Question
This program about write the TLB Class , I would like to get help to write this program by using the C++ language, And this
This program about write the TLB Class ,
I would like to get help to write this program by using the C++ language,
And this is the all anformation you need with some pictures:
the overview of the program :
The purpose of the programs in this course is to write a simulation of the virtual address translation and cache operations for an ARM processor. The general outline of the process is on page 131 of the text.
- 32-bit virtual address
- 32-but physical address
- 64KB page size (exceedingly large)
- 10-entry fully associative micro-TLB
- L2 TLB 512 entries, 4-way set associative
- L1 32K 64B 4-way
-L2 1MB 64B 16-way
Heres how this all works. The processor issues a 32-bit virtual address. Because L1 Cache is virtually tagged and indexed, L1 access can occur at the same time as the Micro-TLB lookup happens. The micro-TLB is 10 fully associative entries. We will consider that all entries operate the same way although this is not entirely true in reality. In order to access the micro-TLB, a tag needs to be obtained. Since the page offset is 16 bits (=64K page sizes) and the microTLB is fully associative, the tag is 16 bits long. The micro-TLB is searched for a tag match. If a tag match occurs, then the 16-bit physical page number is obtained from the TLB entry. If no tag in the microTLB matches, the level 2 TLB must be checked. The L2TLB has 512 entries, and 4-way set associative. The set index for the L2TLB is taken from the 16-bit virtual page number and the remaining bits are the L2TLB tag. The four entries of the indicated set are searched for a tag match. If a match is found, the physical page number is returned to the micro-TLB where it is appended to the page offset of the virtual address to form a physical address that will be used for the L2 cache lookup, if that is necessary.
Meanwhile, the bits of the page offset are decomposed for L1 cache access. Note here that using virtual address bits requires that the number of bits required for cache access has to fit within the page offset bits. That would be why the page size is so large for this implementation. The L1 cache has 128 sets, so 7 bits are used for the set index (64B blocks imply 6-bits for block offset). This means there are three bits left over from the page offset bits which are appended to the virtual page number to form the L1 cache tag. The entries of the indexed set are checked for a tag match. If there is a tag match, then theres an L1 hit. If not, theres an L1 miss and access goes on to L2.
For L2 access, we need the physical page number. If we were able to get that from a micro-TLB hit or a L2TLB hit, fine. If not, the page table needs to be consulted to find out where the page is. It may already be in memory, or it may not be. If the page table indicates the page is in memory, it will supply a physical page number and this is appended to the page offset to form the physical address. At the same time, the physical page number is sent to the L2TLB and the micro-TLB to take care of the misses which just occurred. The page table may indicate that the page is not in memory and must be paged in. This is a page fault. Once the page is in memory, the page table, L2TLB and micro-TLB are updated. The L2 cache is also updated with a data block from the page. The L1 cache is updated with information from L2 cache, and the L1 cache returns the requested data to the CPU.
Note that if the page table needs to be accessed because of a L2TLB miss, the data page containing the requested page table entry may itself not be in memory causing a double page fault. In this case, the page table page from the swap file on disk has to be read into main memory, the page table entry looked up, and possibly another data page fault needs to be processed.
For purposes of this simulation, however, we will not consider page faults or double page faults. All physical address data will reside at least in the L2TLB.
Program 1 will involve writing the TLB class which will be instantiated both for the micro-TLB and the L2TLB.
Program 2 will be writing the Cache class which will be instantiated for the L1 and L2 caches
Program 3 will wire up the TLBs and the Caches to make full running simulation. The simulation will begin with setting data into the TLBs and caches by reading an initialization data file. A virtual address data file will be read line by line and the virtual addresses in that file will be processed by the program. The resulting TLB and cache states will be written to a data file to verify that the simulation ran correctly.
the question is :
In this program, you will write the TLB class. You will be given some general descriptors of what must be in the class, along with a statement of functionality. A set of tests will be prescribed so that you can verify that youve constructed the class correctly.
An entry in the TLB (whether micro- or L2) consists of a valid boolean, a tag, an unsigned 32-bit data field, and an integer which contains LRU (Least Recently Used) data (essentially a clock or cycle value used to show how long ago that entry was last accessed).
The TLB constructor takes three parameters, the number of entries in the TLB, the associativity, and the number of bits in the data (physical page number).
The constructor would be declared as:
public TLB(int Entries, int Associativity, int TLBDataBits)
The class will have three other methods. One to get data from the TLB, one to set data into the TLB, and a third method to print the contents of the TLB. These methods are further specified as follows:
uint GetData(uint uAddress, uint uCycle)
This method will return the 16-bit physical page number from the TLB. In the case of a miss, the return value is 0xffffffff which would not be a 16-bit page number.
The TLB must take the requested address and decompose it into the set index and tag values. A tag match will be used to determine if there is a hit. In the case of a hit, the data value in the TLB entry is returned.
The cycle number is used to update the LRU field of the TLB entry if it exists. If an entry eviction is later required, the LRU values are used to determine which entry is evicted.
void SetData(uint uData, uint uAddress, uint uCycle)
This method enters data into the TLB. The address is decomposed to find out what set in which the tag will be searched for. If the tag is found, the data is written into the TLB entry data field and the LRU number is updated to the current cycle. If the tag is not found and an empty entry (valid == false) exists in the set, then the data is written to that entry. If no tag is found and no invalid entry exists in the set, the lowest LRU number if not equal to zero is overwritten with the new data, tag, and LRU cycle. An LRU entry of 0 with the valid bit set indicates that the TLB entry is locked, that is to say that it cannot be evicted.
void Print(String strFileName)
Print outputs a text file indicating the TLB contents.
The name of the output files is in the calling parameter.
If the TLB is fully associative, each entry is listed on a separate line. The data fields are entry number, valid bit, the tag, the LRU number and finally the data. All numbers are integer format except data which is output in hex. Fields are tab delimited.
If the TLB is not fully associative, then data from a single set appears on a line. The line does not appear if all entries in the set are invalid. If at least one entry is valid, then the line appears as follows. The set number is output as an integer. This is followed by n blocks od data where n is the associativity. So for a 4-way set associative TLB, the set number would be followed by information from the 4 blocks composing the set. The format of each block is the block number followed by valid status, the tag, the LRU number and the data. Again, only the data is shown in hex; the rest of the numbers are integers.
Test code for the fully associative TLB:
These tests are not complete, but they cover 90% of the TLB functionality. The GetData call is not tested in these cases. You should be able to write a GetData call and ensure that you are obtaining the correct data for the input address, and that the LRU number for the TLB entry is being updated.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
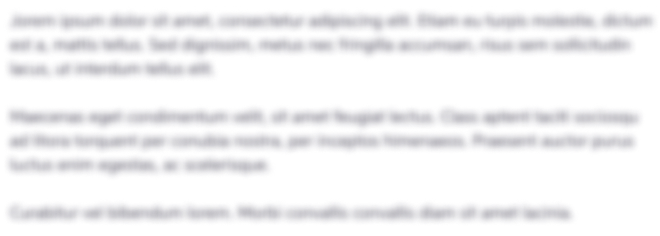
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started