Question
This lab will introduce you to threading where - by default - threads belonging to the same process share the same address space. POSIX Shared
This lab will introduce you to threading where - by default - threads belonging to the same process share the same address space.
POSIX Shared Memory
We will illustrate POSIX shared memory using the Producer-Consumer model.
The Lab
This lab involves creating a separate, "child" thread that generates the Collatz sequence and writing it to global data that it shares with the "parent" thread. When the child thread terminates, the parent will output the sequence.
Your program will run similarly to the previous labs where you will pass the starting number of the command line, such as
./lab3 13
You will have some interesting choices for a data structure to store the sequence. One approach is to simply use an array of integer values to store the numbers in the sequence. For example, you may create a global array of the following capacity:
const int SIZE = 25; int sequence[SIZE]
The obvious issue with this approach is that static allocation may either be too large (you have created an array much larger than necessary) or too small (the array is too small to store the sequence).
A better strategy is to use a linked list. The following program demonstrates how to use a linked list in C:
- list.c
Using the Pthreads program as an example
- thrd-posix.c
Write a multithreaded program that has the child thread construct and populate the list containing the Collatz sequence. When the child thread has terminated, the parent will output the sequence by traversing the list.
List.c:
/** Demonstration of creating and traversing a linked list in C. */ #include#include // the elements in the list struct element { int data; struct element *next; }; // a pointer to the head of the list struct element *head; // a temporary pointer used for freeing memory struct element *temp; int main(void) { int n = 5; struct element *node; // malloc allocates the memory to hold a struct element node = (struct element *) malloc(sizeof(struct element)); head = node; while (n > 0) { // populate the list node->data = n; printf("%d ",node->data); node->next = (struct element *) malloc(sizeof(struct element)); node = node->next; n--; } node->next = NULL; // traverse the list while (head->next != NULL) { printf("%d ",head->data); // free memory after visiting a node temp = head; head = head->next; free(temp); } return 0; }
thrd-posix.c:
/** * A pthread program illustrating how to * create a simple thread and some of the pthread API * This program implements the summation function where * the summation operation is run as a separate thread. * * Most Unix/Linux/OS X users * gcc thrd.c -lpthread */ #include#include #include int sum; /* this data is shared by the thread(s) */ void *runner(void *param); /* the thread */ int main(int argc, char *argv[]) { pthread_t tid; /* the thread identifier */ pthread_attr_t attr; /* set of attributes for the thread */ if (argc != 2) { fprintf(stderr,"usage: a.out "); /*exit(1);*/ return -1; } if (atoi(argv[1]) < 0) { fprintf(stderr,"Argument %d must be non-negative ",atoi(argv[1])); /*exit(1);*/ return -1; } /* get the default attributes */ pthread_attr_init(&attr); /* create the thread */ pthread_create(&tid,&attr,runner,argv[1]); /* now wait for the thread to exit */ pthread_join(tid,NULL); printf("sum = %d ",sum); } /** * The thread will begin control in this function */ void *runner(void *param) { int i, upper = atoi(param); sum = 0; if (upper > 0) { for (i = 1; i <= upper; i++) sum += i; } pthread_exit(0); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
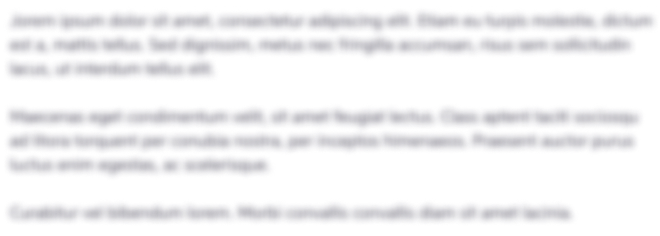
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started