Question
This lesson asks you to write a program that applies a bubble sort to the classarray of SYCS objects: SYCS classarray[9]; you have to sort
This lesson asks you to write a program that applies a bubble sort to the classarray of SYCS objects:
SYCS classarray[9]; you have to sort the average gpas for the classes which the program already does with the heap but you have to modify it so the average gpas will be sorted useing bubble sort code must be c++ thank you
#include
#include
#include
#include
using namespace std;
class student
{
public:
student();
student(string std_name, string std_major, string std_ID, float std_GPA);
string getname();
string getmajor();
string getID();
float getGPA();
student* getnext();
bool operator> (student);
void setname(string);
void setmajor(string);
void setID(string);
void setGPA(float);
void setnext(student*);
void printstudent();
void setstudent(string Student_name, string Student_major, string Student_ID, float Student_GPA);
void setstudent(student);
private:
string std_name;
string std_major;
string std_ID;
float std_GPA;
student* Next;
};
student::student()
{
std_name = "";
std_major = "";
std_ID = "";
std_GPA = 0.0;
Next = NULL;
}
student::student(string Student_name, string Student_major, string Student_ID, float Student_GPA)
{
std_name = Student_name;
std_major = Student_major;
std_ID = Student_ID;
std_GPA = Student_GPA;
}
bool student::operator>(student other)
{
return std_GPA > other.std_GPA;
}
string student::getname()
{
return std_name;
}
string student::getmajor()
{
return std_major;
}
string student::getID()
{
return std_ID;
}
float student::getGPA()
{
return std_GPA;
}
student* student::getnext()
{
return Next;
}
void student::setname(string name)
{
std_name = name;
}
void student::setmajor(string major)
{
std_major = major;
}
void student::setID(string id)
{
std_ID = id;
}
void student::setGPA(float GPA)
{
std_GPA = GPA;
}
void student::setnext(student* next_std)
{
Next = next_std;
}
void student::printstudent()
{
cout << endl << std_name;
cout << endl << std_ID;
cout << endl << std_major;
cout << endl << std_GPA << endl;
}
void student::setstudent(string Student_name, string Student_major, string Student_ID, float Student_GPA)
{
std_name = Student_name;
std_major = Student_major;
std_ID = Student_ID;
std_GPA = Student_GPA;
}
void student::setstudent(student new_student)
{
std_name = new_student.getname();
std_major = new_student.getmajor();
std_ID = new_student.getID();
std_GPA = new_student.getGPA();
}
class SYCS
{
public:
student * first_std;
SYCS();
~SYCS();
SYCS& operator=(SYCS&);
void insertInOrder(student);
void add_front(student);
void add_end(student);
void addstudent(student);
void printclass(student*);
student* getfirst_std();
private:
};
SYCS::SYCS()
{
first_std = NULL;
}
SYCS& SYCS::operator=(SYCS& rhs)
{
if (this != &rhs) // if not A = A
{
student *curr, *nextNode;
curr = first_std;
nextNode = NULL;
while (curr) // destroy A
{
nextNode = curr->getnext();
delete curr;
curr = nextNode;
}
student *cptr = rhs.first_std; //
while (cptr != NULL)
{
add_end(*cptr);
cptr = cptr->getnext();
}
}
return *this;
}
void SYCS::insertInOrder(student data)
{
student* current;
student* previous = first_std;
student* nodePtr = new student; // declare a new nodeTyp
nodePtr->setstudent(data);// with the itemType of the parameter
if ((first_std == NULL) || (first_std->getGPA() > data.getGPA())) // check to see if the sortedListType is empty
{
add_front(data); // if so, simply add a car on the front
return;
}
else
{
for (current = first_std;
((current != NULL) && (current->getGPA() < data.getGPA()));
current = current->getnext())
{
previous = current;
}
if (current == NULL)
{
add_end(data);
return;
}
// needs to be inserted between previous and current
previous->setnext(nodePtr); // link new nodeType to previous
nodePtr->setnext(current);
}
}
void SYCS::addstudent(student pupil)
{
student* new_student = new student; //make a new student location
new_student->setstudent(pupil); //and fill it with the information of the student to be added
if (first_std == NULL) // if it's the first student to be put in the list just point first_std at it
{
first_std = new_student;
}
else // if it's not the first student to be put in list....
{
student* current = first_std;
student* previous = NULL;
while (pupil.getGPA() > current->getGPA() && current->getnext() != NULL) // find the spot where the student to be added goes
{
previous = current;
current = current->getnext();
}
if (previous == NULL) // if the student belongs in the beginning
{
new_student->setnext(first_std); //new student goes at beginning
first_std = new_student; // first student now point at new beginning
}
else if (current->getnext() == NULL && new_student->getGPA() > current->getGPA()) // if it is the greatest GPA yet to come just tack it on the end
{
current->setnext(new_student);
}
else // if it belongs between two different students....
