Question
This practice exercise challenges you to work with a system of classes that share inheritance relationships. Your goal is to be able to analyze code
This practice exercise challenges you to work with a system of classes that share inheritance relationships. Your goal is to be able to analyze code like this and answer questions like these without using the computer to analyze or run the code. However, you could easily create and compile this code if you wish. Here are some partial class definitions (unimportant code has been omitted):
public class Wilma {
// contents not shown
} public class Fred extends Wilma {
public Slate emp; // other contents not shown
} public class Bedrock extends Slate {
// contents not shown
} public class Barney extends Fred {
// contents not shown
} public class Bambam extends Pebbles {
public String speak() { return "Bam Bam!"; } // other contents not shown
} public class Slate {
// contents not shown
} public class Pebbles extends Wilma {
public Dino friend; public String speak() { return "Goo Gah."; } // other contents not shown
} public class Dino extends Slate {
public Pebbles friend; // other not shown
}
Part 1: Draw a class diagram showing all of the inheritance (is-a) relationships among the classes defined above. Remember that a class diagram is different from an object diagram. A class diagram shows relationships among classes.
An inheritance (is-a) relationship exists when one class extends another class, and is represented by an arrow like this:
For example, in the code above, "Fred is-a Wilma, and Fred has-a Slate." This class diagram should help you with the rest of this activity. Part 2: Client code that makes use of the above system of classes includes the following sequence of statements: Bambam m = new Bambam(); Fred f = new Fred(); Barney b = new Barney(); Dino d = new Dino(); Pebbles p = m; Wilma w1 = f; Wilma w2 = p; // ADD one new statements here ONE AT A TIME for Part 3. This code declares 7 reference variables. Every reference variable has a static type and a dynamic type, which might be the same or might be different. What are the static and dynamic types for each of these variables?
m // Answer: f // Answer: b // Answer: d // Answer: p // Answer: w1 // Answer: w2 // Answer:
Part 3: Explain what would happen if each of the following statements were inserted ONE AT A TIME in the spot marked above. Answer the following questions for each statement:
Would the statement cause a syntax error?
If not, would the statement cause a ClassCastException?
For the last 4, if there is no syntax error or runtime exception, explain what output (if any) will be shown on the console.
1. w1 = b; // Answer:
2. m = w1; // Answer:
3. m = (Bambam)w2; // Answer:
4. f = (Slate)d; // Answer:
5. Slate s = d; // Answer:
6. w2 = new Pebbles(); // Answer:
7. p = (Pebbles)w1; // Answer:
8. d = new Bedrock(); // Answer:
9. System.out.println( m.speak() ); // Answer:
10. System.out.println( p.speak() ); // Answer:
11. System.out.println( w2.speak() ); // Answer:
12. System.out.println( (new Pebbles() ).speak() ); // Answer:
As with all practice activities, use the Discussion Forum to ask questions and share your results.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
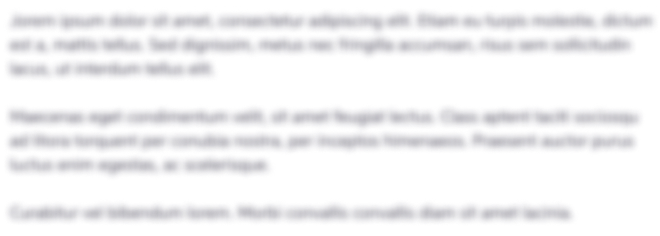
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started