Question
# This program exercises arrays and linked lists of nodes. # Replace any comments with your own code statement(s) # to accomplish the
# This program exercises arrays and linked lists of nodes.
# Replace any "
# The following files must be in the same folder: # arrays.py # node.py
from arrays import Array from node import Node
# Here is the array: theArray = Array(10) for i in range(len(theArray)): theArray[i] = i + 1
# Print the array: print("The array structure:") print(theArray)
head = Node(theArray[0], None) tail = head
# Part 1: # Copy the array items to a linked structure: # The linked structure must consist of Node class items. # You must use some form of a loop to create the linked structure. #
# Part 2: print("The linked structure:") # Print the linked structure with each item on a separate line. # You must use some form of a loop to print the linked structure. #
""" File: node.py Copyright 2015 by Ken Lambert
"""
class Node(object): """Represents a singly linked node."""
def __init__(self, data, next = None): self.data = data self.next = next
class TwoWayNode(Node): """Represents a doubly linked node."""
def __init__(self, data = None, previous = None, next = None): Node.__init__(self, data, next) self.previous = previous
""" File: arrays.py Copyright 2015 by Ken Lambert
An Array is a restricted list whose clients can use only [], len, iter, and str.
To instantiate, use
The fill value is None by default. """
class Array(object): """Represents an array."""
def __init__(self, capacity, fillValue = None): """Capacity is the static size of the array. fillValue is placed at each position.""" self._items = list() for count in range(capacity): self._items.append(fillValue)
def __len__(self): """-> The capacity of the array.""" return len(self._items)
def __str__(self): """-> The string representation of the array.""" return str(self._items)
def __iter__(self): """Supports iteration over a view of an array.""" return iter(self._items)
def __getitem__(self, index): """Subscript operator for access at index.""" return self._items[index]
def __setitem__(self, index, newItem): """Subscript operator for replacement at index.""" self._items[index] = newItem
Step by Step Solution
There are 3 Steps involved in it
Step: 1
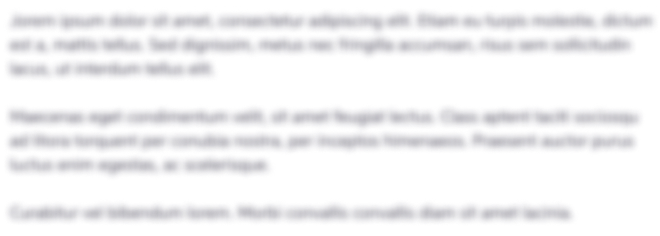
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started