(String[][] arrayIn) {
for (int row = 0; row < arrayIn.length; row++) {
for (int column = 0; column < arrayIn[row].length; column++) {
System.out.print(arrayIn[row][column] + " ");
}
System.out.println();
}
}
// starting exploration
public static void explore(String[][] world, String[][] animalLocations, String[][] observedAnimals) {
System.out.println("Welcome to the world explorer!");
}
// initialize the world
public static void worldCreate(String[][] world) {
for (int column = 0; column < world.length; column++) {
for (int row = 0; row < world[column].length; row++) {
world[column][row] = "*";
}
}
}
public static void updateWorld(String[][] world) {
world[prevX][prevY] = "O";
world[x][y] = "X";
prevX = x; prevY = y;
printWorld(world);
}
// printing move menu
private static void printMoveMenu() {
System.out.println("W. Move Forward");
System.out.println("A. Move Left");
System.out.println("S. Move Backward");
System.out.println("D. Move Right");
System.out.println("I. Display observed animals");
System.out.println("E. Exit");
String moveAnswer = input.next();
}
public static void insertAnimalToWorld(String[][]animalLocations) {
System.out.println("Enter your desired (x,y) coordinate to add animal");
int coordinate1 = input.nextInt();
int coordinate2 = input.nextInt();
System.out.println("What kind of animal would you like to add?");
String animal = input.next();
int[][] updateworld = new int[coordinate1][coordinate2];
//System.out.println(coordinate1 + updateworld.length + updateworld[0].length);
for (int row = 0; row < updateworld.length; row++) {
for (int column = 0; column < updateworld[row].length; column++) {
updateworld[row][column] = input.nextInt();
}
}
}
public static void removeAnimalFromWorld(String[][]animalLocations) {
System.out.println("Enter the desired (x,y) coordinate to remove animal");
int coordinate3 = input.nextInt();
int coordinate4 = input.nextInt();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
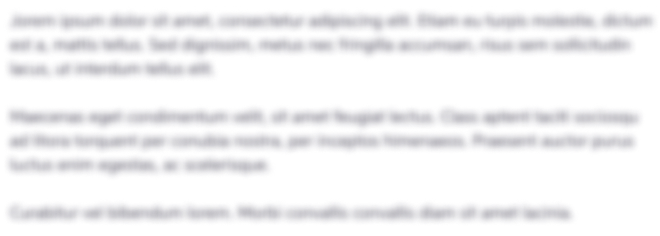
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started