Question
This question is about dijkstra's algorithm. The code (python3) is just open the input file and read it: #!/usr/bin/env python3 def main(): d = {}
This question is about dijkstra's algorithm.
The code (python3) is just open the input file and read it:
#!/usr/bin/env python3
def main():
d = {} #empty dictionary
with open("a.txt", "r") as file:
for line in file:
#split line by space and remove' '
l = [x.strip(" ") for x in line.split(" ")]
#make 1st element as key
#for each element other than 1st element split it by ',' & make a int tuple
d[int(l[0])] = [(int(x.split(",")[0]), int(x.split(",")[1])) for x in l[1:]]
print(d)
main()
Output:
{ 1: [(2, 1), (8, 2)], 2: [(1, 1), (3, 1)], 3: [(2, 1), (4, 1)], 4: [(3, 1), (5, 1)], 5: [(4, 1), (6, 1)], 6: [(5, 1), (7, 1)], 7: [(6, 1), (8, 1)], 8: [(7, 1), (1, 2)] }
I would like to know how to do followint steps.
Step 2. Next you should initialize the two variables needed for Dijkstras algorithm: an array that holds all of the path lengths (if you prefer, you can initialize path lengths to 1000000 instead of infinity), and an initially empty set. For this assignment, your start vertex, s will always be vertex 1, so you should set the distance to vertex 1 to zero and add 1 to the set.
Step 3. In the next step you need to implement the loop in Dijkstras Algorithm. Remember, you will need to loop until every vertex has been visited (another way to say this is that you should loop until |your set| is equal to n). Here are the highlights of what this loop does:
An edge (v,w) should only be considered if v is in your set and w is not.
Thus, for each vertex already in your set you will need to check each of the vertices to which it is connected.
Calculate Dijkstras greedy criterion for each of the considered edges and save the edge with the smallest value (the shortest path length).
Add the appropriate vertex to your set and update the vertexs path length.
Step 4. After the loop, you should print all path lengths as a comma separated list.
0,1,2,3,4,4,3,2
Step by Step Solution
There are 3 Steps involved in it
Step: 1
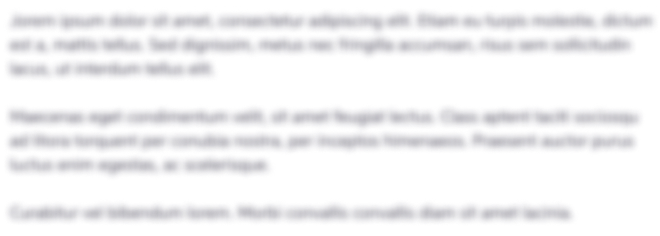
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started