Question
This Python code copies a file, line by line. It presumes thatthe input and output files will be in the same directory (folder)as the code
This Python code copies a file, line by line. It presumes thatthe input and output files will be in the same directory (folder)as the code itself.
infile_name = input("Please enter the name of the file to copy:")
infile = open(infile_name, 'r')
outfile_name = input("Please enter the name of the new copy:")
outfile = open(outfile_name, 'w')
for line in infile:
outfile.write(line)
infile.close()
outfile.close()
(1) Copy this code into your lab7.py file on your own system(or, temporarily, into a separate file if that makes it easier foryou to experiment). Package it into a function called copy_filethat takes no parameters and returns no value (because it does allits work by prompting the user and reading and writing files). Testit out by copying a short text file.
Then download the Project Gutenberg version of The Adventures ofSherlock Holmes fromhttp://www.gutenberg.org/cache/epub/1661/pg1661.txt (ProjectGutenberg is a wonderful resource for non-copyright-protectedtexts). Call your file-copying function to make a copy of thisfile. [Some problems have been reported with reading ProjectGutenberg files. If you run into messages saying that Python can'tdecode a character, open the file with open(infile_name, 'r',errors='ignore').]
(2) Modify your copy_file function to take one parameter, astring. If the parameter is 'line numbers', the copied fileincludes line numbers at the start of each line
1: Project Gutenberg's The Adventures of Sherlock Holmes,by Arthur Conan Doyle
2:
3: This eBook is for the use of anyone anywhere at no cost andwith
...
13052: subscribe to our email newsletter to hear about neweBooks.
If the parameter is anything else, the function just copies thefile as before. Note that the line number is formatted andright-justified in a five-character field.
(3) If you examine the file from Project Gutenberg, you see thatit contains some "housekeeping" information at the beginning and atthe end. You'll also see that the text itself starts after a linebeginning with "*** START" and ends just before a line beginningwith *"*** END". Modify your copy_file function so that if itsparameter is 'Gutenberg trim' it will copy only the body of aProject Gutenberg file, omitting the "housekeeping" material at thefront and end. (You may assume—you don't have to check—that if thisparameter is specified, there will be a "*** START" line and an"*** END" line in the file.)
(4) Modify your copy_file function so that if its parameter is'statistics' it will copy the file as before but also print outthese statistics (which should be familiar) about the text in thefile, following the formatting shown:
16824 lines in the file
483 empty lines
53.7 average characters per line
65.9 average characters per non-empty line
Step by Step Solution
3.45 Rating (152 Votes )
There are 3 Steps involved in it
Step: 1
1 To package the code into a function called copyfile that takes no parameters and returns no value you can do the following def copyfile infilename i...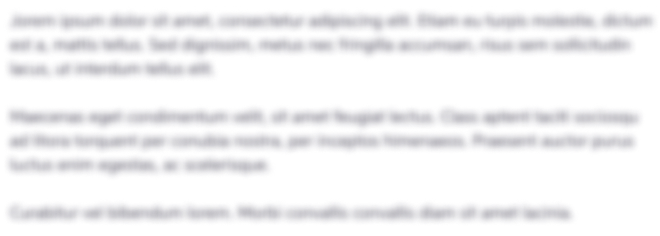
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started