Question
This lab will test your ability to perform deep and shallow copies, overload operators, friendship, and use the copy constructor and assignment as well as
This lab will test your ability to perform deep and shallow copies, overload operators, friendship, and use the copy constructor and assignment as well as the move constructor and assignment.You have to write three files.
This program creates objects of type House. Each house has an owner, address, rooms, bathrooms, and a price. The class functions that operate on this data are as follows:
House();//Default constructor
House(const char* _owner, const char* _address, const double _rooms, const double _bathrooms, const double _price);//Data for the house object
House(const House& house);//Copy constructor
House& operator=(const House& house);//Copy assignment
House(House&& house) noexcept;//Move constructor
House& operator=(House&& house) noexcept;//Move assignment
bool ChangeOwner(char* newOwner);//Change the owner. Test to see if the character array newOwner actually exists. If it doesn't, return false, otherwise return true.
bool ChangePrice(double newPrice);//Change the price. Test to see if the price is a positive number. If it isn't, return false, otherwise return true.
const House& operator+=(double amount);//increase/decrease the price of the house
void DisplayInfo() const;//Display the owner, address, rooms, bathrooms, and price. All double's should be printed to two decimal places.
~House();//Destructor
There are also two functions that operate on this data from outside the class. They are as follows:
bool operator==(const House& house1, const House& house2);//Do the two houses have the same owner?
void operator>>(House& house1, House& house2);//Move contents from the house1 object to the house2 object
Note that operator== might require access to private members of the House object, so you may have to modify House.h. Also, you might decide that operator== and operator>> can be implemented within the class. You are free to move them to within the class if you wish.
You have been given a header file for House and a main file for testing: House.hand HouseMain.cpp. Complete House.cpp.You have to write three files.
A sample run is as follows:
Owner: Lionel Messi Address: 34 Cedarwood Road Rooms: 5.00 Bathrooms: 2.50 Price: $2500000.00 Owner: Neymar da Silva Santos Junior Address: 12 Broadway Street Rooms: 7.00 Bathrooms: 4.00 Price: $5600000.00 Owner: Lionel Messi Address: 34 Cedarwood Road Rooms: 5.00 Bathrooms: 2.50 Price: $2500000.00 Owner: Neymar da Silva Santos Junior Address: 12 Broadway Street Rooms: 7.00 Bathrooms: 4.00 Price: $5600000.00 ---------------------------- Owner: none Address: none Rooms: 0.00 Bathrooms: 0.00 Price: $0.00 Owner: none Address: none Rooms: 0.00 Bathrooms: 0.00 Price: $0.00 Owner: Lionel Messi Address: 34 Cedarwood Road Rooms: 5.00 Bathrooms: 2.50 Price: $2500000.00 Owner: Neymar da Silva Santos Junior Address: 12 Broadway Street Rooms: 7.00 Bathrooms: 4.00 Price: $5600000.00 Owner: Lionel Messi Address: 34 Cedarwood Road Rooms: 5.00 Bathrooms: 2.50 Price: $2500000.00 Owner: Neymar da Silva Santos Junior Address: 12 Broadway Street Rooms: 7.00 Bathrooms: 4.00 Price: $5600000.00 ---------------------------- Houses 3 and 5 are the same Houses 4 and 6 are the same ---------------------------- Owner: Lionel Messi Address: 34 Cedarwood Road Rooms: 5.00 Bathrooms: 2.50 Price: $3500000.00 Owner: Neymar da Silva Santos Junior Address: 12 Broadway Street Rooms: 7.00 Bathrooms: 4.00 Price: $6600000.00 Owner: Lionel Messi Address: 34 Cedarwood Road Rooms: 5.00 Bathrooms: 2.50 Price: $2500000.00 Owner: Neymar da Silva Santos Junior Address: 12 Broadway Street Rooms: 7.00 Bathrooms: 4.00 Price: $5600000.00 ---------------------------- Owner: none Address: none Rooms: 0.00 Bathrooms: 0.00 Price: $0.00 Owner: none Address: none Rooms: 0.00 Bathrooms: 0.00 Price: $0.00 Owner: Lionel Messi Address: 34 Cedarwood Road Rooms: 5.00 Bathrooms: 2.50 Price: $3500000.00 Owner: Neymar da Silva Santos Junior Address: 12 Broadway Street Rooms: 7.00 Bathrooms: 4.00 Price: $6600000.00
To assist you with this lab, you might want to look at the following sample code taken from our SEP101 final exam. A Warehouse class is implemented using operator overloading and copy/move constructor and assignment operators: Warehouse.h, Warehouse.cpp and WarehouseMain.cpp.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
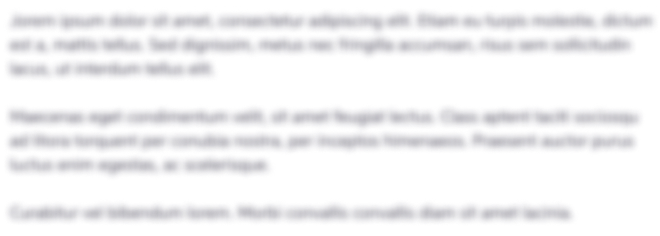
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started