Question
This project is designed to help you better understand the use of: Decision structures Loops Input/Output Files Print functions Methods Create a class named RandomGame
This project is designed to help you better understand the use of:
Decision structures
Loops
Input/Output
Files
Print functions
Methods
Create a class named RandomGame with a main method and any additional methods that you think will help your program be efficient and readable. For this project, you must create a very simple simulation of a game between a human player and the computer. The RandomGame works as follows: A human player competes against the computer by randomly receiving points between 0 and 100. Every turn, the human player can choose to either continue playing the game or end the game. If the human player chooses to end the game, the computer gets a 15% bonus to its final score. Whichever player has the highest final score wins.
The criteria for your code are as follows:
You will first display the rules for the player in your own words. Keep in mind that all your messages to the player whether they be input or output related should be done using the JOptionPane class. To help you out with properly formatting your output and requesting yes/no input from players, think about the tools that we discussed in class.
You must ask the player for his/her name and keep it throughout your program, then ask the player if he/she is ready to start the game.
If the player replies No, then you must continue asking until he/she is ready to play the game.
You will need to open a file and keep track of all the points awarded throughout the game. Format the scoreboard like a table. You must include a heading, a line separating the heading from the scores, and the scores. Dont forget to use the escape characters that weve learned about in class to make your table look nice.
In the game, the human player takes at most 10 turns. For each turn the human player takes, there will be a score awarded to the human and a score awarded to the computer.
For each turn, you will let the player know what turn it is (i.e. Turn 1, 2, 3, etc.), then you will display the current total scores for each player, and finally you will ask the player if he/she wants to end the game now or keep playing
If the human player wants to continue, you will use the Java Random class to find a random number between 0 and 100 and add that number to the players score. The computer will also receive points in the same way
Remember: you must write all scores to a file in a neat table format.
Every turn, after determining how many points each player gets, you must display the round score for each player.
Once the game is over you must display a message declaring a winner and the contents of the file you have been writing to
Keep in mind that if the computer earns the 15% bonus you need to account for that information (including 0 points for the human player) in your file so it can also be displayed at the end of the game.
The last message needs to ask the player if he/she want to play again.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
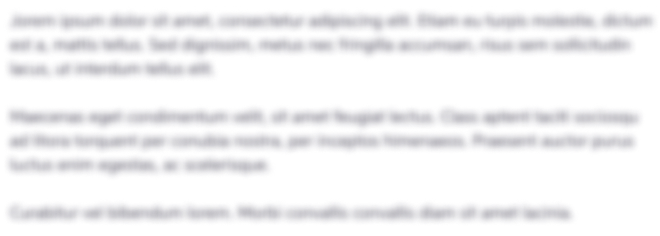
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started