Answered step by step
Verified Expert Solution
Question
1 Approved Answer
This lab is an extension of Lab 6 (IntList intro). In this lab you are required to implement following member functions for IntList class: void
This lab is an extension of Lab 6 (IntList intro). In this lab you are required to implement following member functions for IntList class:
- void push_back(int value): Inserts a data value (within a new node) at the end of the list.
- void pop_back(): Removes the data value at the end of the list.
- IntList(const IntList& item): Copy constructor.
- IntList& operator=(const IntList& item): Copy assignment operator.
For background make sure to read "Rule of three (section 10.12 on ZyBooks)". https://learn.zybooks.com/zybook/UCRCS013Winter2018/chapter/10/section/12
IntList.h
#ifndef INTLIST_H #define INTLIST_H struct IntNode { int data; IntNode* next; IntNode(int data) : data(data), next(0) { } }; class IntList { private: IntNode* head; IntNode* tail; public: IntList(const IntList& item); //Copy constructor IntList(); //Default constructor ~IntList(); IntList& operator=(const IntList& item); //Copy assignment operator void display() const; void push_front(int); void push_back(int); void pop_back(); void pop_front(); bool empty() const; }; #endif
main.cpp test harness for lab
Use this main.cpp file for testing your IntList.
#include #include "IntList.h" using namespace std; int main() { cout << "Enter a test number(1-3): "; int test; cin >> test; //test for push_back and pop_back if(test == 1) { cout << " list1 constructor called"; IntList list1; cout << " pushback 10"; list1.push_back( 10 ); cout << " pushback 20"; list1.push_back( 20 ); cout << " pushback 30"; list1.push_back( 30 ); cout << " list1: "; list1.display(); cout << " pop"; list1.pop_back(); cout << " list1: "; list1.display(); cout << " pop"; list1.pop_back(); cout << " list1: "; list1.display(); cout << " pop"; list1.pop_back(); cout << " list1: "; list1.display(); cout << endl; } //test for assignment operator else if(test == 2){ cout << " default constructor called for list1 and list2"; IntList list1,list2; list1.push_back(1); list1.push_back(2); list1.push_back(3); list1.push_back(4); cout << " list1: "; list1.display(); list2.push_back(6); list2.push_back(7); list2.push_back(8); list2.push_back(9); cout << " list2: "; list2.display(); cout << " calling assignment operator"; //test 1 list1 = list2; cout << " list1: "; list1.display(); cout << " calling assignment operator"; //test 2 list1 = list1; cout << " list1: "; list1.display(); cout << endl; } //test for copy constructor else if(test == 3){ cout << " default constructor called for list1"; IntList list1; list1.push_back(1); list1.push_back(2); list1.push_back(3); list1.push_back(4); cout << " list1: "; list1.display(); cout << " calling copy constructor"; //test 1 IntList list2(list1); cout << " list2: "; list1.display(); cout << " calling copy constructor"; //test 2 IntList list3 = list1; cout << " list3: "; list3.display(); cout << endl; } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
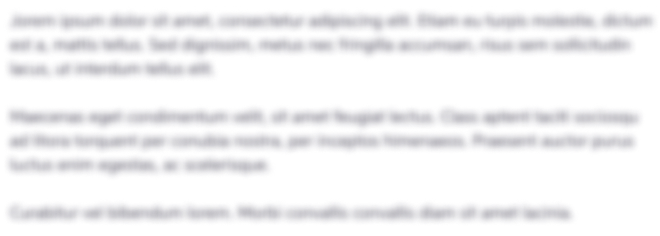
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started