Question
This main lab extends the earlier prep lab Online shopping cart Part 1 (CODE INCLUDED BELOW AND IT'S CORRECT). You will create an on-line shopping
This main lab extends the earlier prep lab "Online shopping cart Part 1" (CODE INCLUDED BELOW AND IT'S CORRECT). You will create an on-line shopping cart like you might use for your on-line purchases. The goal is to become comfortable with setting up classes and using objects.
THIS IS WHAT IS ALREADY SOLVED IN PART 1(THIS IS CORRECT SO FAR)
#ifndef ItemToPurchase_H_
#define ItemToPurchase_H_
#include
using namespace std;
class ItemToPurchase {
private:
string itemName;
double itemPrice;
int itemQuantity;
public:
ItemToPurchase();
void SetName(string name);
void SetPrice(double price);
void SetQuantity(int quantity);
string GetName();
double GetPrice();
int GetQuantity();
};
#endif
#include "ItemToPurchase.h"
ItemToPurchase::ItemToPurchase() {
itemName = "none";
itemPrice = 0.0;
itemQuantity = 0;
}
void ItemToPurchase::SetName(string name) {
itemName = name;
}
void ItemToPurchase::SetPrice(double price) {
itemPrice = price;
}
void ItemToPurchase::SetQuantity(int quantity) {
itemQuantity = quantity;
}
string ItemToPurchase::GetName() {
return itemName;
}
double ItemToPurchase::GetPrice() {
return itemPrice;
}
int ItemToPurchase::GetQuantity() {
return itemQuantity;
}
#include
#include "ItemToPurchase.h"
#include
using namespace std;
int main() {
ItemToPurchase item1, item2;
string name;
double price;
int q;
cout
cout
getline(cin, name);
cout
cin >> price;
cout
cin >> q;
item1.SetName(name);
item1.SetPrice(price);
item1.SetQuantity(q);
cout
cout
cin.ignore();
getline(cin, name);
cout
cin >> price;
cout
cin >> q;
item2.SetName(name);
item2.SetPrice(price);
item2.SetQuantity(q);
cout
cout
cout
cout
cout
return 0;
}
//END OF BEGINNING PART TO GET US STARTED ON THIS LAB
START HERE:
Requirements
Expanded ItemToPurchase Class (15 points)
Extend the ItemToPurchase class as follows. We will not do unit testing in this lab so we will not be giving you the names of the member functions. Create good ones on your own.
Create a parameterized constructor to assign item name, item description, item price, and item quantity (default values of "none" for name and description, and 0 for price and quantity).
Additional public member functions
Set an item description
Get an item description
Print the cost of an item - Outputs the item name followed by the quantity, price, and subtotal (see example)
Print the description of an item - Outputs the item name and description (see example)
Additional Private data members
a string for the description of the item.
Example output of the function which prints the cost of an item:
Bottled Water 10 @ $1.50 = $15.00
Example output of the function which prints the item description:
Bottled Water: Deer Park, 12 oz.
ShoppingCart Class (25 points)
Create three new files - Name all your files exactly as specified as that is required for zyBook file submission.
ShoppingCart.h - Class declaration
ShoppingCart.cpp - Class definition
main.cpp - main function (main's functionality will differ significantly from that of the prep lab)
Build the ShoppingCart class with the following specifications. Note: Some functions can be function stubs (empty functions) initially, to be completed in later steps. Also, we usually declare all variable at the top of the code block. Note that you could be declaring some class objects after receiving user input, so that is a case where it would be all right to declare a variable later in your code.
Parameterized constructor which takes the customer name and date as parameters
Private data members
Name of the customer - Initialized in constructor to "none" if no parameter included
Date the cart was created - Initialized in constructor to "January 1, 2016" if no parameter included
Vector of items objects
Must have the following public member functions
Get the name of the customer
Get the date the cart was created
Add an item object to the cart vector. Accepts a new item object as a parameter.
If item name is already in the cart, output this message: "Item is already in cart. Nothing added."
Remove an item object from the cart. Accepts an item name as a parameter.
If item name cannot be found, output this message: "Item not found in cart. Nothing removed."
Update the quantity of an item object in the cart
If item name cannot be found, output this message: "Item not found in cart. Nothing modified." If the quantity is set to 0, still leave the item in the cart, but treat it as a 0 in all counts, etc.
