Question
*This program needs to simulate a vending machine. In java using this code a vending machine program that uses an Interface. public class Inventory {
*This program needs to simulate a vending machine.
In java using this code a vending machine program that uses an Interface.
public class Inventory {
private Map inventory = new HashMap();
public int getQuantity(T product) {
Integer value = inventory.get(product);
return value == null ? 0 : value;
}
public void add(T product) {
int count = inventory.get(product);
inventory.put(product, count + 1);
}
public void deduct(T product) {
if (hasItem(product)) {
int count = inventory.get(product);
inventory.put(product, count - 1);
}
}
public boolean hasItem(T product) {
return getQuantity(product) > 0;
}
public void clear() {
inventory.clear();
}
public void put(T product, int quantity) {
inventory.put(product, quantity);
}
}
public enum Product {
COKE("Coke", 25), PEPSI("Pepsi", 35), SODA("Soda", 45);
private String name;
private int price;
private Product (String name, int price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public long getPrice() {
return price;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
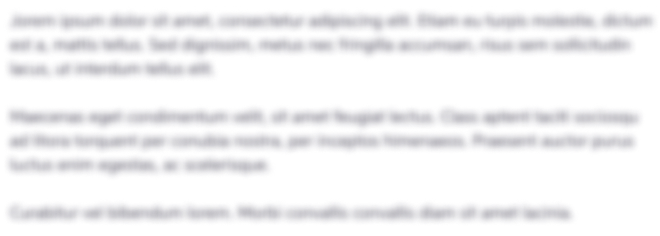
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started