Question
This project deals with some basic descriptive statistics; specifically, mean, median, mode, skewness, and kurtosis. Our recent lecture entitled Working with Data addressed the meanings
This project deals with some basic descriptive statistics; specifically, mean, median, mode, skewness, and kurtosis. Our recent lecture entitled Working with Data addressed the meanings and computations of each of these. Use those lecture slides as a reference while designing and implementing your program. Your program will allow a user to read in the data from a file of their choosing and to choose if they would like to compute the mean, median, mode, skewness, or kurtosis for the data set. The user may also choose to compute all five statistics at once Coding Standards Prior to this assignment, you should re-read the Coding Standards, available on the CMSC 201 course website at the top of the Assignments page. For now, you should pay special attention to the sections about: Comments o File header comments o Function header comments o Note that Input and Output in the function header comment do NOT mean what is shown on the screen with print() or what is gotten from the user with input(). They refer to the parameters taken in, and the return value. (Both Input and/or Output may be None, if appropriate.) Constants o You must use constants instead of magic numbers or strings!!! Make sure to read the last page of the Coding Standards document, which prohibits the use of certain tools and Python keywords. (Note that you can use the built-in sqrt() function and sort() method only for this project.) For this assignment, you may assume that: - The user will enter a valid filename - The user will enter an integer for the menu option, but it may be outside of the allowable range. If the user enters an integer that is out of range, your program should display an error message and re-prompt the user. Design Document The design document will make sure that you begin thinking about your project in a serious way early. This will give you experience doing design work and help you gauge the number of hours you'll need to set aside to complete the project. Your design document must be called design1.txt. For Project 1, the design overview is presented in the Design Information section below, and you are required to follow it. For future projects, you will be creating the design entirely on your own and may choose to design it however you like. Your design document must have four separate parts: 1. A file header, similar to those for your homework assignments 2. Constants a. A list of all the constants your program will need, including a short comment describing what each group of constants is for 3. Function headers a. A complete function header comment for each function 4. Pseudocode for main() a. A brief but descriptive breakdown of the steps your main() function will take to completely solve the problem; note function calls under relevant comments (if applicable) Design Information You are required to implement and use at least the following 11 functions for Project 1, in addition to main(). You may implement more functions if you think them necessary, but the 11 below must be implemented and used. The information for each function is given below. Printing Functions As the name suggests, these functions display information for the user. They do not return any values they only print information, which does NOT count as output. Some of them take in parameters, while others do not. You must implement and use all of the following printing functions. def displayMenu() o Prints out the programs main menu: Compute: 1) Mean 2) Median 3) Mode 4) Standard deviation 5) Skew 6) Kurtosis 7) All statistics o Takes in nothing (the menu is always the same) o Returns nothing, since it is a print function def displaySkew(skewValue) o Determines if a skew value is negative, positive, or zero. Then, displays a message saying which it is. skewValue is a numeric (integer or floating point) skewness value o Returns nothing, since it is a print function def displayKurt(kurtValue) o Determines if a kurtosis value is platykurtic (< 3), leptokurtic (> 3), or mesokurtic (= 3). Then, displays a message saying which it is. kurtValue is a numeric (integer or floating point) kurtosis value o Returns nothing, since it is a print function Helper Functions As the name suggests, these functions help other functions by performing small tasks that will be needed by many pieces of the program. They are often called from functions other than main(). You must implement and use the following helper function. def getValidMenuChoice(prompt, minimum, maximum) o Gets a valid integer from the user that falls within a certain range (between minimum and maximum, inclusive), using the provided string of prompt when asking the user for input. o Takes in a prompt and two integers The prompt is used by the function when asking for input The two integers are the min and max values (inclusive) o Returns a string (the menu choice or the quit value) o Preconditions: minimum <= maximum HINT: Look at Lecture 13, Program Design, for an example of a similar function, getValidInt(), which does not contain a prompt parameter. Note that your