Return the quantity of items in the cart
Return the total cost of all items in the cart
Print the total number and cost of items in the cart
If cart is empty, output this message: "Shopping cart is empty"
Print the description of each item in the cart
If cart is empty, output this message: "Shopping cart is empty"
Example output of the function which prints the number and costs of items in the cart:
John Doe's Shopping Cart - February 1, 2016 Number of Items: 8 Nike Romaleos 2 @ $189.00 = $378.00 Chocolate Chips 5 @ $3.50 = $17.50 Powerbeats 2 Headphones 1 @ $128.00 = $128.00 Total: $523.50
Example output of the function which prints the item descriptions:
John Doe's Shopping Cart - February 1, 2016 Item Descriptions Nike Romaleos: Volt color, Weightlifting shoes Chocolate Chips: Semi-sweet Powerbeats 2 Headphones: Bluetooth headphones
You may also create private helper functions in the ShoppingCart class if you'd like. Hint: A function to find if and where an item name resides in the cart could be helpful in multiple places.
Main Function Options Menu (8 points)
See the full input/output example below to know the expected format. In main, first prompt the user for a customer's name and today's date. Create an object of type ShoppingCart. Note that you have to declare (and thus create) your cart after you have input the name and date, since you have to set those with the parameterized constructor since you do not have setter functions for name and date.
In main, create a loop and allow the user to enter options to manipulate the cart. First implement the options and quit options. If the user enters an incorrect option, just print the options menu.
add - Add item to cart remove - Remove item from cart change - Change item quantity descriptions - Output items' descriptions cart - Output shopping cart options - Print the options menu quit - Quit
In this lab you may assume that when you prompt the user for an input, that they will input an appropriate value (e.g. an int would not be negative, or a string, etc.). Thus, you are not required to do error checking (you may if you want but we will not test for it). Note that in any "real-world" program, you would ALWAYS check for all possible input errors. However, we have done that sufficiently in previous labs for you to understand the concept, so in this lab you may assume legal inputs and focus on other programming aspects. The one exception is if the user enters an unknown option you will print the options menu. Note that you will still have a mix of >> and getline, so make sure you deal with that properly (See section 7.2 if necessary).
Implement the remaining user options (52 points). Think about what parameters need to be passed to class member functions, etc.
cart: Output shopping cart. Note that to do this, all you should need to do is call the appropriate member function on the shopping cart object. Note that by implementing printing options first, it is easy to test and verify later options as you implement them.
descriptions: Output description of item's in the cart.
add: Add an item to the cart.
change: Update the quantity of a current item in the cart to a new value.
remove: Remove an item from the cart.
Deductions graded by TA's
Adherence to the style guidelines. (up to 20 points)
Remember to review the section on Objects and Classes.
You now have multiple files, and each file should have it's own header.
Each class must be created and submitted in separate cpp and header files. (15 points)
Note that you can have all your files in a folder and then drag and drop them ALL AT ONCE into the zyBook submit file area, and zyBook will sort each file into its proper place.
You must solve this problem using classes and members as specified. You must not submit a solution that solves the lab, while not using classes. (60 points)
Sample Input
Mary Jane Lewis September 1, 2017 options descriptions cart add BYU game shirt Perfect shirt to wear with the ROC 5.00 2 add Sunscreen 50 SPF Perfect to avoid the fourth quarter burn 4.50 3 add Sunscreen 50 SPF Perfect to avoid the fourth quarter burn 4.50 1 descriptions cart change buffalo wings 4 change BYU game shirt 4 cart remove Sunscreen 50 SPF descriptions cart quit
Sample Output - Visual Studio View
Enter Customer's Name: Mary Jane Lewis Enter Today's Date: September 1, 2017 Enter option: options MENU add - Add item to cart remove - Remove item from cart change - Change item quantity descriptions - Output items' descriptions cart - Output shopping cart options - Print the options menu quit - Quit Enter option: descriptions Mary Jane Lewis's Shopping Cart - September 1, 2017 Shopping cart is empty. Enter option: cart Mary Jane Lewis's Shopping Cart - September 1, 2017 Shopping cart is empty. Enter option: add Enter the item name: BYU game shirt Enter the item description: Perfect shirt to wear with the ROC Enter the item price: 5.00 Enter the item quantity: 2 Enter option: add Enter the item name: Sunscreen 50 SPF Enter the item description: Perfect to avoid the fourth quarter burn Enter the item price: 4.50 Enter the item quantity: 3 Enter option: add Enter the item name: Sunscreen 50 SPF Enter the item description: Perfect to avoid the fourth quarter burn Enter the item price: 4.50 Enter the item quantity: 1 Item is already in cart. Nothing added. Enter option: descriptions Mary Jane Lewis's Shopping Cart - September 1, 2017 Item Descriptions BYU game shirt: Perfect shirt to wear with the ROC Sunscreen 50 SPF: Perfect to avoid the fourth quarter burn Enter option: cart Mary Jane Lewis's Shopping Cart - September 1, 2017 Number of Items: 5 BYU game shirt 2 @ $5.00 = $10.00 Sunscreen 50 SPF 3 @ $4.50 = $13.50 Total: $23.50 Enter option: change Enter the item name: buffalo wings Enter the new quantity: 4 Item not found in cart. Nothing modified. Enter option: change Enter the item name: BYU game shirt Enter the new quantity: 4 Enter option: cart Mary Jane Lewis's Shopping Cart - September 1, 2017 Number of Items: 7 BYU game shirt 4 @ $5.00 = $20.00 Sunscreen 50 SPF 3 @ $4.50 = $13.50 Total: $33.50 Enter option: remove Enter name of the item to remove: Sunscreen 50 SPF Enter option: descriptions Mary Jane Lewis's Shopping Cart - September 1, 2017 Item Descriptions BYU game shirt: Perfect shirt to wear with the ROC Enter option: cart Mary Jane Lewis's Shopping Cart - September 1, 2017 Number of Items: 4 BYU game shirt 4 @ $5.00 = $20.00 Total: $20.00 Enter option: quit Goodbye.