{
previous->setnext(new_student);
new_student->setnext(current);
}
}
}
void SYCS::add_front(student data) // add first nodeType to sortedListType
{
if (first_std == NULL)
{
first_std = new student;
first_std->setnext(NULL);
first_std->setstudent(data);
}
else
{
student* hold_front;
hold_front = first_std;
first_std = new student;
first_std->setnext(hold_front);
first_std->setstudent(data);
}
}
void SYCS::add_end(student data) // add car to end
{
if (first_std == NULL)
{
add_front(data);
return;
}
else //find the end of the train(traverse the train)
{
student* current;
for (current = first_std; current->getnext() != NULL;
current = current->getnext()); //no body or use {} without ;
current->setnext(new student);
current->getnext()->setnext(NULL);
current->getnext()->setstudent(data);
}
}
void SYCS::printclass(student* First_Student)
{
if (First_Student->getnext() != NULL) // general case... base case is implicit.
printclass(First_Student->getnext());
First_Student->printstudent();
}
student* SYCS::getfirst_std()
{
return first_std;
}
SYCS::~SYCS()
{
student* deleter;
while (first_std != NULL)
{
deleter = first_std;
first_std = first_std->getnext();
delete deleter;
}
}
struct node
{
public:
string course_code; // 7 character course code
student* first; // points to linked-list of students
bool operator> (node);
bool operator< (node);
bool operator <= (node);
float getaverage(student*);
};
bool node::operator> (node other)
{
return getaverage(first) > other.getaverage(other.first);
}
bool node::operator< (node other)
{
return getaverage(first) < other.getaverage(other.first);
}
bool node::operator<= (node other)
{
return getaverage(first) <= other.getaverage(other.first);
}
float node::getaverage(student* begin)
{
float average = 0.0, total = 0.0, length = 0.0;
if (begin != NULL)
{
while (begin->getnext() != NULL) // general case... base case is implicit.
{
total = total + begin->getGPA();
length++;
begin = begin->getnext();
}
total = total + begin->getGPA();
length++;
}
//cout << "returns " << (total / length) << endl;
return (total / length);
}
class HeapType
{
private:
public:
int length;
node elements[20];
HeapType();
void Enqueue(node);
void Dequeue(node&);
void ReheapDown(int, int);
void ReheapUp(int, int);
void Swap(int, int);
};
HeapType::HeapType()
{
length = 0;
}
void HeapType::Enqueue(node newItem)
{
if(length==20){
cout<<"Full"< } else{ length++; elements[length-1]=newItem; ReheapUp(0,length-1); } //YOU MUST WRITE THIS CODE } void HeapType::Dequeue(node& item) { if(length==0){ cout<<"empty"< } else { item=elements[0]; elements[0]=elements[length-1]; length--; ReheapDown(0, length-1); } //YOU MUST WRITE THIS CODE } void HeapType::ReheapUp(int root, int bottom) { //compare tp the parent int parent; //test to see if bottom is greater than the root if(bottom>root) { parent=(bottom-1)/2; if(elements[parent] { Swap(parent,bottom); ReheapUp(root,parent); } } } void HeapType::Swap(int A, int B) { node temp; temp=elements[A]; elements[A]=elements[B]; elements[B]=temp; //need a third thing to hold one of them //we are swapping sycs objects //YOU MUST WRITE THIS CODE } void HeapType::ReheapDown(int root, int bottom) { int maxchild,rightchild,leftchild; leftchild=(root*2)+1; rightchild=(root*2)+2; if(leftchild<=bottom) { if(leftchild==bottom) { maxchild=leftchild; } else { if(elements[leftchild]<=elements[rightchild]){ maxchild=rightchild; } else{ maxchild=leftchild; } if(elements[root] Swap(root,maxchild); ReheapDown(maxchild,bottom); } } } } int main() { string name; string fname; string lname; string maj; string id; float gpa; char dummy; ifstream infile; student the_student; SYCS spring2018; SYCS classarray[9]; int count = 0; while (count < 9) { switch (count) { case 0: infile.open("infile1.txt"); break; case 1: infile.open("infile2"); break; case 2: infile.open("infile3.txt"); break; case 3: infile.open("infile4.txt"); break; case 4: infile.open("infile5.txt"); break; case 5: infile.open("infile6.txt"); break; case 6: infile.open("infile7.txt"); break; case 7: infile.open("infile8.txt"); break; case 8: infile.open("infile9.txt"); break; default: infile.open("infile.txt"); } getline(infile, name); getline(infile, maj); getline(infile, id); infile >> gpa; infile.get(dummy); while (infile) //populate the class with eof loop. { the_student.setstudent(name, maj, id, gpa); spring2018.add_front(the_student); getline(infile, name); getline(infile, maj); getline(infile, id); infile >> gpa; infile.get(dummy); } classarray[count] = spring2018; infile.close(); count++; } HeapType theheap; node thenode; string classname; count = 0; while (count < 9) { switch (count) { case 0: classname = "SYCS 135"; break; case 1: classname = "SYCS 354"; break; case 2: classname = "SYCS 660"; break; case 3: classname = "SYCS 470"; break; case 4: classname = "SYCS 788"; break; case 5: classname = "SYCS 624"; break; case 6: classname = "SYCS 540"; break; case 7: classname = "SYCS 450"; break; case 8: classname = "SYCS 136"; break; default: classname = "SYCS 000"; } thenode.course_code = classname; thenode.first = classarray[count].getfirst_std(); theheap.Enqueue(thenode); count++; } count = 0; node x; while (theheap.length != 0) { theheap.Dequeue(x); cout << x.course_code << " " << setprecision(4) << x.getaverage(x.first) << endl; count++; } //system("pause"); return 0; } /* SYCS 788 3.054 SYCS 470 3.044 SYCS 624 2.994 SYCS 136 2.991 SYCS 450 2.983 SYCS 540 2.975 SYCS 660 2.967 SYCS 135 2.533 SYCS 354 2.533 */ infile1.txt steve Jobs Computer & Science @1800001 3.0 Jhonny Blaze Psychology @76547787 2.0 Aubrey Graham Fashion Design @00032452 2.3 Micheal Jordan Sports Medicine @45982323 3.0 Betty Davis Electrical Engineering @8099000 1.8 James Bond Criminal Justice @23458007 3.1 infile2.txt Fred Cook Computer Science @1324354 3.4 Betty Davis Electrical Engineering @8099000 1.8 Jim Rockville Mathematics @1155000 4.00 Kevin Smith English @2230090 3.1 Emmanuel Ademuwagun Electrical Engineer @552235 4.0 Ronald Doku Computer Science @256897 3.5 Fola Onibiyo Computer Science @215893 3.7 infile3.txt Alexis Desouza Information Systems @489562 3.8 Desayo Ajisegiri Chemical Engineer @359874 4.0 Jeremy Blackstone Computer Science @859647 3.7 infile4.txt Kevin Howard English @4230090 3.6 Mary Goins English @1230090 3.5 Elisabeth McArthur English @1230090 3.3 Terrance Howard English @2239990 3.2 Vinnie Okoli English @2235090 3.7 J'adore Tillman English @2430090 1.8 Veronica Goins English @2230091 2.9 infile5.txt Layla Smith English @2130090 3.9 Smith Jared English @2231190 1.3 Lauren Taylor English @4230090 2.1 Kevin Howard English @4230090 3.6 Mary Goins English @1230090 3.5 Elisabeth McArthur English @1230090 3.3 Terrance Howard English @2239990 3.2 Vinnie Okoli English @2235090 3.7 infile6.txt Malachi Schirner @0265425 Computer Engineering 1.9 Michelle Washington @0264358 Biology 2 August Hayes @5469632 Mechanical Engineering 2.7 Ashley Williams @0268547 Anthropology 2.9 Kevin Smith @2230090 English 3.1 Portia Herndon @0264311 Computer Science 3.1 Fred Cook @1324354 Computer Science 3.4 Micheal Blacksmith @2548596 Musical Theatre 3.4 infile7.txt Angelica Pickles @2489657 Education 3.7 Brittany Anderson @3456987 Musical Therapy 3.8 Jim Rockville @1155000 Mathematics 1.4 George Rodriguez @8546985 Dance 2.4 infile8.txt Shakir Mack Advertising @0987655 2.23 Omed Muzzafery Nuclear engineering @0243378 3.54 Glenn Reyes Psychology @0345163 3.12 Erik Ellis Business @0987654 3.33 Dougal Hutton Information Systems @0954876 3.0 infile9.txt Kevin Smith English @2230090 3.1 Bobby johnson Speech Pathology @0243389 2.33 Jacinto Cox Film @0345567 3.16 Steve White Biology @0145563 2.6 Terrance Ellis Computer Science @02599017 3.87 Mike Mitchell Film @0946784 3.2
Step by Step Solution
There are 3 Steps involved in it
Step: 1
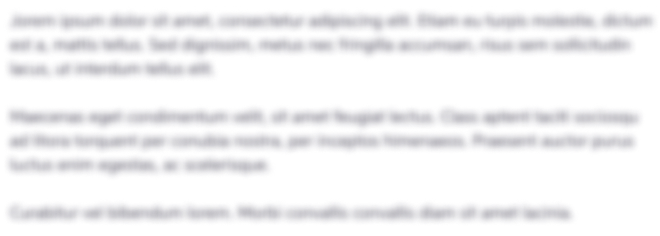
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started