getValidMenuChoice function returns a string, not an integer. This is because your function must accommodate the quit value of q. General Functions These are the heavy lifters of the program, and do the majority of the work, and are almost always called from main(). They often call other functions, often more than once. You must implement and use all of the general functions given below. def makeList(filename) o Takes in a filename as a string o Reads in the contents of a .csv (comma-separated value) file of integer values Values will be integer values >= 0 The file will contain at least two integers o Returns a list of integer values This function is given to you!!! o You can find it in Dr. Mitchells public directory (proj1.py). o Make sure to include the provided function header. o Do not change the code it works perfectly as is! def mean(values) o Computes the mean of a set of numeric values values is a list of numeric (integer, floating point, or mixed) values o Returns the mean of the values o Preconditions: values contains >= 1 value o Note that this function does not display the mean. It simply computes and returns it. def median(values) o Finds the median of a set of numeric values values is list of numeric (integer, floating point, or mixed) values o Returns the median of the values o Preconditions: values contains >= 2 values o Note that this function does not display the median. It simply finds and returns it. o You will need to sort the list of values from smallest to largest in order to find the median. Use the Python built-in sort()method, as you did in Homework 5, Part 2. Dont forget to make a local copy of values before sorting! def mode(values) o Finds the mode of a set of integer values, all >= 0 values is a list of integer values o If the distribution of values is unimodal (i.e. has only one mode) returns the integer mode of the values o If the distribution is not unimodal (i.e. has more than one mode) displays a message and returns a mode of -1 o Preconditions values contains integer values, all >= 0 values contains >= 1 value o Note that this function does not display the mode. It simply finds and returns it. o You will need to sort the list of values from smallest to largest in order to find the mode. Use the Python built-in sort()method, as you did in Homework 5, Part 2. Dont forget to make a local copy of values before sorting! def stdev(values) o Computes the standard deviation of a set of numeric values values is a list of numeric (integer, floating point, or mixed) values o Returns the floating point standard deviation of the values o Preconditions: values contains >= 2 values o Note that this function does not display the standard deviation. It simply computes and returns it. o The standard deviation computation requires the use of a square root. Use the Python built-in sqrt()method. Use it as follows: math.sqrt(value) where value = a numeric value HINT: Take advantage of the mean() function that you have written def skew(values) o Computes the skew of a set of numeric values values is a list of numeric (integer, floating point, or mixed) values o Returns the floating point skew of the values o Preconditions: values contains >= 2 values o Note that this function does not display the skew value. It simply computes and returns it. HINT: Take advantage of the mean() and stdev() functions that you have written! def kurt(values) o Computes the kurtosis of a set of numeric values values is a list of numeric (integer, floating point, or mixed) values o Returns the floating point kurtosis of the values o Preconditions: values contains >= 2 values o Note that this function does not display the kurtosis value. It simply computes and returns it. HINT: Take advantage of the mean() and stdev() functions that you have written!
1.Coding Standard document.:https://www.csee.umbc.edu/courses/undergraduate/201/fall18/docs/CMSC%20201%20-%20Python%20Coding%20Standards.pdf
2.Sample format for design document: https://s3.us-east 1.amazonaws.com/blackboard.learn.xythos.prod/5954eb74c7df4/3730175?response-content-disposition=inline%3B%20filename%2A%3DUTF-8%27%27design1%25281%2529.txt&response-content-type=text%2Fplain&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Date=20181021T031818Z&X-Amz-SignedHeaders=host&X-Amz-Expires=21600&X-Amz-Credential=AKIAIL7WQYDOOHAZJGWQ%2F20181021%2Fus-east-1%2Fs3%2Faws4_request&X-Amz-Signature=aa6ec0adc90ac06c95a5a502f391610b488fbeb2adf31218c95577c8808919ae
3.Lecture slides: https://s3.us-east-1.amazonaws.com/blackboard.learn.xythos.prod/5954eb74c7df4/3730174?response-content-disposition=inline%3B%20filename%2A%3DUTF-8%27%27CMSC%2520201%2520-%2520Project%25201%2520-%2520Descriptive%2520Statistics_F18.pdf&response-content-type=application%2Fpdf&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Date=20181021T031538Z&X-Amz-SignedHeaders=host&X-Amz-Expires=21600&X-Amz-Credential=AKIAIL7WQYDOOHAZJGWQ%2F20181021%2Fus-east-1%2Fs3%2Faws4_request&X-Amz-Signature=f4271f54f8c49063bbcd2debc6af2baebcd0d38ecf6d900cfb918e4b97619540
4. Format of file: As shown in the example and slide.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
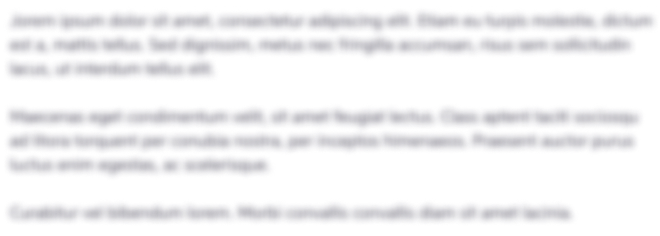
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started