Question
This program implements a recursive descent parser for grammar rules 5, 6, 7 below. Please implement rules 1, 2, 3, 4 for this parser. Relational
This program implements a recursive descent parser for grammar rules 5, 6, 7 below.
Please implement rules 1, 2, 3, 4 for this parser.
Relational operators in Rule 4 have spaces in front of and after each operator. The True and False literals in rule 3 also have spaces in front of and after each operator.
'|' means the | is a terminal symbol.
| alone is a meta-symbol separating different rewriting rules.
1
2
3
4
5
6
7
--------------------------------------------------------------------------------------------
import math
class ParseError(Exception): pass
i = 0
err = None
def texpression(): #implement rule 1
def tterm(): #implement rule 2
def tfact(): #implement rule 3
def relational(): #implement rule 4
def expression():
global i, err
val = term()
while True:
if s[i] == '+':
i += 1
val = operations('+', val, term())
elif s[i] == '-':
i += 1
val = operations('-', val, term())
else:
break
return val
def term():
global i, err
val = factor()
while True:
if s[i] == '*':
i += 1
val = operations('*', val, factor())
elif s[i] == '/':
i += 1
val = operations('/', val, factor())
else:
break
return val
def factor():
global i, err
val = None
if s[i] == '(':
i += 1
val = expression()
if s[i] == ')':
i += 1
return val
else:
print('missing )')
raise ParseError
elif s[i] == 'e':
i += 1
return math.e
else:
try:
val = float(s[i])
i += 1
except ValueError:
print('number expected')
val = None
#print('factor returning', val)
if val == None: raise ParseError
return val
def operations(op, left, right):
if op == '+': return left + right
elif op == '-': return left - right
elif op == '*': return left * right
elif op == '/': return left / right
else: return None
def float(x):
return float(x)
s = input(' Expression: ')
while s != '':
for c in '()+-*/':
s = s.replace(c, ' '+c+' ')
s = s.split()
s.append('$') # EOF marker
print(' Token Stream: ', end = '')
for t in s: print(t, end = ' ')
print(' ')
i = 0
try:
print('val: ', bexpression())
except:
print('parse error')
print()
if s[i] != '$': print('Syntax error:')
print('read | did not read: ', end = '')
for c in s[:i]: print(c, end = ' ')
print(' | ', end = '')
for c in s[i:]: print(c, end = ' ')
print()
s = input(' Expression: ')
'''
Example:
Expression: 2*(3 + 4) < 15 & 1 + 2 == 3
Token Stream: 2 * ( 3 + 4 ) < 15 & 1 + 2 == 3 $
val: True
read : did not read: 2 * ( 3 + 4 ) < 15 & 1 + 2 == 3 : $
Expression: ~ 2*(3 + 4) < 15 & 1 + 2 == 3
Token Stream: ~ 2 * ( 3 + 4 ) < 15 & 1 + 2 == 3 $
val: False
read : did not read: ~ 2 * ( 3 + 4 ) < 15 & 1 + 2 == 3 : $
'''
Step by Step Solution
There are 3 Steps involved in it
Step: 1
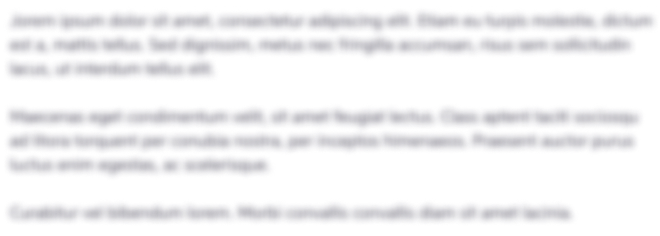
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started