MY CURRENT START IS CORRECT AND IS INCLUDED BELOW:
main.cpp:
#include
#include "ItemToPurchase.h"
#include "ShoppingCart.h"
#include
using namespace std;
int main() {
ItemToPurchase item;
string name;
string desc;
double price;
int q;
string custName;
string date;
string option = "";
cout
getline(cin, custName);
cout
getline(cin, date);
ShoppingCart sCart(name, date);
sCart.setName(custName);
sCart.setDate(date);
do {
cout
getline(cin, option);
if(option == "options") {
sCart.showOption();
}
else if(option == "add") {
cout
cout
getline(cin, name);
cout
getline(cin, desc);
cout
cin >> price;
cout
cin >> q;
item.SetName(name);
item.SetDescription(desc);
item.SetPrice(price);
item.SetQuantity(q);
sCart.add(item);
//item.clean();
cin.ignore();
}
else if(option == "remove") {
cout
getline(cin, name);
sCart.remove(name);
}
else if(option == "change") {
cout
getline(cin, name);
cout
cin >> q;
item.SetName(name);
item.SetQuantity(q);
sCart.update(item);
//item.clean();
cin.ignore();
}
else if(option == "descriptions") {
sCart.showDescription();
}
else if(option == "cart") {
sCart.showCart();
}
else if (option != "quit") {
sCart.showOption();
}
} while(option != "quit");
cout
return 0;
}
//end of main.cpp
I still need ItemToPurchase.cpp, ShoppingCart.h, ItemToPurchase.h, and ShoppingCart.cpp files-- I need these files to link up correctly with my existing main.cpp to produce the correct output shown above--given the user input.
additional input and output:
INPUT :
Snow White Feb 21, 1456 add Dwarves Grumpy, Sleepy, etc. 50.00 7 add Wicked Witch Bad News 29.01 1 add Wicked Witch More Bad news 30.00 2 add Rotten Apple It only takes one 5.10 15 descriptions cart quit
OUTPUT :
Enter Customer's Name:
Enter Today's Date:
Enter option:
Enter the item name:
Enter the item description:
Enter the item price:
Enter the item quantity:
Enter option:
Enter the item name:
Enter the item description:
Enter the item price:
Enter the item quantity:
Enter option:
Enter the item name:
Enter the item description:
Enter the item price:
Enter the item quantity:
Item is already in cart. Nothing added.
Enter option:
Enter the item name:
Enter the item description:
Enter the item price:
Enter the item quantity:
Enter option:
Snow White's Shopping Cart - Feb 21, 1456
Item Descriptions
Dwarves: Grumpy, Sleepy, etc.
Wicked Witch: Bad News
Rotten Apple: It only takes one
Enter option:
Snow White's Shopping Cart - Feb 21, 1456
Number of Items: 23
Dwarves 7 @ $50.00 = $350.00
Wicked Witch 1 @ $29.01 = $29.01
Rotten Apple 15 @ $5.10 = $76.50
Total: $455.51
Enter option:
Goodbye.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
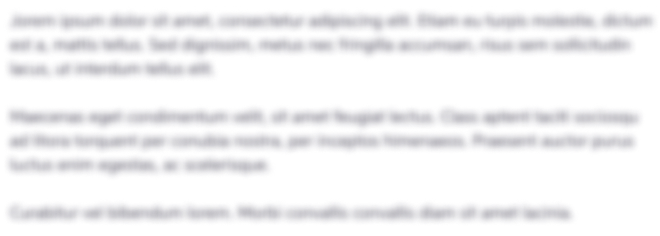
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started