Question
This lab lets you create a Swing component that displays a real-time animation using Java concurrency to execute an animation thread that runs on its
This lab lets you create a Swing component that displays a real-time animation using Java concurrency to execute an animation thread that runs on its own independently of what the human user happens to be doing with the component. This thread will animate a classic particle field where a swarm of thousands of independent little particles buzzes randomly around the component. (This same architecture could then, with surprisingly little modification, be used to implement some real-time game, with this animation thread moving the game entities according to the rules of the game 50 frames per second, and the event listeners giving orders to the game entity that happens to represent the human player.)
First, create a class Particle whose instances represent individual particles that will randomly around the two-dimensional plane. Each particle should remember its x- and y-coordinates on the two-dimensional plane, stored in two data fields of type double. This class should also have a random number generator shared between all objects:
private static final Random rng = new Random();
The class should then have the following methods:
public Particle(int width, int height)
The constructor that places this particle in random coordinates inside the box whose possible values for the x-coordinate range from 0 to width, and for the y-coordinate from 0 to height.
public double getX()
public double getY()
The accessor methods for the current x- and y-coordinates of this particle.
public void move()
Adds the value of the expression rng.nextGaussian() separately to the x- and y-coordinates of this particle, thus moving this particle a random distance to a random direction in the spirit of Brownian motion.
Having completed the class to represent individual particles, write a class ParticleField that extends javax.swing.JPanel. The instances of this class represent an entire field of random particles. This class should have the following private instance fields.
private boolean running = true;
private java.util.List
new java.util.ArrayList
The class should have the following public methods:
public ParticleField(int n, int width, int height)
The constructor that first sets the preferred size of this component to be width-by-height. Next, creates n separate instances of Particle and places them in the particles list.
Having initialized the individual particles, this constructor should create and launch one new Thread using a Runnable argument whose method run consists of one while(running) loop. The body of this loop should first sleep for 20 milliseconds. After waking up from its sleep, it should loop through all the particles and call the method move() for each of them. Then call repaint() and go to the next round of the while-loop.
@Override public void paintComponent(Graphics g)
Renders this component by looping through particles and rendering each one of them as a 3-by-3 pixel rectangle to its current x- and y-coordinates.
public void terminate()
Sets the field running of this component to be false, causing the animation thread to terminate in the near future.
To admire the literal and metaphorical buzz produced by your particle swarm, write another class ParticleMain that contains a main method that creates one JFrame instance that contains a ParticleField of size 800-by-800 that contains 2,000 instances of Particle. Using the main method of the example class SpaceFiller as a model of how to do this, attach a WindowListener to the JFrame so that the listener's method windowClosing first calls the method terminate of the ParticleField instance shown inside the JFrame before disposing that JFrame itself.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
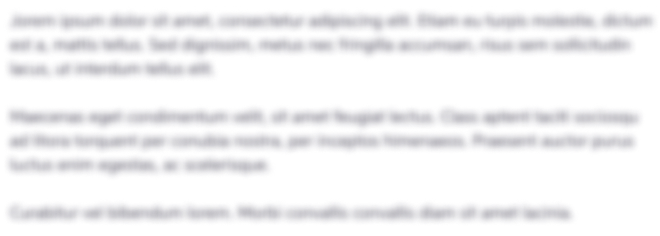
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started