Question
This program will utilize arrays to store data about 4 students and their scores. Each student will have 3 scores. Therefore, a normal single dimensional
This program will utilize arrays to store data about 4 students and their scores. Each student will have 3 scores. Therefore, a normal single dimensional array will not be sufficient. The nature of this data requires a special data structure a rectangular array. A rectangular array can be visualized like a regular table, with rows (dimension 0) and columns (dimension 1). Each row will represent a student. Within each row, the students scores will be distributed in columns. We will utilize for loops to go through or traverse the arrays. Since there are rows and columns one loop, the outer one will be used to traverse through rows (students). The inner loop will be used to traverse through columns (scores for each student)
Instructions Rename your Programc.s file to RectangularArrays.cs. Within the Main method of your application, write C# statements for following parts. Make sure you are putting in your name on line 1!
1. Declare Variables 1.1. Declare a rectangular array of integers called scores. Remember to use the square brackets with the data type and insert a comma to denote that the array is rectangular. The square brackets will follow the data type. Do not initialize the array on this line. 1.2. Declare variables for storing (a) score total and (b) average. Decide which data types will be appropriate and initialize the variables.
2. Initialize the arrays 2.1. Initialize the scores array by allocating it 4 rows and 3 columns. This is not a declaration therefore, do not specify the data type on the left hand side. Data types are only specified when declaring a new variable. Use the new operator on the right-hand side and specify the data type of the array (it must be the same data type as when you declared it in #1) and put in the two-dimensional array size within square brackets. Use the following structure: e.g. arrayName = new datatype[rows, columns]; Doing the above will create a new array of with the specified row and columns and link it to the variable on the left hand side.
3. Using WriteLine statements, create the header of the program. Look at the sample application screenshot for details on what to write. Loop through the scores array using nested for loops and ask for user input as shown below. This nested for loop will become the standard for loop for going through or traversing rectangular arrays:
4. Create a for loop for traversing through rows. It should have 1) a counter variable called i that starts at 0 as its initial value. 2) the condition should test that the variable i is less than the number of elements in Dimension 0. Get the number of elements in Dimension 0 using the following structure: arrayName.GetLength(dimension_number) Remember that dimension number for rows is 0 and for columns is 1.
4.1. Using a Write statement, prompt the user to enter scores for Student. Specify the student number by using the counter variable. You will need to offset the counter variable so that users see the count start from 1.
4.2. Create a nested for loop for traversing through columns. This loop should look identical to the outer loop you created to go through the rows. However, this time, you need to traverse through Dimension 1. Therefore, make sure you are using that dimension number when you utilize GetLength.
4.2.1. Using a Write statement, prompt the user to enter a score for Student. Specify the score number by using the counter variable from the inner loop. The final prompt should look like this: Score 1:. You will need to offset the counter variable so that users see the count start from 1.
4.2.2. Using ReadLine, read input from the Console (remember to do a Convert since we are asking the user to enter numeric input). You dont need a separate variable for input here. Store the input in the array element remember that you must use square brackets since index number since you are storing a value into an array element (not the array itself). Within the square brackets, you will need to specify the row number and the column number. e.g. // assign a value to element at row i and column j in the array // arrayName[i,j] = value; Create a second nested for loop structure for calculating average as shown below. This is not connected to the previous for!
5. Create an outer for loop. Use the nested structure you just implemented. The outer for will loop through the rows dimension 0.
5.1. Create an inner for loop. The inner for will loop through all the columns dimension 1.
5.1.1. Use the accumulated totals structure to maintain a total of all the scores in the rectangular array. In order to get values out of the array, you need to type in the name of the array, the square brackets, and within the brackets specify the row and the column number. e.g. Write ( arrayName[row, column]); The example above reads the value stored at the specified row and column number. The central difference in reading from and writing to an array location is that to write to an array location involves an assignment symbol and a value to the right of the assignment symbol. If there is no assignment symbol, the compiler will read from that location (as shown above).
6. Tell the total number of scores present. Use a WriteLine statement to tell the user how many scores were entered. There is no need to keep a track of scores manually. Using the Length property of the array gives you the total number of elements in the array. The Length property is giving you the total number of elements in the entire array (including all rows and all columns), whereas GetLength only returns the number of arrays in one dimension.
7. Calculate the average score by dividing the total of the scores (you calculated this earlier!) by the total number of elements.
8. Print the average to the Console using a WriteLine statement
The Final Project should like that USE C# TO DO THIS PROJECT!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
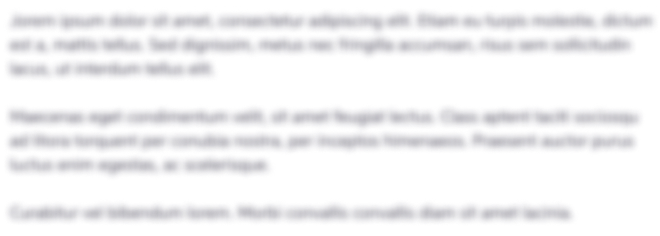
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started