Question
This project will study the coordination of multiple threads using semaphores. The design should consist of two things: (1) a list of every semaphore, its
This project will study the coordination of multiple threads using semaphores.
The design should consist of two things: (1) a list of every semaphore, its purpose, and its initial value, and (2) pseudocode for each function. The pseudocode should be similar to the pseudocode shown in the textbook for the barbershop problem. Every wait and signal call must be included in the pseudocode.
Your code should be nicely formatted with plenty of comments. The code should be easy to read, properly indented, employ good naming standards, good structure, and should correctly implement the design. Your code should match your pseudocode.
This project must target a Unix platform and execute properly on Linux server.
The project must be written in C, C++, or Java.
If using C or C++, you must use POSIX pthreads and semaphores (no mutexes, locks, etc.)
If using Java, you must use Java Threads and Java Semaphores (java.util.concurrent.Semaphore).
You may not use the synchronized keyword in Java for mutual exclusion.
You may not use Java data structures that have built-in mutual exclusion.
A bank is simulated by using threads and semaphores to model customer and employee behavior.
This project is similar to the barbershop example in the textbook. The following rules apply:
Customer:
1)5 created initially, one thread each.
2)Each customer will make 3 visits to the bank.
3)Each customer starts with a balance of $1000.
4)On each visit a customer is randomly assigned one of the following tasks:
a)make a deposit of a random amount from $100 to $500 in increments of $100
b)make a withdrawal of a random amount from $100 to $500 in increments of $100
withdrawals may exceed the balance
c)request a loan of a random amount from $100 to $500 in increments of $100
5)Steps for each task are defined in the task table.
Bank Teller:
1)Two created initially, one thread each.
2)Serves next customer in the teller line.
3)Processes customer request and updates customer balance.
Loan Officer:
1)One created initially as one thread.
2)Serves next customer in line for a loan.
3)Approves loan request and updates customer balance by adding the loan amount.
Main
1)Creates and joins all customer threads.
2)When last customer has exited, prints the summary report and ends the simulation.
Other rules:
1)Each activity of each thread should be printed with identification (e.g., customer 1).
2)All mutual exclusion and coordination must be achieved with semaphores.
3)A thread may not use sleeping as a means of coordination.
4)Busy waiting (polling) is not allowed.
5)Mutual exclusion should be kept to a minimum to allow the most concurrency.
6)The semaphore value may not be obtained and used as a basis for program logic.
7)Each customer thread should print when it is created and when it is joined.
8)All activities of a thread should only be output by that thread.
9)Threads use sleep to simulate task time but scaled so that 1 minute only delays 1/10 second.
Output:
Your output must be a logical ordering of events. Your output should match the wording of the sample output. The order of statements, worker assigned, amounts involved and transactions processed may vary.
It is okay to have all threads print created before processing begins, but in my output processing begins as soon as a thread is created.
1)Thread activity
Loan Officer created 0
Customer 0 created
Teller 0 created
Teller 0 begins serving customer 0
Customer 0 requests of teller 0 to make a withdrawal of $300
Teller 1 created
Customer 1 created
Loan Officer serving customer 1
Customer 1 requests of loan officer to apply for a loan of $100
Customer 2 created
Teller 1 begins serving customer 2
Customer 2 requests of teller 1 to make a deposit of $500
Teller 0 processes withdrawal of $300 for customer 0
Loan Officer approves loan for customer 1
Teller 1 processes deposit of $500 for customer 2
Customer 3 created
Customer 4 created
Customer 0 gets cash and receipt from teller 0
Customer 1 gets loan from loan officer
Customer 2 gets receipt from teller 1
Teller 0 begins serving customer 4
Customer 4 requests of teller 0 to make a withdrawal of $400
Loan Officer serving customer 3
Customer 3 requests of loan officer to apply for a loan of $300
Teller 1 begins serving customer 0
Customer 0 requests of teller 1 to make a withdrawal of $100
Teller 0 processes withdrawal of $400 for customer 4
Loan Officer approves loan for customer 3
Teller 1 processes withdrawal of $100 for customer 0
Customer 3 gets loan from loan officer
Customer 4 gets cash and receipt from teller 0
Customer 0 gets cash and receipt from teller 1
Loan Officer serving customer 3
Customer 3 requests of loan officer to apply for a loan of $300
Teller 0 begins serving customer 1
Customer 1 requests of teller 0 to make a deposit of $300
Teller 1 begins serving customer 2
Customer 2 requests of teller 1 to make a withdrawal of $200
Loan Officer approves loan for customer 3
Teller 0 processes deposit of $300 for customer 1
Teller 1 processes withdrawal of $200 for customer 2
Customer 3 gets loan from loan officer
Customer 1 gets receipt from teller 0
Customer 2 gets cash and receipt from teller 1
Loan Officer serving customer 0
Customer 0 requests of loan officer to apply for a loan of $300
Teller 0 begins serving customer 4
Teller 1 begins serving customer 3
Customer 4 requests of teller 0 to make a deposit of $300
Customer 3 requests of teller 1 to make a withdrawal of $300
Loan Officer approves loan for customer 0
Teller 1 processes withdrawal of $300 for customer 3
Teller 0 processes deposit of $300 for customer 4
Customer 0 gets loan from loan officer
Customer 3 gets cash and receipt from teller 1
Customer 4 gets receipt from teller 0
Customer 0 departs the bank
Customer 3 departs the bank
Customer 0 is joined by main
Teller 1 begins serving customer 1
Customer 1 requests of teller 1 to make a withdrawal of $500
Teller 0 begins serving customer 2
Customer 2 requests of teller 0 to make a deposit of $300
Teller 1 processes withdrawal of $500 for customer 1
Teller 0 processes deposit of $300 for customer 2
Customer 2 gets receipt from teller 0
Customer 1 gets cash and receipt from teller 1
Customer 2 departs the bank
Customer 1 departs the bank
Teller 0 begins serving customer 4
Customer 4 requests of teller 0 to make a deposit of $500
Customer 1 is joined by main
Customer 2 is joined by main
Customer 3 is joined by main
Teller 0 processes deposit of $500 for customer 4
Customer 4 gets receipt from teller 0
Customer 4 departs the bank
Customer 4 is joined by main
At the end of the simulation, display a report as follows:
Bank Simulation Summary
Ending balance Loan Amount
Customer 0 900 300
Customer 1 900 100
Customer 2 1600 0
Customer 3 1300 600
Customer 4 1400 0
Totals 6100 1000
Step by Step Solution
There are 3 Steps involved in it
Step: 1
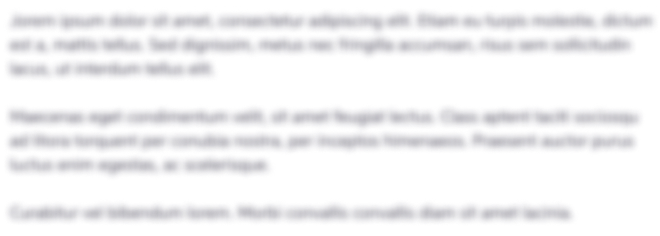
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started